


Learn the template functions in Go language and implement dynamic rendering of web page templates
Learn the template functions in the Go language and implement dynamic rendering of web page templates
Introduction:
In Web development, the use of dynamic web page templates is very common. In the Go language, we can achieve dynamic rendering of web page templates by using template functions. This article will introduce how to learn and use template functions in the Go language, and demonstrate through examples how to implement dynamic rendering of web page templates.
- Introduction to template functions in Go language
The template function is a powerful template extension mechanism provided by the Go language template package. We can customize functions and call them in templates to achieve various dynamic rendering effects.
In Go language templates, we can register custom template functions through the Funcs
method. The sample code is as follows:
package main import ( "html/template" "os" ) func main() { tpl := template.New("example") // 创建一个模板对象 tpl.Funcs(template.FuncMap{ "add": func(a, b int) int { // 注册名为add的自定义函数 return a + b }, }) tpl.Parse(`{{ add 1 2 }}`) // 在模板中使用自定义函数 tpl.Execute(os.Stdout, nil) }
In the above sample code, we registered a custom function named add
through the tpl.Funcs
method and added it in the template This function is used. The final output we get is 3
.
Through template functions, we can customize various powerful processing methods, such as string conversion, timestamp conversion, data formatting, etc.
- Implementing dynamic rendering of web page templates
Next, we will use template functions to implement dynamic rendering of web page templates. We assume that there is a user information structure defined as follows:
type User struct { Name string Age int }
Now we create a web page template and use the template function to dynamically render user information. The sample code is as follows:
package main import ( "html/template" "os" ) type User struct { Name string Age int } func main() { tpl := template.New("example") // 创建一个模板对象 tpl.Funcs(template.FuncMap{ "toUpperCase": func(s string) string { // 实现字符串转换为大写的自定义函数 return strings.ToUpper(s) }, }) tpl.Parse(` <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>User Info</title> </head> <body> <h1>Hello, {{ toUpperCase .Name }}</h1> <p>Your age is: {{ .Age }}</p> </body> </html> `) user := User{ Name: "Alice", Age: 25, } tpl.Execute(os.Stdout, user) }
In the above sample code, we convert the user name to uppercase through the toUpperCase
custom function and dynamically render the user information in the web page template. The final output we get is as follows:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>User Info</title> </head> <body> <h1>Hello, ALICE</h1> <p>Your age is: 25</p> </body> </html>
Through this example, we can see that using template functions can help us achieve a more flexible dynamic rendering effect of web page templates.
Conclusion:
The template function in the Go language provides us with a simple and efficient way to achieve dynamic rendering of web page templates. Through custom functions, we can handle various complex operations to better meet project needs. In actual development, we can combine template functions and data models to achieve richer and more personalized dynamic rendering effects of web pages. I hope this article will be helpful to you when learning and using template functions in the Go language!
The above is the detailed content of Learn the template functions in Go language and implement dynamic rendering of web page templates. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


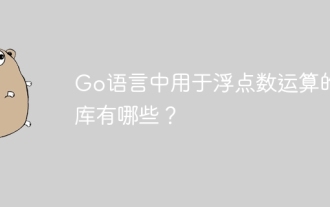
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
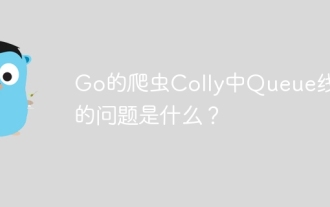
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
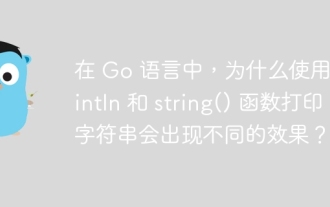
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
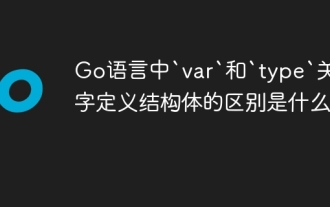
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
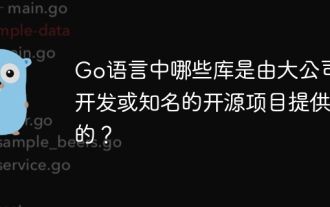
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
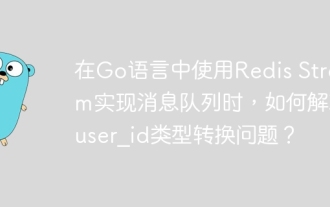
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
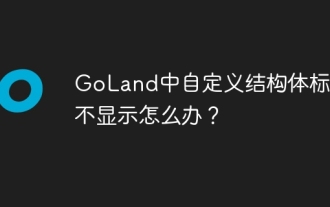
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
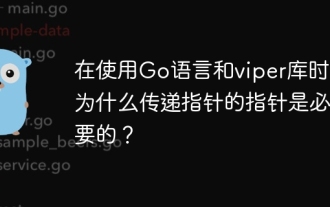
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
