


Quick Start: Use Go language functions to implement simple image recognition functions
Quick Start: Use Go language functions to implement simple image recognition functions
In today's technological development, image recognition technology has become a hot topic. As a fast and efficient programming language, Go language has the ability to implement image recognition functions. This article will provide readers with a quick start guide by using Go language functions to implement simple image recognition functions.
First, we need to install the Go language development environment. You can download the installation package suitable for your operating system from the official Go language website (https://golang.org/), and then install it according to the prompts.
Next, we need to use some libraries in the Go language to implement the image recognition function. In the Go language, there is a standard library called "image", which provides functions for processing and manipulating images. In particular, the "image.Decode" function in the "image" library can decode image files into image objects (Image) in the Go language.
The following is a sample code that uses Go language functions to implement image recognition functions:
package main import ( "fmt" "image" "os" ) func main() { // 打开图像文件 file, err := os.Open("image.jpg") if err != nil { fmt.Println("打开图像文件失败:", err) return } defer file.Close() // 解码图像文件 img, _, err := image.Decode(file) if err != nil { fmt.Println("解码图像文件失败:", err) return } // 获取图像尺寸 bounds := img.Bounds() width := bounds.Dx() height := bounds.Dy() // 输出图像尺寸信息 fmt.Println("图像尺寸:", width, "x", height) // 进行图像识别操作 // ... // 输出识别结果 // ... }
In the above code, we first decode the image file into an image object through the "image.Decode" function . Then, use the "Bounds" method of the image object to obtain the size information of the image, and obtain the width and height of the image through the "Dx" and "Dy" methods. Next, we can perform logical operations on image recognition. Finally, according to your own needs, you can output the recognition results to the console or save them as a file.
It should be noted that in actual applications, simply using the above code may not achieve accurate and efficient image recognition functions. In order to improve the recognition accuracy and speed, we can use some open source third-party libraries, such as "tensorflow", "opencv", etc. These libraries provide different image recognition algorithms and models, which can achieve more complex and accurate image recognition functions. You can introduce these libraries into your Go language project and configure and call them according to their usage documentation.
In summary, this article uses Go language functions to implement simple image recognition functions to help readers quickly get started with image recognition technology. At the same time, it also introduces readers to how to combine third-party libraries to further improve the accuracy and speed of image recognition. I hope this article will be helpful to readers in learning and applying image recognition technology.
The above is the detailed content of Quick Start: Use Go language functions to implement simple image recognition functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
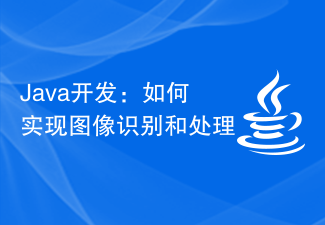
Java Development: A Practical Guide to Image Recognition and Processing Abstract: With the rapid development of computer vision and artificial intelligence, image recognition and processing play an important role in various fields. This article will introduce how to use Java language to implement image recognition and processing, and provide specific code examples. 1. Basic principles of image recognition Image recognition refers to the use of computer technology to analyze and understand images to identify objects, features or content in the image. Before performing image recognition, we need to understand some basic image processing techniques, as shown in the figure
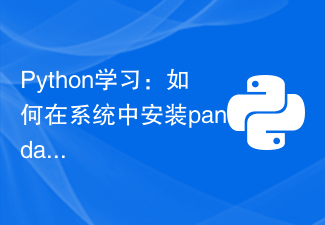
Quick Start: How to install the pandas library in Python requires specific code examples 1. Overview Python is a widely used programming language with a powerful development ecosystem that includes many practical libraries. Pandas is one of the most popular data analysis libraries. It provides efficient data structures and data analysis tools, making data processing and analysis easier. This article will introduce how to install the pandas library in Python and provide corresponding code examples. 2. Install Py
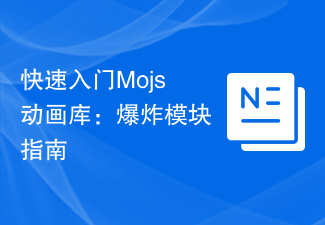
We start this series by learning how to animate HTML elements using mojs. In this second tutorial, we continue using the Shape module to animate built-in SVG shapes. The third tutorial covers more ways to animate SVG shapes using ShapeSwirl and the stagger module. Now we will learn how to animate different SVG shapes in bursts using the Burst module. This tutorial will depend on the concepts we introduced in the previous three tutorials. If you haven't read them yet, I recommend reading them first. Creating a Basic Burst Animation The first thing we need to do before creating any burst animation is to instantiate a Burst object. Afterwards, we can specify different properties
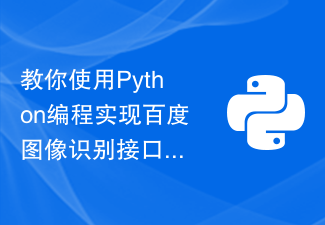
Teach you to use Python programming to implement the docking of Baidu's image recognition interface and realize the image recognition function. In the field of computer vision, image recognition technology is a very important technology. Baidu provides a powerful image recognition interface through which we can easily implement image classification, labeling, face recognition and other functions. This article will teach you how to use the Python programming language to realize the image recognition function by connecting to the Baidu image recognition interface. First, we need to create an application on Baidu Developer Platform and obtain
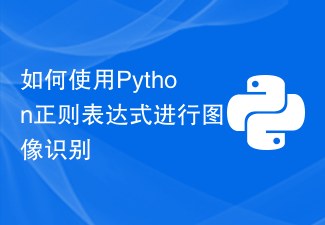
In computer science, image recognition has always been an important field. Using image recognition, we can let the computer recognize and analyze the content in the image and process it. Python is a very popular programming language that can be used in many fields, including image recognition. This article will introduce how to use Python regular expressions for image recognition. Regular expressions are a text pattern matching tool used to find text that matches a specific pattern. Python has a built-in "re" module for regular expressions
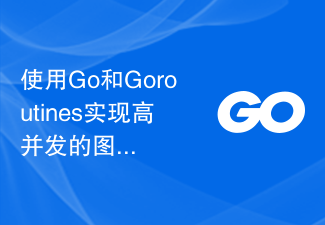
Using Go and Goroutines to implement a highly concurrent image recognition system Introduction: In today's digital world, image recognition has become an important technology. Through image recognition, we can convert information such as objects, faces, scenes, etc. in images into digital data. However, for recognition of large-scale image data, speed often becomes a challenge. In order to solve this problem, this article will introduce how to use Go language and Goroutines to implement a high-concurrency image recognition system. Background: Go language
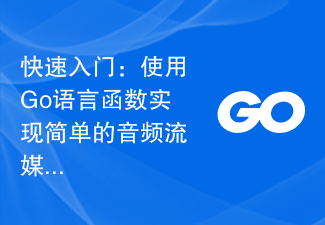
Quick Start: Implementing a Simple Audio Streaming Service Using Go Language Functions Introduction: Audio streaming services are becoming more and more popular in today's digital world, which allow us to play audio files directly over the network without performing a complete download. This article will introduce how to use Go language functions to quickly implement a simple audio streaming service so that you can better understand and use this function. Step 1: Preparation First, you need to install the Go language development environment. You can download it from the official website (https://golan

How to do image processing and recognition in Python Summary: Modern technology has made image processing and recognition an important tool in many fields. Python is an easy-to-learn and use programming language with rich image processing and recognition libraries. This article will introduce how to use Python for image processing and recognition, and provide specific code examples. Image processing: Image processing is the process of performing various operations and transformations on images to improve image quality, extract information from images, etc. PIL library in Python (Pi
