


PHP data filtering: How to prevent file upload vulnerabilities
PHP Data Filtering: How to Prevent File Upload Vulnerabilities
The file upload function is very common in web applications, but it is also one of the most vulnerable functions. Attackers may exploit file upload vulnerabilities to upload malicious files, leading to security issues such as server system intrusion, user data being leaked, or malware spreading. In order to prevent these potential threats, we should strictly filter and inspect files uploaded by users.
- Verify file type
An attacker may rename the .txt file to a .php file, upload it to the server, and then execute it by directly accessing the file Malicious code. To prevent this from happening, we need to verify the type of file the user uploads to make sure it is the type we expect.
Here is a simple code example to verify the file type:
function checkFileType($file) { $allowedTypes = array('image/jpeg', 'image/png', 'image/gif'); if (in_array($file['type'], $allowedTypes)) { return true; } return false; } // 检查上传的文件 if (isset($_FILES['file'])) { if (checkFileType($_FILES['file'])) { // 文件类型合法,继续处理 } else { // 文件类型不合法,抛出错误或进行其他操作 } }
- Check the file size
An attacker may upload a file that is too large files, causing server storage space to run out or system to crash. We should limit the size of files uploaded by users and check them.
Here is a simple code example for checking file size:
function checkFileSize($file) { $maxSize = 1024 * 1024; // 限制文件大小为1MB if ($file['size'] <= $maxSize) { return true; } return false; } // 检查上传的文件 if (isset($_FILES['file'])) { if (checkFileSize($_FILES['file'])) { // 文件大小合法,继续处理 } else { // 文件大小不合法,抛出错误或进行其他操作 } }
- Preventing file upload vulnerabilities
In addition to verifying file type and file size In addition, we also need to prevent file upload vulnerabilities. An attacker could potentially execute arbitrary code by uploading a file containing malicious code. To prevent this from happening, we can use the following methods:
- Save the uploaded file in a non-Web-accessible directory, which prevents attackers from directly accessing the uploaded file;
- Use randomly generated file names and unique file paths to avoid malicious scripts from directly accessing uploaded files;
- Set appropriate file permissions to ensure that uploaded files are not executable.
The following is a simple code example to implement the above method:
function saveUploadedFile($file) { $uploadDir = '/path/to/uploads/'; $filename = uniqid() . '_' . $file['name']; $targetFile = $uploadDir . $filename; // 将上传的文件保存在指定目录 if (move_uploaded_file($file['tmp_name'], $targetFile)) { // 文件保存成功,继续处理 } else { // 文件保存失败,抛出错误或进行其他操作 } } // 检查上传的文件 if (isset($_FILES['file'])) { if (checkFileType($_FILES['file']) && checkFileSize($_FILES['file'])) { saveUploadedFile($_FILES['file']); } else { // 文件类型或大小不合法,抛出错误或进行其他操作 } }
Summary
By strictly filtering and checking user uploaded files, we Can effectively prevent file upload vulnerabilities. Verifying file types, file sizes, and preventing file upload vulnerabilities are important steps in securing web applications. At the same time, we should also keep the code updated and strengthen security awareness to ensure the continued security of web applications.
The above is the detailed content of PHP data filtering: How to prevent file upload vulnerabilities. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
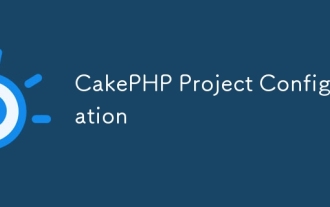
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
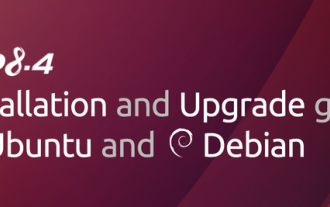
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
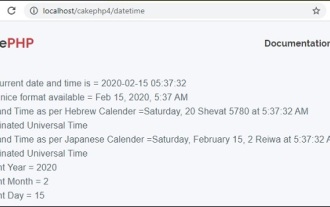
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
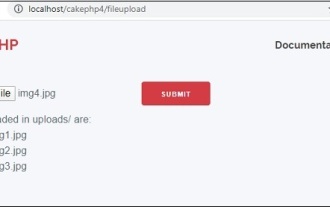
To work on file upload we are going to use the form helper. Here, is an example for file upload.
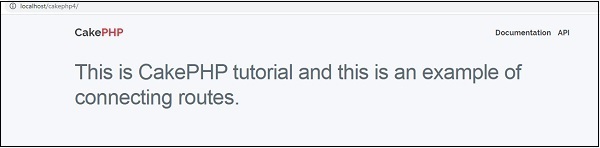
In this chapter, we are going to learn the following topics related to routing ?
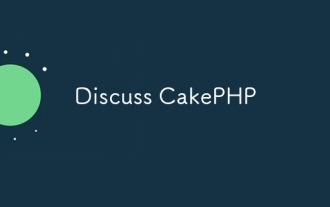
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
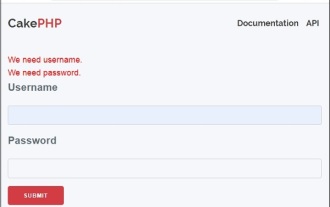
Validator can be created by adding the following two lines in the controller.
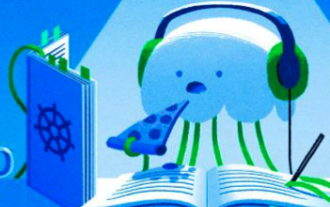
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
