


How to use network programming functions in Go language to implement FTP server downloading files?
How to use network programming functions in Go language to implement FTP server downloading files?
FTP (File Transfer Protocol) is a standard protocol for file transfer on the network. In actual development, we often encounter situations where we need to download files from an FTP server. Using the network programming functions in the Go language, we can easily implement the file download function of the FTP server. This article will introduce how to use Go language to implement FTP server file download, and attach code examples.
First, we need to import the net
and io
packages. The net
package provides some functions for network programming, while the io
package provides some functions for file and IO operations.
package main import ( "fmt" "io" "net" )
Next, we define a downloadFile
function to download files from the FTP server. This function receives three parameters: the address of the FTP server ftpAddr
, the name of the file to be downloaded fileName
, and the name of the locally saved file saveAs
.
func downloadFile(ftpAddr string, fileName string, saveAs string) error { // 建立与FTP服务器的连接 conn, err := net.Dial("tcp", ftpAddr) if err != nil { return fmt.Errorf("无法连接到FTP服务器:%v", err) } defer conn.Close() // 发送登录请求 conn.Write([]byte("USER anonymous ")) conn.Write([]byte("PASS ")) // 打开被动模式 conn.Write([]byte("PASV ")) // 接收服务器的响应 reply := make([]byte, 1024) _, err = conn.Read(reply) if err != nil { return fmt.Errorf("FTP服务器响应错误:%v", err) } // 解析服务器的响应,获取数据传输的 IP 和端口 ip, port, err := parseFtpReply(string(reply)) if err != nil { return fmt.Errorf("解析FTP服务器响应错误:%v", err) } // 建立与数据传输的服务器的连接 dataConn, err := net.Dial("tcp", fmt.Sprintf("%s:%d", ip, port)) if err != nil { return fmt.Errorf("无法连接到FTP服务器:%v", err) } defer dataConn.Close() // 发送下载文件的命令 conn.Write([]byte("RETR " + fileName + " ")) // 创建本地文件 file, err := os.Create(saveAs) if err != nil { return fmt.Errorf("无法创建本地文件:%v", err) } defer file.Close() // 从数据连接中读取文件内容,写入本地文件 _, err = io.Copy(file, dataConn) if err != nil { return fmt.Errorf("下载文件失败:%v", err) } return nil }
In the above code, we first establish a connection with the FTP server and send a login request. Then, send the PASV
command to tell the server that we want to use passive mode for data transmission. Next, we parse the server's response and obtain the IP and port for data transmission. After that, we establish a connection to the data transfer server and send the command to download the file. Finally, we create the local file and read the file content from the data connection and write it to the local file.
Next, we implement an auxiliary function parseFtpReply
for parsing the response from the FTP server.
func parseFtpReply(reply string) (string, int, error) { // 解析服务器响应 // 样例响应: 227 Entering Passive Mode (192,168,1,100,20,155) re := regexp.MustCompile(`(d+),(d+),(d+),(d+),(d+),(d+)`) matches := re.FindStringSubmatch(reply) if matches == nil { return "", 0, fmt.Errorf("解析服务器响应错误") } // 解析 IP 和端口 ip := strings.Join(matches[1:5], ".") portHighBits, _ := strconv.Atoi(matches[5]) portLowBits, _ := strconv.Atoi(matches[6]) port := portHighBits*256 + portLowBits return ip, port, nil }
In the above code, we use regular expressions to parse the response of the FTP server and obtain the IP and port of the data transmission.
Finally, we write a main
function to test our downloadFile
function.
func main() { ftpAddr := "ftp.example.com:21" fileName := "example.txt" saveAs := "local_file.txt" err := downloadFile(ftpAddr, fileName, saveAs) if err != nil { fmt.Println("下载文件失败:", err) } else { fmt.Println("文件下载成功!") } }
In the above code, we specify the address of the FTP server, the file name to be downloaded and the file name saved locally, and call the downloadFile
function to download the file.
Through the above code examples, we have successfully used the network programming functions in the Go language to implement the file download function of the FTP server. I hope this article can help you understand network programming and how to use Go language to download FTP files.
The above is the detailed content of How to use network programming functions in Go language to implement FTP server downloading files?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


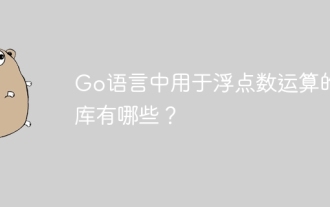
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
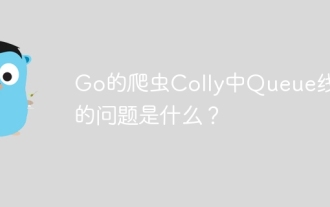
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
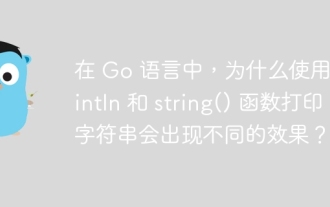
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
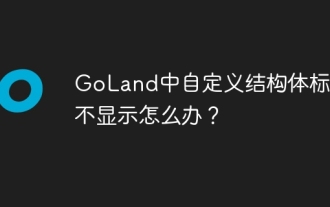
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
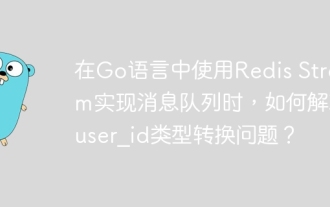
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
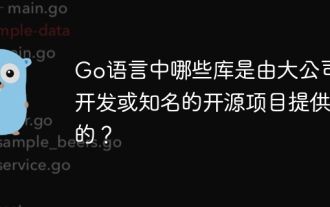
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
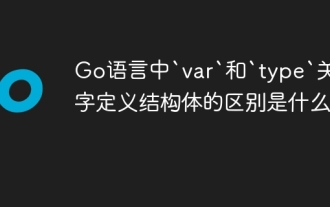
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
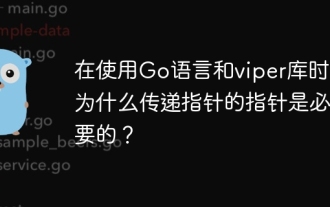
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
