


PHP Exception Handling Guide: How to use the set_exception_handler function to handle uncaught exceptions
PHP Exception Handling Guide: How to use the set_exception_handler
function to handle uncaught exceptions
Introduction: Exception handling is a very important part of any programming language. As a widely used server-side scripting language, PHP also provides rich exception handling functions. Among them, the set_exception_handler
function is an important tool in PHP for handling uncaught exceptions. This article will introduce how to use the set_exception_handler
function and show how to handle uncaught exceptions through code examples.
- Basic concept of exceptions
In PHP, exceptions refer to errors or unexpected situations that occur during program execution. When an exception occurs, if appropriate handling is not performed, the program will interrupt execution and display the system's default error message in the browser. To handle exceptions gracefully, PHP providestry-catch
blocks where exceptions can be caught and handled. However, in some cases, we want to perform custom processing of exceptions that cannot be caught, in which case we need to use theset_exception_handler
function. -
Usage of set_exception_handler function
set_exception_handler
is a function provided by the PHP core library for setting a custom exception handling function. This function accepts a callback function as a parameter, which will be called when an uncaught exception occurs. The following is the syntax of theset_exception_handler
function:bool set_exception_handler ( callable $exception_handler )
Copy after loginAmong them,
exception_handler
is a callback function used to handle uncaught exceptions. The callback function accepts only one parameter, the exception object itself. The return value of the callback function is of typebool
, used to indicate whether execution should be terminated.
The following is a simple example showing how to use the set_exception_handler
function to handle uncaught exceptions:
function exceptionHandler($exception) { echo "发生异常:". $exception->getMessage(); } set_exception_handler("exceptionHandler"); throw new Exception("测试异常");
In the above code, we first A function named exceptionHandler
is defined to handle uncaught exceptions. In the function body, we obtain the exception error message through $exception->getMessage()
and output it. Next, we set the exceptionHandler
function to a custom exception handling function by calling the set_exception_handler
function. Finally, we throw a test exception by throw new Exception
. Executing the above code, you can see that the abnormal error message is printed.
More uses of exception handling
In addition to simply outputting exception information, theset_exception_handler
function can also be used for more complex exception handling. For example, record exception information to a log file, or send exception notification emails to developers, etc. The following is an example of writing exception information to a log file:function exceptionHandler($exception) { $message = "发生异常:" . $exception->getMessage(); // 将异常信息写入日志文件 error_log($message, 3, "error.log"); } set_exception_handler("exceptionHandler"); throw new Exception("测试异常");
Copy after loginIn the above code, we use the
error_log
function to write the exception information to a file namederror.log
in the log file. In this way, we can easily view and track exception information for debugging and troubleshooting.- The calling sequence of multiple exception handling functions
If theset_exception_handler
function is called multiple times in the program, the last call will overwrite the previous setting and become the program The current exception handling function. This means that when an uncaught exception occurs, only the function set by the last call will be executed.
The following is an example that demonstrates the effect of calling the set_exception_handler
function multiple times:
function exceptionHandler1($exception) { echo "异常处理函数1"; } function exceptionHandler2($exception) { echo "异常处理函数2"; } // 第一次调用 set_exception_handler("exceptionHandler1"); // 第二次调用 set_exception_handler("exceptionHandler2"); throw new Exception("测试异常");
In the above code, we first called set_exception_handler( "exceptionHandler1")
, and then called set_exception_handler("exceptionHandler2")
. In the end, the program output result is "Exception Handling Function 2", indicating that the exception handling function set by the second call overwrites the result of the first call.
Summary:
By using the set_exception_handler
function, we can customize the handling of uncaught exceptions. Whether it is simply outputting exception information or performing more complex processing, it can be achieved by setting a custom exception handling function. At the same time, we can also write exception information to log files, send exception notifications, etc. as needed. Mastering exception handling skills can make our PHP applications more robust and reliable.
The above is the detailed content of PHP Exception Handling Guide: How to use the set_exception_handler function to handle uncaught exceptions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


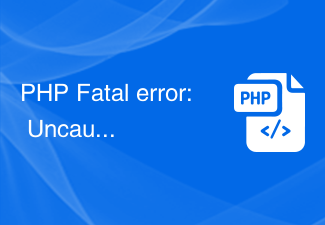
PHP is a widely used server-side programming language that provides powerful and dynamic capabilities to websites. However, in practice, developers may encounter a wide variety of errors and exceptions. One of the common errors is PHPFatalerror:Uncaughtexception'Exception'. In this article, we will explore the causes of this error and how to fix it. The concept of exception In PHP, exception refers to the unexpected situation encountered during the running process of the program, resulting in
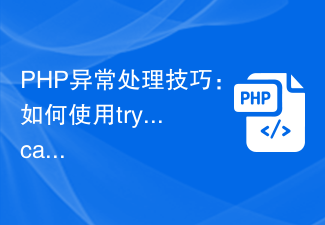
PHP exception handling tips: How to use try...catch blocks to catch and handle multiple exceptions Introduction: In PHP application development, exception handling is a very important part. When an error or exception occurs in the code, reasonable exception handling can improve the robustness and reliability of the program. This article will introduce how to use the try...catch block to capture and handle multiple exceptions, helping developers perform more flexible and efficient exception handling. Introduction to Exception HandlingExceptions refer to errors or special situations that occur when a program is running. When an exception occurs
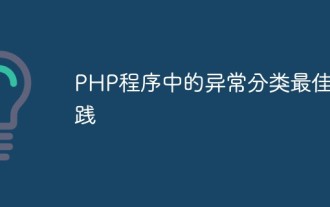
When writing PHP code, exception handling is an integral part of making the code more robust and maintainable. However, exception handling also needs to be used with caution, otherwise it may cause more problems. In this article, I will share some best practices for exception classification in PHP programs to help you make better use of exception handling to improve code quality. The concept of exception In PHP, exception refers to an error or unexpected situation that occurs when the program is running. Typically, exceptions cause the program to stop running and output an exception message.
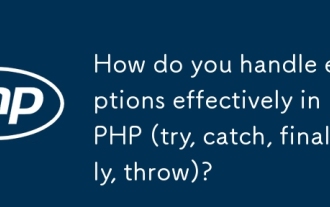
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
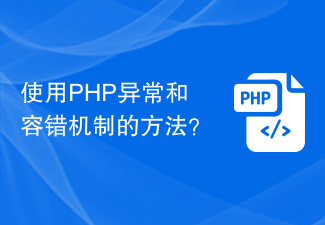
How to use PHP's exception handling and fault tolerance mechanism? Introduction: In PHP programming, exception handling and fault tolerance mechanisms are very important. When errors or exceptions occur during code execution, exception handling can be used to capture and handle these errors to ensure program stability and reliability. This article will introduce how to use PHP's exception handling and fault tolerance mechanism. 1. Basic knowledge of exception handling: What is an exception? Exceptions are errors or abnormal conditions that occur during code execution, including syntax errors, runtime errors, logic errors, etc. When different
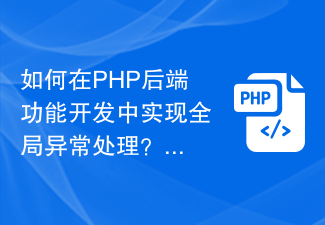
How to implement global exception handling in PHP backend function development? In PHP back-end development, exception handling is a very important part. It can help us catch errors in the program and handle them appropriately, thereby improving system stability and performance. This article will introduce how to implement global exception handling in PHP back-end function development and provide corresponding code examples. PHP provides an exception handling mechanism. We can catch exceptions and handle them accordingly through the try and catch keywords. Global exception handling refers to all
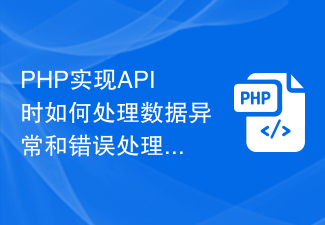
As the use of APIs becomes more and more widespread, we also need to consider data exception and error handling strategies during the development and use of APIs. This article will explore how PHP handles these issues when implementing APIs. 1. Handling data anomalies There may be many reasons for data anomalies, such as user input errors, network transmission errors, internal server errors, etc. When developing in PHP, we can use the following methods to handle data exceptions. Return the appropriate HTTP status code. The HTTP protocol defines many status codes that can help us
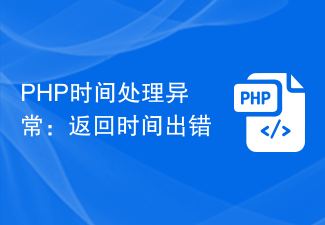
PHP time processing exception: error in returning time, specific code examples are needed. In web development, time processing is a very common requirement. As a commonly used server-side scripting language, PHP provides a wealth of time processing functions and methods. However, in practical applications, sometimes you encounter abnormal situations where the return time is wrong, which may be caused by errors in the code or improper use. In this article, we will introduce some common situations that may cause return time errors and provide some specific code examples to help readers better understand
