


How to use php to extend SQLite for lightweight database management
How to use PHP to extend SQLite for lightweight database management
Introduction:
SQLite is a lightweight embedded database engine that supports creating and managing databases locally or in memory. It does not require any server and is very convenient to use. In PHP, we can use SQLite extensions to operate SQLite databases. This article will introduce how to use PHP to extend SQLite for lightweight database management and provide some code examples.
Part One: Install SQLite Extension and SQLite Database
Before we begin, we need to make sure that PHP's SQLite extension and SQLite database have been installed. In most PHP installations, the SQLite extension is already installed by default. If you are using an older version of PHP, you can enable the SQLite extension via compile options. The process of installing a SQLite database is similar to installing other software packages. You can download the latest stable version from the SQLite official website (https://www.sqlite.org/).
Part 2: Connect to the SQLite database
Before using the SQLite database, we need to connect to the database first. The following is a sample code to connect to the database:
<?php $db = new SQLite3('database.db');
The above code will create a database file named database.db in the current directory and connect it. If the database file already exists, the code will connect to the existing database. Otherwise, it automatically creates a new, empty database.
Part 3: Create tables and insert data
After connecting to the database, we can create tables and insert data. The following is a sample code:
<?php $db->exec('CREATE TABLE users (id INTEGER PRIMARY KEY, name TEXT, email TEXT)'); $db->exec("INSERT INTO users (name, email) VALUES ('John', 'john@example.com')"); $db->exec("INSERT INTO users (name, email) VALUES ('Jane', 'jane@example.com')");
The above code creates a table named users and inserts two pieces of data into the table.
Part 4: Querying Data
After inserting data, we can use various query statements to retrieve data from the database. The following are some commonly used query sample codes:
<?php $result = $db->query('SELECT * FROM users'); while ($row = $result->fetchArray()) { echo "ID: {$row['id']}, Name: {$row['name']}, Email: {$row['email']} "; } // 查询特定条件的数据 $result = $db->query("SELECT * FROM users WHERE name = 'John'"); while ($row = $result->fetchArray()) { echo "ID: {$row['id']}, Name: {$row['name']}, Email: {$row['email']} "; }
In the above code, we use the SELECT statement to retrieve data from the users table. The first example code retrieves all the data, the second example code retrieves only the data with the name "John".
Part 5: Update and delete data
In addition to querying data, we can also update and delete data in the database. The following are some commonly used update and delete sample codes:
<?php // 更新数据 $db->exec("UPDATE users SET email = 'new_email@example.com' WHERE id = 2"); // 删除数据 $db->exec("DELETE FROM users WHERE id = 1");
In the above code, we use the UPDATE statement to update the email of the user with id 2, and use the DELETE statement to delete the user with id 1.
Conclusion:
This article introduces how to use PHP to extend SQLite for lightweight database management. We learned the basic operations of connecting to a database, creating tables and inserting data, querying data, and updating and deleting data. I hope these sample codes will be helpful for you to learn SQLite database management.
The above is the detailed content of How to use php to extend SQLite for lightweight database management. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


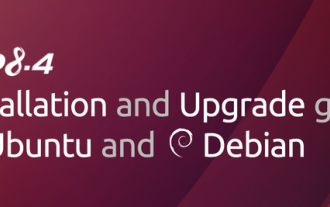
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
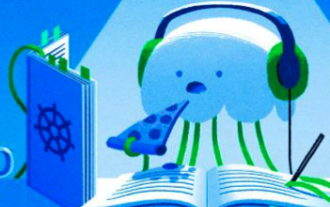
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
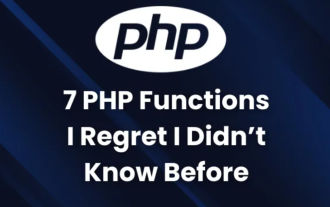
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
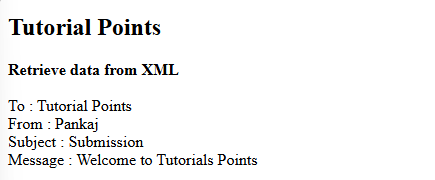
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
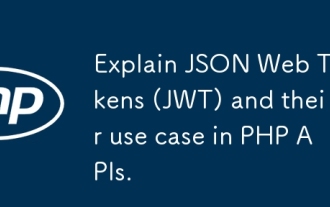
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
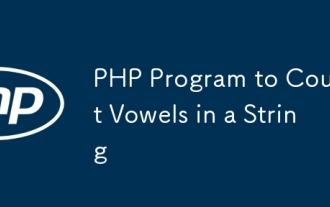
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
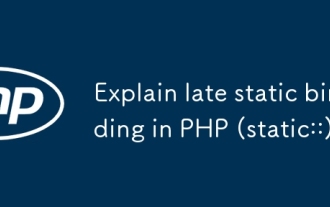
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
