


Quick Start: Use Go language functions to implement simple video processing functions
Quick Start: Use Go language functions to implement simple video processing functions
Introduction:
In today's digital era, video has become an indispensable part of our lives. However, processing videos requires efficient algorithms and powerful computing power. As a simple and efficient programming language, Go language also shows its unique advantages in video processing. This article will introduce how to use Go language functions to implement simple video processing functions, and use code examples to let everyone understand the process more intuitively.
Step 1: Import related packages
To perform video processing, you first need to import the relevant Go language package. In the Go language, there are many third-party libraries for video processing available, such as ffmpeg, gocv, etc. In this article, we will use the gocv package to process videos.
The sample code is as follows:
import "gocv.io/x/gocv"
Step 2: Load the video file
To process the video, you first need to load the video from the file. Video files can be easily read and converted into images using the imread()
function of the gocv package.
The sample code is as follows:
func LoadVideo(filename string) *gocv.VideoCapture { video, _ := gocv.VideoCaptureFile(filename) return video }
Step 3: Get the video frame
When processing the video, we need to read the images in the video frame by frame and process each frame. Use the Grab()
function of the gocv package to get the image of the next frame.
The sample code is as follows:
func GetFrame(video *gocv.VideoCapture) gocv.Mat { frame := gocv.NewMat() video.Grab(1) video.Read(&frame) return frame }
Step 4: Process the video frame
After obtaining the video frame, we can perform various processing on it, such as adjusting brightness, sharpening the image, etc. Here, we take adjusting brightness as an example to demonstrate how to process video frames.
The sample code is as follows:
func AdjustBrightness(frame gocv.Mat, value float64) gocv.Mat { newFrame := gocv.NewMat() frame.ConvertTo(&newFrame, -1, 1, value) return newFrame }
Step 5: Save the video frame
After processing the video frame, we can save it to a new video file. Images can be saved as files using the imWrite()
function of the gocv package.
The sample code is as follows:
func SaveFrame(frame gocv.Mat, filename string) { gocv.IMWrite(filename, frame) }
Step 6: Process the entire video
Now that we have understood how to process a single video frame, next we need to process the entire video. Use a loop to process the video frame by frame and save the processed frames.
The sample code is as follows:
func ProcessVideo(filename string, outputFilename string, brightness float64) { video := LoadVideo(filename) for { frame := GetFrame(video) if frame.Empty() { break } adjustedFrame := AdjustBrightness(frame, brightness) SaveFrame(adjustedFrame, outputFilename) frame.Close() adjustedFrame.Close() } video.Close() }
Conclusion:
This article introduces how to use Go language functions to implement simple video processing functions. By loading the video, getting the frame image, processing the image, and saving the image, we can perform various operations on the video. The above code examples can help readers better understand and use Go language for video processing. I hope readers can apply this knowledge in actual projects to further optimize and expand video processing functions.
The above is the detailed content of Quick Start: Use Go language functions to implement simple video processing functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


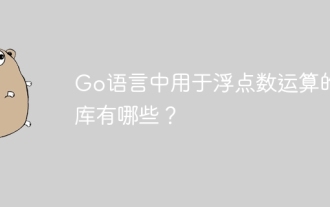
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
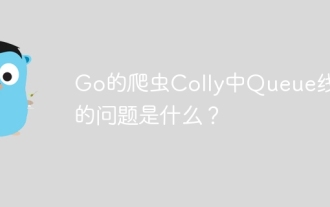
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
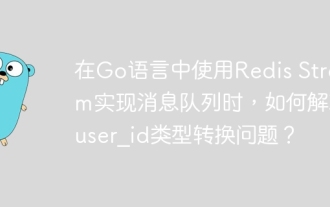
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
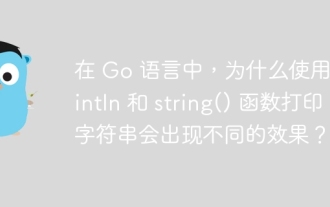
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
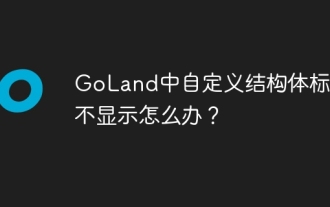
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
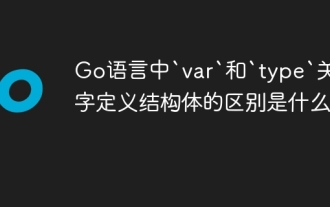
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
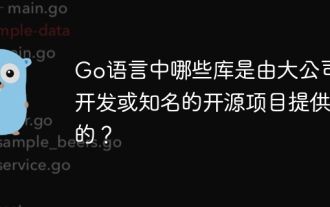
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
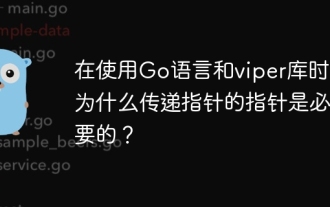
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
