


PHP data filtering tips: How to use the filter_var function to validate user input
PHP data filtering skills: How to use the filter_var function to verify user input
In Web development, the verification and filtering of user input data are very important links. Malicious input may be exploited by malicious users to attack or compromise the system. PHP provides a series of filter functions to help us process user input data, the most commonly used of which is the filter_var function.
The filter_var function is a filter-based way of validating user input. It allows us to validate and filter user input using various built-in filters. Here are some common filters and examples of their usage:
-
Validate integer
1
2
3
4
5
6
$input
=
$_POST
[
'input'
];
if
(filter_var(
$input
, FILTER_VALIDATE_INT)) {
// 输入是一个有效的整数
}
else
{
// 输入不是一个有效的整数
}
Copy after login Validate email
1
2
3
4
5
6
$email
=
$_POST
[
'email'
];
if
(filter_var(
$email
, FILTER_VALIDATE_EMAIL)) {
// 输入是一个有效的邮箱地址
}
else
{
// 输入不是一个有效的邮箱地址
}
Copy after loginValidate URL
1
2
3
4
5
6
$url
=
$_POST
[
'url'
];
if
(filter_var(
$url
, FILTER_VALIDATE_URL)) {
// 输入是一个有效的URL
}
else
{
// 输入不是一个有效的URL
}
Copy after loginFilter HTML tags
1
2
$input
=
$_POST
[
'input'
];
$filtered_input
= filter_var(
$input
, FILTER_SANITIZE_STRING);
Copy after login
When using the filter_var function, we need to specify the user input and requirements to be verified The type of filter to use. If the verification passes, the function will return the filtered value; if the verification fails, the function will return false.
In addition to the filters in the above examples, PHP also provides many other filters, such as filtering IP addresses, filtering floating point numbers, filtering specific characters, etc. We can choose the appropriate filter type to validate user input according to actual needs.
In addition, we can also achieve more precise verification by using a combination of filters. For example, we can limit the minimum value of the input value by using the FILTER_VALIDATE_INT filter and the FILTER_FLAG_MIN_RANGE flag:
1 2 3 4 5 6 7 |
|
In practical applications, we usually validate multiple user input data, in order to reduce redundant code , we can encapsulate a verification function to uniformly handle the verification of input data:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
When writing code to verify and filter user input, you also need to pay attention to the following points:
- Validation and filtering of user input should be done on the server side, do not rely on client-side validation.
- Don't trust user input, always perform necessary validation and filtering.
- Validating and filtering user input should be based on specific application scenarios. Different applications may require different validation rules.
To summarize, using the filter_var function can help us verify and filter user input data, thereby improving the security of the application. In practical applications, we can choose the appropriate filter type as needed and customize verification rules according to specific circumstances. Reasonable use of the filter_var function can help us better handle user input data and improve the robustness and security of the application.
The above is the detailed content of PHP data filtering tips: How to use the filter_var function to validate user input. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


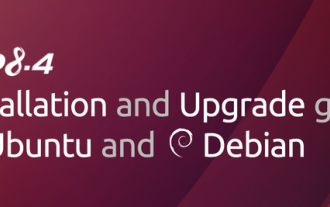
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
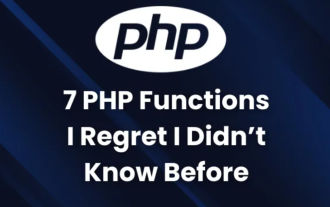
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
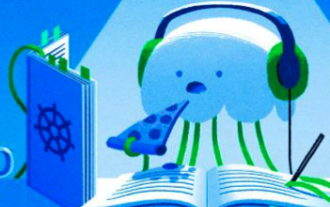
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
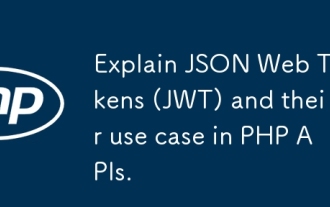
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
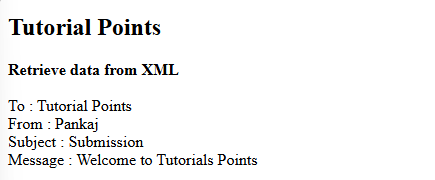
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
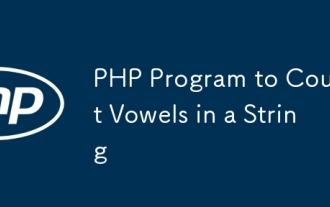
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
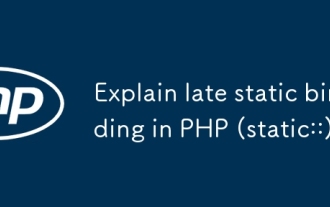
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
