How to use Java code to display traffic information on Baidu Maps?
How to use Java code to display traffic information on Baidu Map?
Baidu Map is a commonly used map application that provides rich map data and functions. Displaying traffic information in the app can help users better plan travel routes. This article will introduce how to use Java code to display traffic information on Baidu Maps.
First of all, we need to prepare some necessary tools and resources. First, you need to obtain the API key of Baidu Maps, which can be obtained through registration and application on Baidu Open Platform. Secondly, we need to download the Java SDK of Baidu Maps, which provides rich Java code examples and documents to help developers use Baidu Maps functions.
Before we start writing code, we need to import Baidu Map’s Java SDK into the project. You can import the downloaded SDK directly into a Java project, or use tools such as Maven for dependency management.
Next, we can write Java code to display traffic information on Baidu Maps. The following is a simple example:
import com.baidu.mapapi.MapStatusUpdateFactory; import com.baidu.mapapi.SDKInitializer; import com.baidu.mapapi.map.BaiduMap; import com.baidu.mapapi.map.MapView; import com.baidu.mapapi.overlayutil.TrafficOverlay; import javax.swing.*; import java.awt.event.WindowAdapter; import java.awt.event.WindowEvent; public class TrafficMapExample { public static void main(String[] args) { // 初始化地图SDK SDKInitializer.initialize(); // 创建地图视图 MapView mapView = new MapView(null); // 获取百度地图对象 BaiduMap baiduMap = mapView.getMap(); // 显示交通图层 baiduMap.setTrafficEnabled(true); // 设置地图中心点和缩放级别 baiduMap.animateMapStatus(MapStatusUpdateFactory.newLatLngZoom(MapWrapper.BEIJING, 13)); // 创建窗口 JFrame frame = new JFrame("百度地图 - 路况信息示例"); frame.setSize(800, 600); frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); frame.addWindowListener(new WindowAdapter() { @Override public void windowClosing(WindowEvent e) { // 释放地图资源 mapView.onDestroy(); } }); // 将地图视图添加到窗口中 frame.getContentPane().add(mapView); // 显示窗口 frame.setVisible(true); } }
The above code creates a simple window that displays a Baidu map and turns on the display of traffic information. By calling the baiduMap.setTrafficEnabled(true)
method, we can display real-time traffic information on the map. At the same time, use the baiduMap.animateMapStatus(MapStatusUpdateFactory.newLatLngZoom(MapWrapper.BEIJING, 13))
method to set the center point and zoom level of the map.
Before using this code, please make sure you have correctly imported Baidu Map's Java SDK and replace the API key and map center point coordinates in the code.
Through the above simple example, we can easily implement the function of displaying traffic information on Baidu Maps in a Java application. At the same time, Baidu Map's Java SDK also provides other rich functions and interfaces, which developers can expand and customize according to their own needs.
The above is the detailed content of How to use Java code to display traffic information on Baidu Maps?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


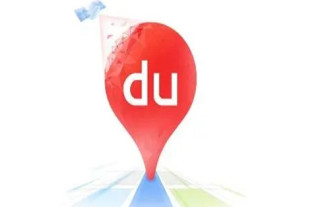
Baidu Map APP has now become the preferred travel navigation software for many users, so some of the functions here are comprehensive and can be selected and operated for free to solve some of the problems that you may encounter in daily travel. You can all check some of your own travel routes and plan some of your own travel plans. After checking the corresponding routes, you can choose appropriate travel methods according to your own needs. So whether you choose some public transportation, Cycling, walking or taking a taxi can all satisfy your needs. There are corresponding navigation routes that can successfully lead you to a certain place. Then everyone will feel more convenient if they choose to take a taxi. There are many drivers They are all able to take orders online, and taxi-hailing has become super
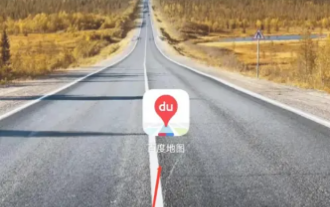
In daily travel, we often need to take a taxi, and now Baidu Maps also provides a taxi service, which is convenient and fast. However, many people still don’t know how to pay after taking a taxi on Baidu Maps. Below, we will introduce in detail how to pay for taxis on Baidu Maps. How to pay for a taxi on Baidu Map 1. First open the Baidu Map APP and enter the main page; 2. Then jump to the page shown in the picture below and click [Taxi] on the right; 3. Then enter the taxi function page in the picture below , select [Personal Center]; 4. Then on the Personal Center page, find [Payment Management]; 5. Finally, on the payment management function page, select the payment method you want to activate and click [Go to Activate].
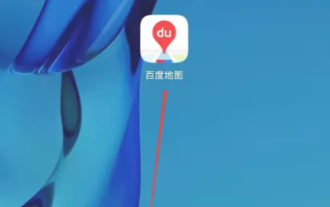
If you want to add a new location on Baidu Maps, you may need to go through some complicated steps. But don’t worry, next I will explain in detail how to add a new place on Baidu Maps, making it easier for you to share your location information or help others find their destination. How to add a new location on Baidu Map 1. First open the Baidu Map APP and enter the main page; 2. Then enter the main page as shown below and click the [Report] button on the right; 3. Then jump to the reporting function page , select the [Add Location] service below; 4. Then enter the information in the [Other Information] box in the Add Location area; 5. Finally enter the corresponding information and click [Submit] at the bottom to complete.
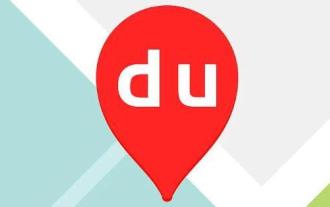
Baidu Maps has a 3D real-life map function, so how to view the 3D real-life map? Users need to find more options in My, and then find the 3D map in it to view the map. This introduction to the method of viewing 3D real-life maps can tell you how to set it up. The following is a detailed introduction, so take a look. Baidu Map usage tutorial How to view the 3D real-life map of Baidu Map Answer: Go to My-More-3D Map Specific method: Mobile version: 1. First, click My on the lower right. 2. Find more functions inside. 3. Click on the 3D real scene to use it. Web version: 1. First, you need to enter https://map.baidu.com to enter the web version. 2. Click View method in the lower right corner.
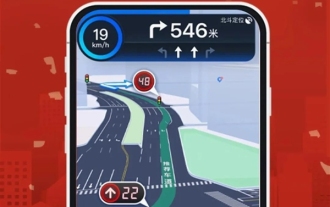
Baidu Maps recently announced that they have successfully launched a true city lane-level navigation system, which has covered more than 200 cities across the country. The introduction of this system has greatly improved the driver's navigation experience. Baidu Maps' lane-level navigation provides a more immersive and three-dimensional interface compared to previous navigation methods that only provide enlarged images. This system allows drivers to have a clearer understanding of the current road conditions by finely restoring real-world road details, such as traffic lights, lane dividing lines, and bus lanes. This kind of lane-level navigation can not only help drivers choose lanes more accurately, but also provide more comprehensive traffic information, making the driving process safer and more convenient. It is understood that in order to achieve this goal, Baidu Maps independently developed the industry's first large-scale map generation model.
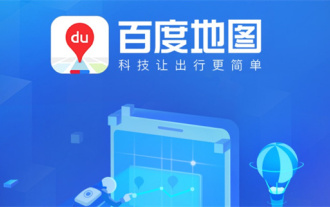
Baidu Map Navigation mobile version free download is a very professional mobile map navigation software. The navigation function in the software is very powerful. As long as you want to go, you can find the most accurate travel plan. It is very convenient to go wherever you want to go. At the same time, you can also use other navigation functions. You can download and use the voice packs of various celebrities for free. Various navigation modes can be used online to protect everyone's travel safety. You can also scan the code to take a bus more conveniently. It is a must-have travel navigation assistant. , don’t worry about getting lost, now the editor will carefully introduce to Baidu Map partners how to view 3D real-time street view online. 1. Open Baidu Maps and click More in the common function bar. 2. Then search to find the 3D real scene. 3. Then enter the 3D viewing interface. 4. Such as
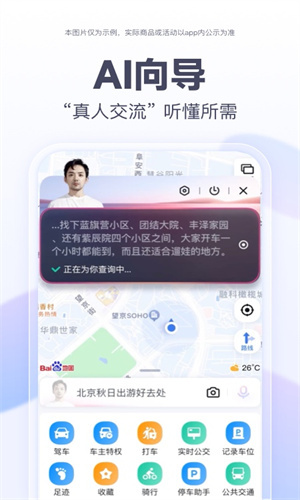
There are many functions above, especially for maps that can mark multiple places. When we know some places, we will definitely use some punctuation functions, so that we can bring you a variety of different aspects. Some of the functions you mark will produce distance differences, that is, you can know how far away they are. Of course, some names and detailed information of the above places will also be displayed. However, many netizens may not be familiar with some of the above. The content information is not very clear, so in order to allow everyone to make better choices in various aspects, today the editor will bring you some choices in various aspects, so friends who are interested in ideas, If you are also interested, come and give it a try. Standard
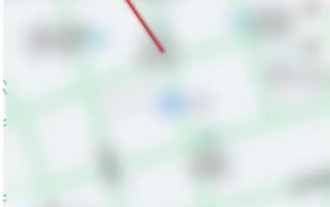
With the popularity of online taxi hailing, more and more people choose to use Baidu Maps to hail taxis. However, for users who need to reimburse or issue invoices, how to issue an invoice after taking a taxi on Baidu Map is a more important issue. This article will introduce you how to issue an invoice after taking a taxi on Baidu Maps. How to invoice Baidu Map Taxi 1. First open the Baidu Map APP and enter the [Avatar] in the upper left corner of the main page; 2. Then enter the personal center function page and select the [Taxi] function as shown below; 3. Then Go to the taxi function page and click the [Personal Center] button on the right; 4. Then in the personal center area, select [Invoicing]; 5. Then on the invoicing page, click [Travel Service Invoicing]; 6. Press the order On the invoicing function page, check the
