


Quick Start: Use Go language functions to implement simple task queue functions
Quick Start: Use Go language functions to implement simple task queue functions
Introduction:
In modern software development, task queue (Task Queue) is a very common concept, used to solve multiple problems. Requirements for concurrent execution of tasks. Task queues can help us implement asynchronous processing of tasks and improve the system's response speed and concurrency capabilities. This article will introduce how to use Go language functions to implement a simple task queue to help you get started quickly.
- Understanding task queue
The tasks in the task queue can be various work units, such as calculations, I/O, network requests, etc. The main purpose of the task queue is to manage and schedule these tasks according to certain strategies to achieve optimal system performance. - Use Go language functions to implement task queues
In order to implement the task queue function, we can use Go language goroutine and channel to complete.
First, we can define a structure to represent a task:
type Task struct { ID int Func func() error } // NewTask 创建一个新的任务 func NewTask(id int, f func() error) *Task { return &Task{ ID: id, Func: f, } }
Then, we need to define a structure for the task queue:
type TaskQueue struct { queue chan *Task }
Next , we can add some common methods to the task queue, such as adding tasks, executing tasks, etc.:
// Push 将任务添加到队列中 func (tq *TaskQueue) Push(task *Task) { tq.queue <- task } // Execute 从队列中取出任务并执行 func (tq *TaskQueue) Execute() { for task := range tq.queue { if err := task.Func(); err != nil { fmt.Printf("Task %d failed: %s ", task.ID, err.Error()) } } }
Finally, we can use the task queue to create and execute tasks:
func main() { // 创建任务队列 tq := TaskQueue{ queue: make(chan *Task), } // 启动并发的任务执行 go tq.Execute() // 添加任务到队列中 for i := 0; i < 10; i++ { id := i task := NewTask(id, func() error { time.Sleep(time.Second) fmt.Printf("Task %d executed ", id) return nil }) tq.Push(task) } // 等待所有任务执行完成 time.Sleep(11 * time.Second) }
above In the sample code, we create a task queue and add tasks to the queue by calling the Push
method. Then in the Execute
method, we can continuously remove tasks from the queue and execute them. Finally, we wait for all tasks to complete.
- Summary
This article introduces how to use Go language functions to implement a simple task queue and provides corresponding code examples. By mastering the basic concepts and usage of task queues, it can help us better handle the requirements for concurrent execution of multiple tasks and improve the performance and stability of the system. At the same time, readers can further expand the functions and features of the task queue according to their actual needs. I hope this article is helpful to you, thank you for reading!
The above is the detailed content of Quick Start: Use Go language functions to implement simple task queue functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


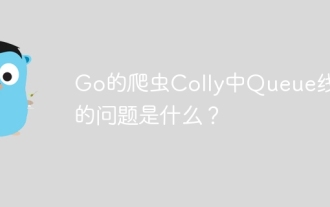
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
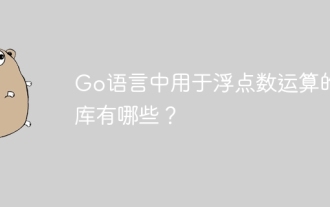
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
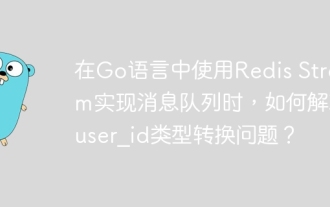
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
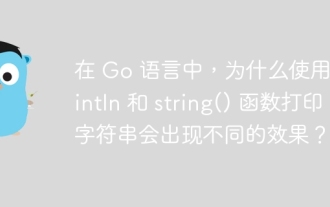
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
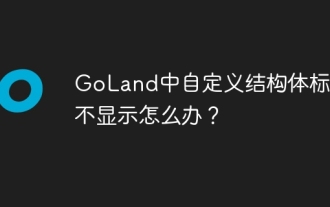
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
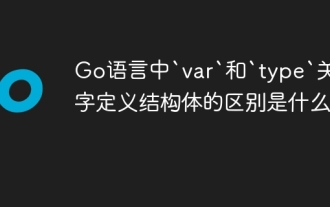
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
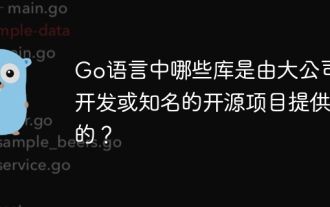
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
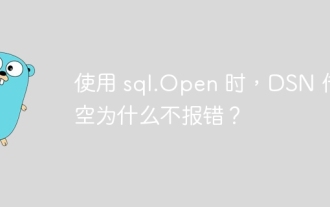
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
