Build a high-performance search engine using Redis and Ruby
Using Redis and Ruby to build a high-performance search engine
Search engines play a vital role in the modern Internet era, helping users quickly find the information they need. In order to implement a high-performance search engine, we can use two powerful tools, Redis and Ruby, to build it.
Redis is an in-memory database that is widely used in scenarios such as caching, message queues, and real-time analysis. Its high-speed reading and writing capabilities and support for high concurrency make it an ideal choice when building search engines. As a simple and elegant programming language, Ruby has rich web development frameworks, such as Rails, which can easily interact with Redis and provide comprehensive search functions.
First, we need to install and configure Redis. You can download the latest stable version from the official Redis website and install it according to the official guide. After the installation is complete, start the Redis service.
The following is an example, using Ruby and Redis to build a simple search engine:
The first step is to install the necessary dependent libraries and Gem packages:
require 'redis' require 'redis-namespace' require 'redis-search'
Second Step 1, configure Redis and Redis-Search:
# 连接到Redis服务器 redis = Redis.new(host: 'localhost', port: 6379) # 使用Redis命名空间 namespace = Redis::Namespace.new(:search_engine, redis: redis) # 配置Redis-Search redis_search = Redis::Search.configure do |config| config.redis = namespace config.complete_max_length = 100 end
Step 3, define a search model:
class Post < ActiveRecord::Base include Redis::Search # 设置搜索的字段 def self.search_fields [:title, :content] end end # 修改Post模型的字段 class AddSearchFieldsToPosts < ActiveRecord::Migration[6.0] def change # 添加搜索字段title和content add_column :posts, :title, :string add_column :posts, :content, :text # 设置Redis-Search Post.setup_redis_search( prefix: 'search_engine', stopwords: %w[in on the for] ) end end
Step 4, use the search engine to query:
# 创建索引 Post.rebuild_index # 查询 result = Post.search('Ruby') # 输出结果 result.each do |post| puts "标题:#{post.title}" puts "内容:#{post.content}" end
Through the above code examples, we can clearly understand how to use Redis and Ruby to build a high-performance search engine.
It should be noted that the above examples only show basic search functions. Actual search engines also need to consider more aspects, such as paging, sorting, filtering, etc. At the same time, other functions can be expanded according to specific needs, such as automatic completion, spelling correction, etc.
When building a search engine, in addition to Redis and Ruby, you can also consider other tools and technologies, such as Elasticsearch and Solr. Each tool has its own unique characteristics and applicable scenarios. It is very important to choose the right tool according to the specific needs of the project.
To sum up, it is feasible and effective to use Redis and Ruby to build a high-performance search engine. Redis provides powerful caching and high-speed reading and writing capabilities, and Ruby, as a powerful and elegant programming language, can easily interact with Redis and provide comprehensive search functions. I hope that through the introduction and examples of this article, readers can have a deeper understanding of how to build a high-performance search engine.
The above is the detailed content of Build a high-performance search engine using Redis and Ruby. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
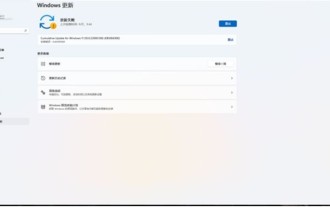
1. Start the [Start] menu, enter [cmd], right-click [Command Prompt], and select Run as [Administrator]. 2. Enter the following commands in sequence (copy and paste carefully): SCconfigwuauservstart=auto, press Enter SCconfigbitsstart=auto, press Enter SCconfigcryptsvcstart=auto, press Enter SCconfigtrustedinstallerstart=auto, press Enter SCconfigwuauservtype=share, press Enter netstopwuauserv , press enter netstopcryptS
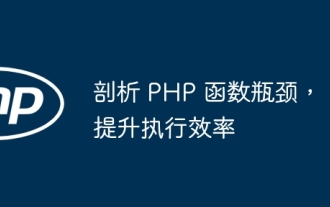
PHP function bottlenecks lead to low performance, which can be solved through the following steps: locate the bottleneck function and use performance analysis tools. Caching results to reduce recalculations. Process tasks in parallel to improve execution efficiency. Optimize string concatenation, use built-in functions instead. Use built-in functions instead of custom functions.
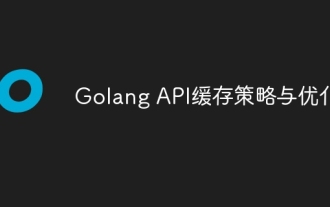
The caching strategy in GolangAPI can improve performance and reduce server load. Commonly used strategies are: LRU, LFU, FIFO and TTL. Optimization techniques include selecting appropriate cache storage, hierarchical caching, invalidation management, and monitoring and tuning. In the practical case, the LRU cache is used to optimize the API for obtaining user information from the database. The data can be quickly retrieved from the cache. Otherwise, the cache can be updated after obtaining it from the database.
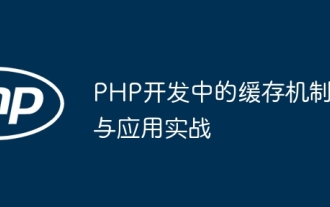
In PHP development, the caching mechanism improves performance by temporarily storing frequently accessed data in memory or disk, thereby reducing the number of database accesses. Cache types mainly include memory, file and database cache. Caching can be implemented in PHP using built-in functions or third-party libraries, such as cache_get() and Memcache. Common practical applications include caching database query results to optimize query performance and caching page output to speed up rendering. The caching mechanism effectively improves website response speed, enhances user experience and reduces server load.
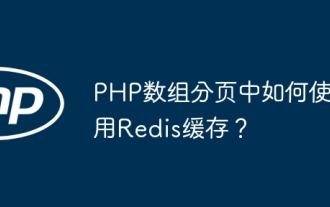
Using Redis cache can greatly optimize the performance of PHP array paging. This can be achieved through the following steps: Install the Redis client. Connect to the Redis server. Create cache data and store each page of data into a Redis hash with the key "page:{page_number}". Get data from cache and avoid expensive operations on large arrays.
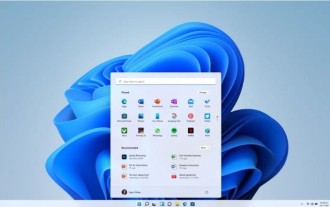
First you need to set the system language to Simplified Chinese display and restart. Of course, if you have changed the display language to Simplified Chinese before, you can just skip this step. Next, start operating the registry, regedit.exe, directly navigate to HKEY_LOCAL_MACHINESYSTEMCurrentControlSetControlNlsLanguage in the left navigation bar or the upper address bar, and then modify the InstallLanguage key value and Default key value to 0804 (if you want to change it to English en-us, you need First set the system display language to en-us, restart the system and then change everything to 0409) You must restart the system at this point.

Yes, Navicat can connect to Redis, which allows users to manage keys, view values, execute commands, monitor activity, and diagnose problems. To connect to Redis, select the "Redis" connection type in Navicat and enter the server details.
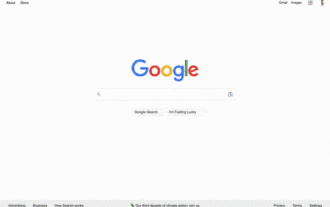
According to news from this site on June 26, according to SearchEngineLand, Google has now canceled the "continuous scrolling" display of the search results interface and switched to the "paging" mode that it has been using before. This site’s inquiry found that Google initially introduced “continuous scrolling” for mobile phones in October 2021, and then brought it to the desktop at the end of 2022. That said, "continuous scrolling" only lasted about a year and a half on desktop. A Google spokesperson told SearchEngineLand that the continuous scrolling feature will be removed from desktop search results today, and that the feature will be removed from mobile phones "in the coming months." As shown in the picture, Google has brought back the classic paging bar, allowing users to click on the number to jump to a specific page, or simply click "
