


How to build a highly available distributed system using Vue.js and Java language
How to use Vue.js and Java language to build a highly available distributed system
1. Introduction
With the rapid development of the Internet, distributed systems are becoming more and more important. Distributed systems can provide higher availability, scalability, and fault tolerance to meet the needs of modern applications. In this article, we will introduce how to use Vue.js and Java language to build a highly available distributed system.
2. What are Vue.js and Java language?
Vue.js is a popular JavaScript framework for building user interfaces. It's easy to use, performs well, and has tons of community support. Vue.js adopts a component-based development approach, allowing developers to easily build complex user interfaces.
The Java language is a widely used programming language and is widely used in enterprise-level application development. It has a rich library and tools that provide great power and reliability, making it ideal for building distributed systems.
3. Steps to build a highly available distributed system
1. Design system architecture
First, we need to design the architecture of the distributed system. A highly available distributed system needs to consider the following aspects:
- Load balancing: Ensure that the system can balance the load and be able to scale horizontally as needed.
- High availability: Each component in the system needs to be designed to be fault-tolerant and automatically recover.
- Data consistency: Ensure that the data in the system is consistent, whether on a single node or multiple nodes.
2. Use Vue.js to build the user interface on the front end
Using Vue.js to build the user interface can provide a good user experience. Vue.js provides a large number of components and tools that allow developers to easily build complex user interfaces. The following is a simple Vue.js component example:
<template> <div> <h1>{{ title }}</h1> <ul> <li v-for="item in items" :key="item.id">{{ item.name }}</li> </ul> </div> </template> <script> export default { data() { return { title: 'Hello World', items: [ { id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }, { id: 3, name: 'Item 3' } ] } } } </script> <style scoped> h1 { color: red; } </style>
3. The backend uses Java to build business logic and data processing
In the backend, we can use the Java language to build business logic and process data. Java provides a wealth of libraries and tools that allow developers to easily handle various complex tasks. The following is a simple Java example:
@RestController @RequestMapping("/api/items") public class ItemController { private final ItemRepository itemRepository; public ItemController(ItemRepository itemRepository) { this.itemRepository = itemRepository; } @GetMapping public List<Item> getAllItems() { return itemRepository.findAll(); } @PostMapping public Item createItem(@RequestBody Item item) { return itemRepository.save(item); } }
4. Use Spring Boot to build distributed systems
Spring Boot is a popular Java framework for building microservices and distributed systems. It provides a wealth of features and tools that allow developers to easily build highly available distributed systems. The following is a simple Spring Boot application example:
@SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } }
5. Summary
In this article, we introduced how to use Vue.js and Java language to build a highly available distributed system. Through reasonable system architecture design and selection of appropriate frameworks and tools, we can build distributed systems that meet the needs of modern applications. I hope these code examples will help you build distributed systems.
The above is the detailed content of How to build a highly available distributed system using Vue.js and Java language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


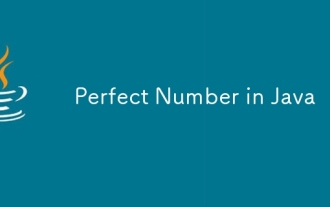
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
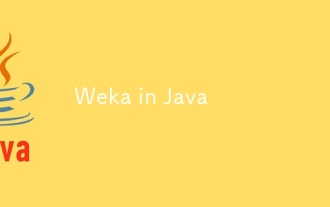
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
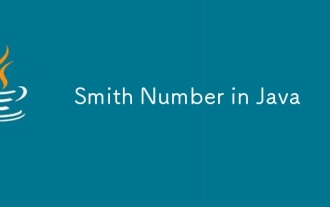
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
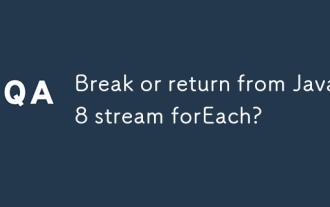
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
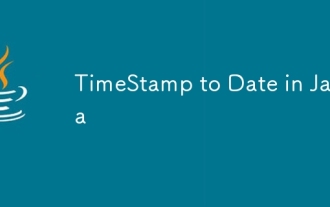
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
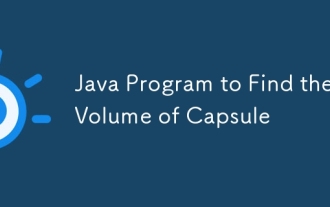
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
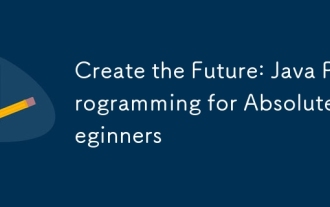
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
