


How to use thinkorm to implement related queries between data tables
How to use thinkorm to implement associated queries between data tables
Introduction:
During database development, we often encounter situations where we need to perform associated queries between multiple data tables. Using thinkorm, an excellent database ORM framework, you can easily implement associated queries of data tables and improve development efficiency. This article will introduce how to use thinkorm to implement related queries between data tables, and provide code examples to help readers better understand.
1. Basic concepts
Before performing associated queries, you first need to understand several basic concepts in thinkorm:
- Model: The model in thinkorm is a Class used to represent a data table.
- Relation: Relation refers to the connection relationship between one model and another model.
- Relation Type: Depending on the connection relationship, relationships can be divided into one-to-one (hasOne), one-to-many (hasMany), many-to-many (belongsToMany) and other types.
2. One-to-one correlation query
One-to-one correlation query refers to the correlation between one model and another model through foreign keys. The following is a sample code for using thinkorm to perform a one-to-one association query:
# 导入必要的模块 from thinkorm import Model, database # 创建数据库实例 db = database() # 定义模型 class User(Model): __table__ = 'users' __primary_key__ = 'id' class UserProfile(Model): __table__ = 'user_profiles' __primary_key__ = 'id' # 创建关联关系 User.hasOne({'profile': {'model': UserProfile, 'foreign_key': 'user_id'}}) UserProfile.belongsTo({'user': {'model': User, 'foreign_key': 'user_id'}}) # 查询 user = User.get(1) profile = user.profile print(user.name) # 输出用户姓名 print(profile.bio) # 输出用户简介
In the above sample code, a one-to-one association relationship is created by using the hasOne and belongsTo methods on the User model and UserProfile model. Among them, the parameter model represents the associated model, and foreign_key represents the foreign key field.
3. One-to-many association query
One-to-many association query refers to the association between one model and another model through foreign keys, and one model corresponds to multiple other models. The following is a sample code for using thinkorm to perform a one-to-many association query:
# 导入必要的模块 from thinkorm import Model, database # 创建数据库实例 db = database() # 定义模型 class User(Model): __table__ = 'users' __primary_key__ = 'id' class Post(Model): __table__ = 'posts' __primary_key__ = 'id' # 创建关联关系 User.hasMany({'posts': {'model': Post, 'foreign_key': 'user_id'}}) Post.belongsTo({'user': {'model': User, 'foreign_key': 'user_id'}}) # 查询 user = User.get(1) posts = user.posts for post in posts: print(post.title) # 输出文章标题
In the above sample code, a one-to-many association relationship is created by using the hasMany and belongsTo methods on the User model and Post model. All articles published by this user can be obtained through user.posts.
4. Many-to-many correlation query
Many-to-many correlation query refers to the correlation between one model and another model through an intermediate table, and one model can correspond to multiple other models. The following is a sample code for using thinkorm to perform a many-to-many association query:
# 导入必要的模块 from thinkorm import Model, database # 创建数据库实例 db = database() # 定义模型 class User(Model): __table__ = 'users' __primary_key__ = 'id' class Role(Model): __table__ = 'roles' __primary_key__ = 'id' Role.belongsToMany({'users': {'model': User, 'through': 'user_roles', 'foreignKey': 'role_id', 'otherKey': 'user_id'}}) User.belongsToMany({'roles': {'model': Role, 'through': 'user_roles', 'foreignKey': 'user_id', 'otherKey': 'role_id'}}) # 查询 user = User.get(1) roles = user.roles for role in roles: print(role.name) # 输出角色名称
In the above sample code, a many-to-many association relationship is created by using the belongsToMany method on the User model and Role model. The roles owned by the user can be obtained through user.roles.
Conclusion:
Using thinkorm to implement associated queries between data tables can enable more efficient database development. This article introduces the implementation methods of one-to-one, one-to-many, and many-to-many related queries through specific sample codes, and explains the differences between related types. Readers can flexibly use these methods to improve development efficiency according to their own needs and actual conditions.
The above is the detailed content of How to use thinkorm to implement related queries between data tables. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


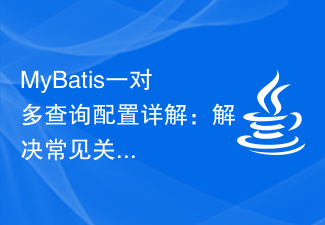
Detailed explanation of MyBatis one-to-many query configuration: To solve common associated query problems, specific code examples are required. In actual development work, we often encounter situations where we need to query a master entity object and its associated multiple slave entity objects. In MyBatis, one-to-many query is a common database association query. With correct configuration, the query, display and operation of associated objects can be easily realized. This article will introduce the configuration method of one-to-many query in MyBatis, and how to solve some common related query problems. It will also
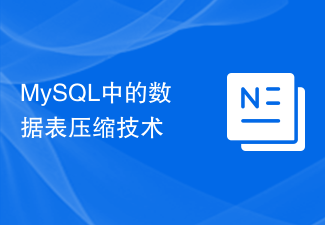
MySQL is a common relational database that is a core component of many websites and applications. As the amount of data becomes larger and larger, how to optimize the performance of MySQL becomes particularly important. One of the key areas is the compression of data tables. In this article we will introduce the data table compression technology in MySQL. Compressed tables and non-compressed tables There are two types of data tables in MySQL: compressed tables and non-compressed tables. Uncompressed tables are MySQL's default table type, which use fixed-length row format to store data. This means data
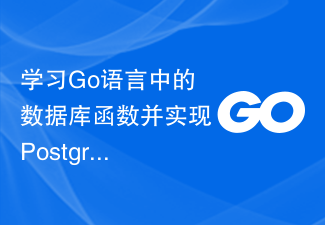
Learn the database functions in the Go language and implement the addition, deletion, modification, and query operations of PostgreSQL data. In modern software development, the database is an indispensable part. As a powerful programming language, Go language provides a wealth of database operation functions and toolkits, which can easily implement addition, deletion, modification and query operations of the database. This article will introduce how to learn database functions in Go language and use PostgreSQL database for actual operations. Step 1: Install the database driver in Go language for each database
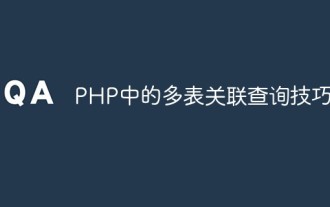
Multi-table related query skills in PHP Related queries are an important part of database queries, especially when you need to display data in multiple related database tables. In PHP applications, multi-table related queries are often used when using databases such as MySQL. The meaning of multi-table association is to compare data in one table with data in another or multiple tables, and connect those rows that meet the requirements in the result. When performing multi-table correlation queries, you need to consider the relationship between tables and use appropriate correlation methods. The following introduces several types of
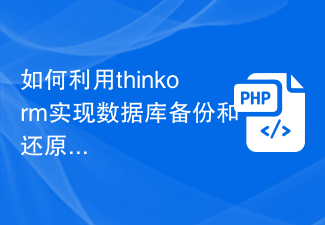
Title: Using ThinkORM to realize database backup and restoration Introduction: In the development process, database backup and restoration is a very important task. This article will introduce how to use the ThinkORM framework to implement database backup and restoration, and provide corresponding code examples. 1. Background introduction During the development process, we usually use databases to store and manage data. The principle of database backup and restore is to perform regular backups of the database so that the data can be quickly restored in the event of database problems or data loss. With the help of
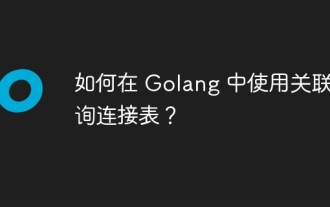
Correlation queries join tables to combine data from multiple tables through SQL queries. In Golang, use the sql.DB.Query() function to specify the query string and parameters. Different join types can be used, such as INNERJOIN, LEFTJOIN, RIGHTJOIN, and FULLOUTERJOIN, depending on the desired result set.
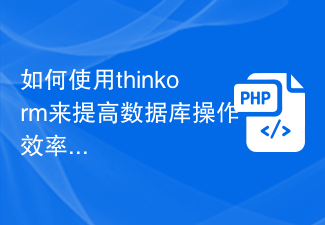
How to use thinkorm to improve database operation efficiency With the rapid development of the Internet, more and more applications require a large number of database operations. In this process, the efficiency of database operations becomes particularly important. In order to improve the efficiency of database operations, we can use thinkorm, a powerful ORM framework, to perform database operations. This article will introduce how to use thinkorm to improve the efficiency of database operations and illustrate it through code examples. 1. What is thinkormthi?
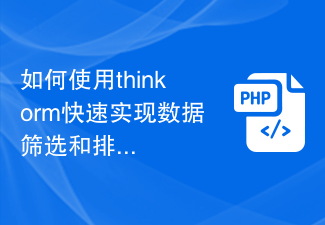
How to use ThinkORM to quickly implement data filtering and sorting Introduction: With the continuous increase of data, quickly finding the required data has become an important task in development. ThinkORM is a powerful and easy-to-use ORM (Object Relational Mapping) tool that can help us quickly filter and sort data. This article will introduce how to use ThinkORM to filter and sort data, and provide code examples. 1. Install ThinkORM: First, we need to install Thin
