


PHP form validation tips: How to use the filter_input function to verify user input
PHP form validation skills: How to use the filter_input function to verify user input
Introduction:
When developing web applications, forms are an important tool for interacting with users. Correctly validating user input is one of the key steps to ensure data integrity and security. PHP provides the filter_input function, which can easily verify and filter user input. This article will introduce how to use the filter_input function to verify user input and provide relevant code examples.
1. Basic usage of filter_input function
The filter_input function is a very powerful function used to filter and verify user input. It accepts three parameters: input type (input source), input name and filter type. Here is a basic example:
$input = filter_input(INPUT_POST, 'email', FILTER_VALIDATE_EMAIL); if ($input) { echo '有效的邮箱地址。'; } else { echo '请输入一个有效的邮箱地址。'; }
In the above example, we used the filter_input function to validate the email field submitted by the user through the POST method. The FILTER_VALIDATE_EMAIL filter type is used to verify the validity of email addresses. If the email address is valid, output "valid email address", otherwise output "please enter a valid email address".
2. Commonly used filter types
The filter_input function supports multiple filter types for validating different types of user input. Here are a few commonly used filter types:
- FILTER_VALIDATE_EMAIL: Verify the validity of an email address.
$email = filter_input(INPUT_POST, 'email', FILTER_VALIDATE_EMAIL); if (!$email) { echo '请输入一个有效的邮箱地址。'; }
- FILTER_VALIDATE_INT: Verify the validity of the integer.
$age = filter_input(INPUT_POST, 'age', FILTER_VALIDATE_INT); if (!$age) { echo '请输入一个有效的年龄。'; }
- FILTER_VALIDATE_URL: Verify the validity of the URL.
$url = filter_input(INPUT_POST, 'url', FILTER_VALIDATE_URL); if (!$url) { echo '请输入一个有效的URL地址。'; }
- FILTER_SANITIZE_STRING: Remove tags and special characters from user input.
$name = filter_input(INPUT_POST, 'name', FILTER_SANITIZE_STRING); if (!$name) { echo '请输入一个有效的姓名。'; }
3. Custom filter function
In addition to the built-in filter types, we can also customize filter functions to verify user input. The custom filter function should accept one parameter (the value to be verified) and return a verification result (true or false). The following is an example of a custom filter function:
function validate_username($username) { // 用户名必须以字母开头,只能包含字母、数字和下划线 return preg_match('/^[a-zA-Z][a-zA-Z0-9_]*$/', $username); } $username = filter_input(INPUT_POST, 'username', FILTER_CALLBACK, array('options' => 'validate_username')); if (!$username) { echo '请输入一个有效的用户名。'; }
In the above example, we define a validate_username function to verify the validity of the username. This function checks if the username starts with a letter and contains only letters, numbers, and underscores by using a regular expression. We then call that custom function using the FILTER_CALLBACK filter type and an array containing options to validate the user input.
Summary:
In this article, we introduced how to use PHP's filter_input function to validate user input. We learned the basic usage of the filter_input function and provided examples of commonly used filter types and custom filter functions. Properly validating user input is critical to ensuring data integrity and security. Hopefully these tips will help you validate user input effectively and improve the quality and user experience of your web applications.
Reference materials:
- PHP filter_input function documentation: https://www.php.net/filter_input
- PHP filter type documentation: https:// www.php.net/manual/en/filter.filters.php
The above is the detailed content of PHP form validation tips: How to use the filter_input function to verify user input. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


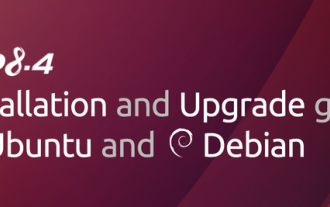
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
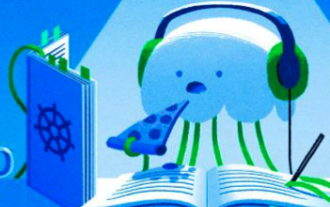
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
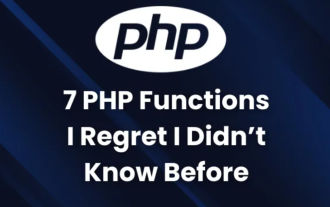
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
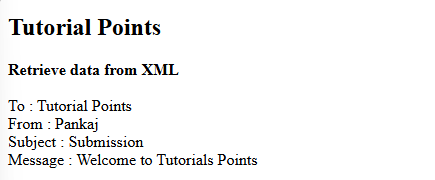
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
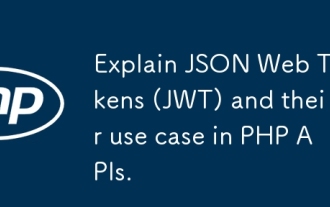
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
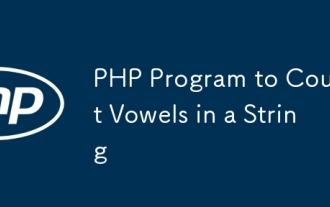
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
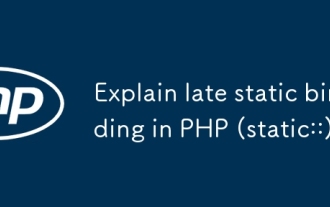
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
