


Application of Redis key-value pair operations in Java development: how to quickly access data
Application of Redis key-value pair operations in Java development: How to quickly access data
In Java development, data access operations are a very important task. How to access data quickly and efficiently is a key issue that developers are concerned about. As a high-performance in-memory database, Redis has the characteristics of fast read and write operations, so it is widely used in data caching and storage implementation in Java development.
Redis is an in-memory database that supports key-value pair access. It stores data in memory, so data can be read and written very quickly. Compared with traditional relational databases, Redis has higher performance and lower latency in data access. In Java development, you can implement fast access operations of key-value pairs by using the Jedis library provided by Redis.
First, we need to introduce the Jedis library into the project. The Jedis library can be introduced by adding the following dependency in the pom.xml file of the Maven project:
<dependency> <groupId>redis.clients</groupId> <artifactId>jedis</artifactId> <version>3.7.0</version> </dependency>
Then, we can demonstrate the usage of Redis key-value pair operations through the following code example:
import redis.clients.jedis.Jedis; public class RedisExample { public static void main(String[] args) { // 创建一个Jedis对象,连接Redis服务器 Jedis jedis = new Jedis("localhost", 6379); // 设置一个键值对 jedis.set("name", "张三"); // 获取键对应的值 String name = jedis.get("name"); System.out.println("姓名:" + name); // 删除一个键值对 jedis.del("name"); // 关闭连接 jedis.close(); } }
In the above code, we first create a Jedis object and specify the address of the Redis server (usually localhost if local) and port number (default is 6379). Then through jedis.set("name", "Zhang San")
a key-value pair is set, the key is "name" and the value is "Zhang San". Then use jedis.get("name")
to get the value corresponding to the key, and use System.out.println
to output the result. Finally, the key-value pair is deleted through jedis.del("name")
. Finally, we close the connection to the Redis server and release the resources.
In addition to basic access operations, Redis can also support more operations, such as setting the expiration time of keys, incrementally updating key values, etc. The following are some common methods for Redis key-value pair operations:
-
jedis.set(key, value)
: Set a key-value pair. -
jedis.get(key)
: Get the value corresponding to the key. -
jedis.del(key)
: Delete a key-value pair. -
jedis.expire(key, seconds)
: Set the expiration time of the key, in seconds. -
jedis.incr(key)
: Add 1 to the value corresponding to the key. -
jedis.decr(key)
: Decrease the value corresponding to the key by 1.
By learning and mastering the usage of Redis key-value pair operations, we can achieve fast and efficient data access in Java development. Whether it is data caching or data storage, Redis can meet our needs through its fast performance and rich data operation methods. Therefore, it is a wise choice to make more use of Redis in daily development to improve the performance and response speed of the program.
In short, Redis key-value pair operations have the ability to quickly access data in Java development. By flexibly using the methods it provides, developers can efficiently perform data access operations. Through in-depth research and practice, we can better master the usage skills of Redis, thereby achieving fast and efficient data access and processing in Java development.
The above is the detailed content of Application of Redis key-value pair operations in Java development: how to quickly access data. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
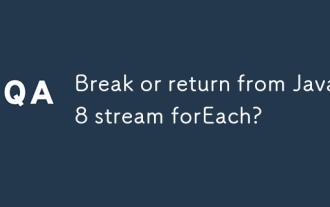
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
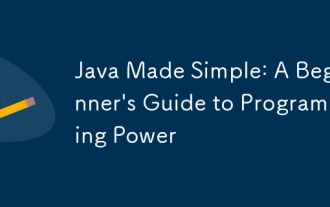
Java Made Simple: A Beginner's Guide to Programming Power Introduction Java is a powerful programming language used in everything from mobile applications to enterprise-level systems. For beginners, Java's syntax is simple and easy to understand, making it an ideal choice for learning programming. Basic Syntax Java uses a class-based object-oriented programming paradigm. Classes are templates that organize related data and behavior together. Here is a simple Java class example: publicclassPerson{privateStringname;privateintage;
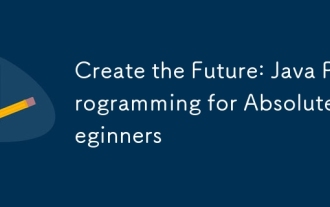
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
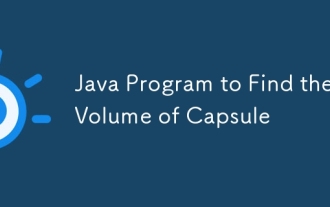
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
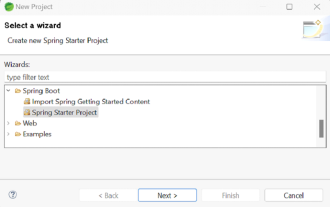
Spring Boot simplifies the creation of robust, scalable, and production-ready Java applications, revolutionizing Java development. Its "convention over configuration" approach, inherent to the Spring ecosystem, minimizes manual setup, allo
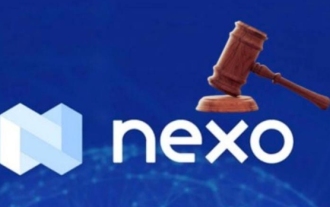
Nexo Exchange: Swiss cryptocurrency lending platform In-depth analysis Nexo is a platform that provides cryptocurrency lending services, supporting the mortgage and lending of more than 40 crypto assets, fiat currencies and stablecoins. It dominates the European and American markets and is committed to improving the efficiency, security and compliance of the platform. Many investors want to know where the Nexo exchange is registered, and the answer is: Switzerland. Nexo was founded in 2018 by Swiss fintech company Credissimo. Nexo Exchange Geographical Location and Regulation: Nexo is headquartered in Zug, Switzerland, a well-known cryptocurrency-friendly region. The platform actively cooperates with the supervision of various governments and has been in the US Financial Crime Law Enforcement Network (FinCEN) and Canadian Finance
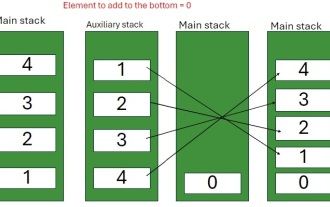
A stack is a data structure that follows the LIFO (Last In, First Out) principle. In other words, The last element we add to a stack is the first one to be removed. When we add (or push) elements to a stack, they are placed on top; i.e. above all the
