


Optional class in Java 8: How to filter possibly null values using filter() method
Optional class in Java 8: How to filter possibly empty values using the filter() method
In Java 8, the Optional class is a very useful tool that allows us to better handle possible A null value avoids the occurrence of NullPointerException. The Optional class provides many methods to manipulate potential null values, one of the important methods is filter().
The function of the filter() method is that if the value of the Optional object exists and meets the given conditions, the Optional object itself is returned; if the value does not exist or the conditions are not met, an empty value is returned. Optional object.
The following code example demonstrates how to use the filter() method to filter potentially empty values:
import java.util.Optional; public class OptionalFilterExample { public static void main(String[] args) { String name = "John Doe"; Optional<String> nameOptional = Optional.ofNullable(name); // 使用filter()方法过滤值为空的Optional对象 Optional<String> filteredOptional = nameOptional.filter(n -> n.length() > 5); if (filteredOptional.isPresent()) { System.out.println("Name is longer than 5 characters"); } else { System.out.println("Name is either null or shorter than 5 characters"); } } }
In the above example, we first create a non-empty Optional object nameOptional, Its value is "John Doe". Then, we use the filter() method, passing the condition n -> n.length() > 5
to it. This condition checks if the length of the string is greater than 5. If the condition is met, the filter() method returns an Optional object containing the same value; otherwise, it returns an empty Optional object.
Finally, we use the isPresent() method to check whether the filtered Optional object contains a value, and output the corresponding information based on the result.
In actual development, we often need to filter out values that may be empty. Using the filter() method, we can accomplish this task concisely. Here is another example that demonstrates how to filter out integers greater than 10 in a list:
import java.util.ArrayList; import java.util.List; import java.util.Optional; public class OptionalFilterListExample { public static void main(String[] args) { List<Integer> numbers = new ArrayList<>(); numbers.add(5); numbers.add(15); numbers.add(8); numbers.add(20); List<Integer> filteredNumbers = new ArrayList<>(); for (Integer number : numbers) { Optional<Integer> optionalNumber = Optional.ofNullable(number); optionalNumber.filter(n -> n > 10).ifPresent(filteredNumbers::add); } System.out.println(filteredNumbers); } }
In the above example, we first created an integer list numbers, which contains some numbers. We then use a for-each loop to iterate over each element in the list and wrap them into Optional objects.
Next, we use the filter() method to filter out numbers greater than 10, and use the ifPresent() method to add the filtered numbers to the filteredNumbers list.
Finally, we output the filteredNumbers list, which contains all filtered numbers.
By using the filter() method of the Optional class, we can filter possible null values more concisely, avoiding cumbersome null checks and situations that may lead to NullPointerException. This makes our code more robust and readable. It is recommended to make full use of various methods of the Optional class in development to improve code quality and development efficiency.
The above is the detailed content of Optional class in Java 8: How to filter possibly null values using filter() method. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


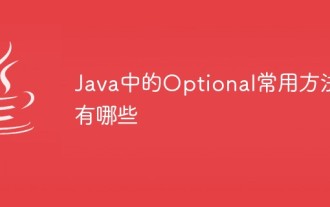
Preface Optional in Java is a container object, which can contain a non-null value or be empty. Its main purpose is to avoid null pointer exceptions when writing code. The complete usage of Optional in java8 is as follows: 1. Create an Optional object. You can create an Optional object containing a non-null value through the of() method, for example: Optionaloptional=Optional.of("value"); It can also be created through the ofNullable() method. An Optional object containing a possibly null value, for example: Optionaloptional=Optiona
![How to solve the '[Vue warn]: Failed to resolve filter' error](https://img.php.cn/upload/article/000/887/227/169243040583797.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
Methods to solve the "[Vuewarn]:Failedtoresolvefilter" error During the development process using Vue, we sometimes encounter an error message: "[Vuewarn]:Failedtoresolvefilter". This error message usually occurs when we use an undefined filter in the template. This article explains how to resolve this error and gives corresponding code examples. When we are in Vue
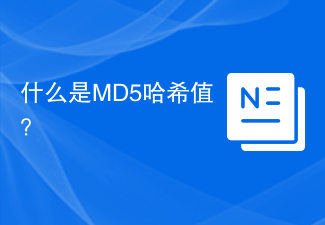
What is the MD5 value? In computer science, MD5 (MessageDigestAlgorithm5) is a commonly used hash function used to digest or encrypt messages. It produces a fixed-length 128-bit binary number, usually represented in 32-bit hexadecimal. The MD5 algorithm was designed by Ronald Rivest in 1991. Although the MD5 algorithm is considered no longer secure in the field of cryptography, it is still widely used in data integrity verification and file verification.
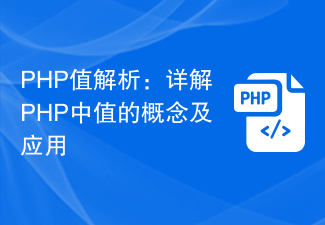
PHP value analysis: Detailed explanation of the concept and application of value in PHP In PHP programming, value is a very basic and important concept. In this article, we will take a deep dive into the concept of values in PHP and its application in real-world programming. We will analyze in detail basic value types, variables, arrays, objects and constants, etc., and provide specific code examples to help readers better understand and use values in PHP. Basic value types In PHP, the most common basic value types include integer, floating point, string, Boolean and null. These basic

How to use the Optional function to handle null values in Java In Java programming, we often encounter situations where null values are handled. Null pointer exception is a common error. In order to avoid this situation, Java8 introduced the Optional class to handle null value situations. The Optional class is a container class that can contain a non-empty value or no value. Using the Optional class, we can handle null value situations more gracefully and avoid null pointer exceptions. under
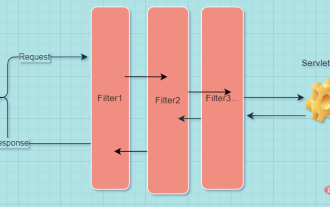
1. Filter First look at the location of the filter of the web server. Filter is a chain connected before and after. After the previous processing is completed, it is passed to the next filter for processing. 1.1Filter interface definition publicinterfaceFilter{//Initialization method, only executed once in the entire life cycle. //Filtering services cannot be provided until the init method is successfully executed (failure such as throwing an exception, etc.). //The parameter FilterConfig is used to obtain the initialization parameter publicvoidinit(FilterConfigfilterConfig)throwsServletException;//
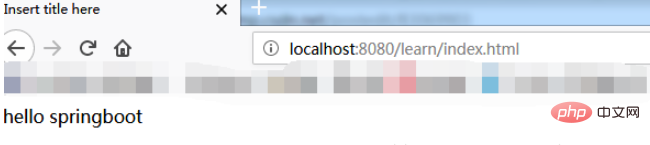
First define a Filter for unified access URL interception. The code is as follows: publicclassUrlFilterimplementsFilter{privateLoggerlog=LoggerFactory.getLogger(UrlFilter.class);@OverridepublicvoiddoFilter(ServletRequestrequest,ServletResponseresponse,FilterChainchain)throwsIOException,ServletException{H
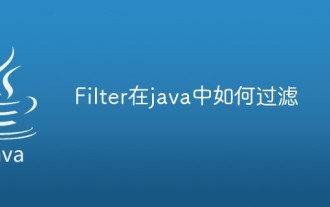
Note 1. If the Lambda parameter generates a true value, the filter (Lambda that can generate a boolean result) will generate an element; 2. When false is generated, this element will no longer be used. Example to create a List collection: ListstringCollection=newArrayList();stringCollection.add("ddd2");stringCollection.add("aaa2");stringCollection.add("bbb1");stringC
