


PHP and PDO: How to insert and read image data into a database
PHP and PDO: How to insert and read image data into the database
Introduction:
With the development of web applications, image processing and storage are becoming more and more important. In PHP, by using PDO (PHP Data Objects) extension, we can easily insert and read image data into the database. This article will introduce how to use PDO to insert and read image data in the database, and provide corresponding code examples.
1. Insert image data into the database
- Create database table
First, we need to create a table to store image data. The following is an example of creating a table:
CREATE TABLE images ( id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(255), image LONGBLOB );
- Connecting to the database
In PHP, we first need to create a connection to the database through PDO. Here is the sample code:
$dsn = "mysql:host=localhost;dbname=test"; $username = "root"; $password = "password"; try { $pdo = new PDO($dsn, $username, $password); } catch(PDOException $e) { die("Connection failed: " . $e->getMessage()); }
- Insert image data
Next, we will use PHP's file upload function to upload the image file to the server and insert it into the database. The following is the sample code:
$imageName = $_FILES['image']['name']; $imageData = file_get_contents($_FILES['image']['tmp_name']); $imageType = $_FILES['image']['type']; $sql = "INSERT INTO images (name, image) VALUES (:name, :image)"; $stmt = $pdo->prepare($sql); $stmt->bindParam(':name', $imageName); $stmt->bindParam(':image', $imageData, PDO::PARAM_LOB); $stmt->execute();
Code explanation:
- First, we get the name, data and type of the uploaded image file.
- Then, we create an INSERT statement using a parameterized query. :name and :image are placeholders, which will be replaced with actual values later.
- Next, we use PDO’s prepare method to prepare the query.
- Use the bindParam method to bind parameters to placeholders. The first parameter is the name of the placeholder, the second parameter is the variable to be bound, and the third parameter specifies the data type as LOB (Large Object).
- Finally, we use the execute method to execute the query and insert the image data into the database.
2. Reading image data from the database
- Reading image data
Use PDO to read image data from the database and read other types of data similar. The following is the sample code:
$id = 1; // 图像在数据库中的ID $sql = "SELECT image FROM images WHERE id = :id"; $stmt = $pdo->prepare($sql); $stmt->bindParam(':id', $id); $stmt->execute(); $imageData = $stmt->fetchColumn();
Code explanation:
- First, we prepare the SELECT statement and use the placeholder:id to represent the image data to be obtained from the database ID.
- Then, use PDO’s prepare method again to prepare the query.
- Use the bindParam method to bind parameters to placeholders.
- Finally, use the execute method to execute the query and the fetchColumn method to obtain the image data.
- Display image
Finally, we can use the following code to display the image data on the web page:
header("Content-type: image/jpeg"); echo $imageData;
Code explanation:
- Use the header function to set the Content-type header information and specify the type of image.
- Then, the image data is output in the form of an image file and displayed on the web page.
Conclusion:
By using PHP and PDO, we can easily insert image data into the database, read and display image data from the database. This simplifies the processing and storage of images, improving the performance and efficiency of web applications.
The above is the introduction and sample code on how to use PHP and PDO to insert and read image data into the database. Hopefully this article will help you understand and learn about image data processing and storage.
Reference:
- [PHP: PDO](https://www.php.net/manual/en/book.pdo.php)
- [PHP: image functions](https://www.php.net/manual/en/book.image.php)
The above is the detailed content of PHP and PDO: How to insert and read image data into a database. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
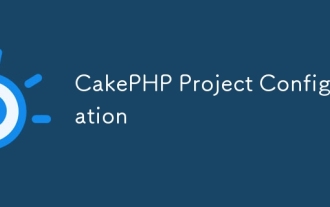
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
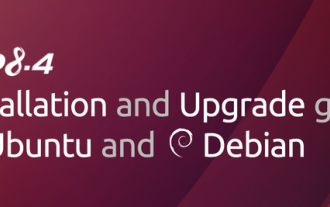
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
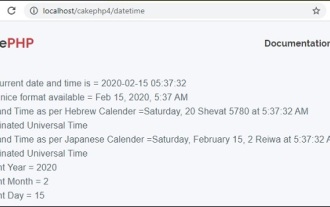
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
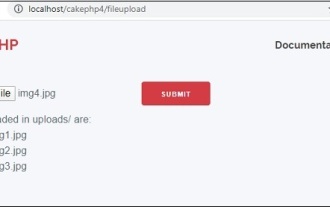
To work on file upload we are going to use the form helper. Here, is an example for file upload.
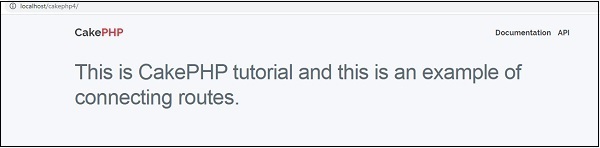
In this chapter, we are going to learn the following topics related to routing ?
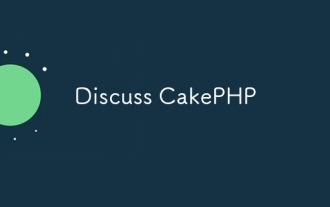
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
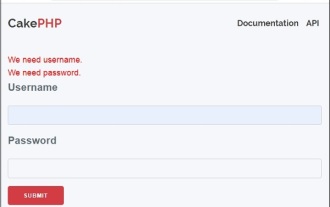
Validator can be created by adding the following two lines in the controller.
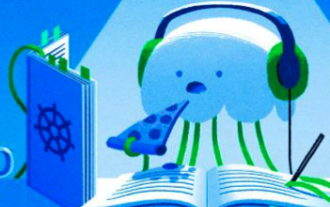
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
