


How to use PHP for server-side push and real-time communication
How to use PHP for server-side push and real-time communication
With the continuous development of technology and the popularity of the Internet, real-time communication is becoming more and more important in Web applications. Server-side push and real-time communication enable developers to send real-time updated data to and interact with clients without the client actively requesting data from the server.
In PHP development, we can use some technologies to achieve server-side push and real-time communication, such as: WebSocket, Long Polling, Server-Sent Events, etc. This article will focus on using Server-Sent Events (SSE) to implement server-side push and real-time communication.
Server-Sent Events (SSE) is a one-way communication technology between the browser and the server, which can push data from the server to the client in real time. SSE technology relies on the HTTP protocol and does not require the use of WebSocket.
First, we need to establish a push service on the PHP server. The following is a simple PHP code example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
|
In the above example, first we set the response header to tell the browser that the SSE event stream is returned. Then, in an infinite loop, we get the data from the database or other data source, convert the data to JSON format, and send the data to the client using the echo
statement. Note that after each data is sent, we call the ob_flush()
and flush()
functions to ensure that the data is sent to the client. Finally, we use the sleep()
function to let the server sleep for a period of time to control the push speed.
Next, use JavaScript on the client to receive the data pushed by the server. Here is a simple HTML and JavaScript code example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
|
In the above example, we use the EventSource
object to establish a connection to the server and specify the URL to receive data. Then, we receive the pushed data from the server by listening to the onmessage
event, and display the data on the page.
Of course, this is just a simple example. In actual development, we can expand the code according to needs and handle more complex logic.
To sum up, using PHP for server-side push and real-time communication can help us build richer and more real-time web applications. Through Server-Sent Events technology, we can easily push real-time updated data to the client and interact with the client at the same time. I hope this article can be helpful to you, thank you for reading!
The above is the detailed content of How to use PHP for server-side push and real-time communication. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
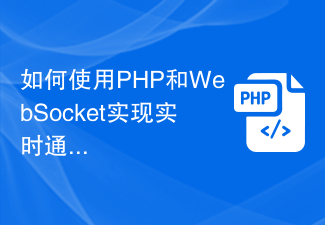
With the continuous development of Internet technology, real-time communication has become an indispensable part of daily life. Efficient, low-latency real-time communication can be achieved using WebSockets technology, and PHP, as one of the most widely used development languages in the Internet field, also provides corresponding WebSocket support. This article will introduce how to use PHP and WebSocket to achieve real-time communication, and provide specific code examples. 1. What is WebSocket? WebSocket is a single
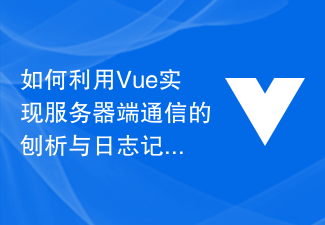
How to use Vue to implement parsing and logging of server-side communication In modern web applications, server-side communication is crucial for processing real-time data and interactivity. Vue is a popular JavaScript framework that provides a simple and flexible way to build user interfaces and process data. This article will explore how to use Vue to implement server-side communication and perform detailed analysis and logging. A common way to implement server-side communication is to use WebSockets. WebSo
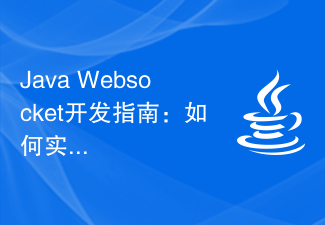
Java Websocket Development Guide: How to implement real-time communication between the client and the server, specific code examples are required. With the continuous development of web applications, real-time communication has become an indispensable part of the project. In the traditional HTTP protocol, the client sends a request to the server, and the data can only be obtained after receiving the response. This causes the client to continuously poll the server to obtain the latest data, which will lead to performance and efficiency problems. And WebSocket is for understanding
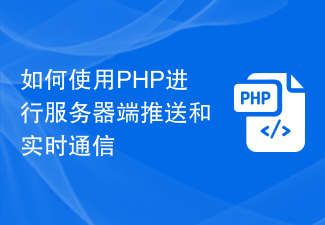
How to use PHP for server-side push and real-time communication With the continuous development of technology and the popularity of the Internet, real-time communication is becoming more and more important in web applications. Server-side push and real-time communication enable developers to send real-time updated data to and interact with clients without the client actively requesting data from the server. In PHP development, we can use some technologies to achieve server-side push and real-time communication, such as: WebSocket, LongPolling, Serve
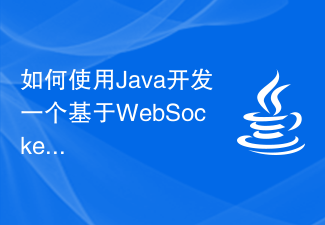
How to use Java to develop a real-time communication application based on WebSocket. In modern Web applications, real-time communication has become a necessary function. WebSocket technology plays an important role in this regard. WebSocket is a full-duplex communication protocol that allows real-time two-way communication between the server and client. This article will introduce how to use Java to develop a real-time communication application based on WebSocket, and provide some specific code examples. Preparations are beginning

Building a real-time chat room using Redis and C#: How to implement instant messaging Introduction: In today's Internet era, instant messaging has become an increasingly important way of communication. Whether it’s social media, online gaming or online customer service, live chat rooms play an important role. This article will introduce how to use Redis and C# to build a simple real-time chat room and understand the messaging mechanism based on the publish/subscribe model. 1. Preparation Before starting, we need to prepare some tools and environment: Visual Studio
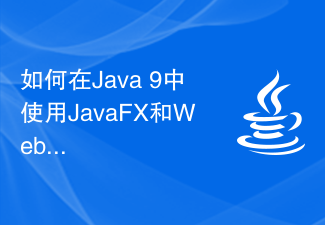
How to use JavaFX and WebSocket to implement a graphical interface for real-time communication in Java9 Introduction: With the development of the Internet, the need for real-time communication is becoming more and more common. In Java9, we can use JavaFX and WebSocket technology to implement real-time communication applications with graphical interfaces. This article will introduce how to use JavaFX and WebSocket technology to implement a graphical interface for real-time communication in Java9, and attach corresponding code examples. Part One: Ja
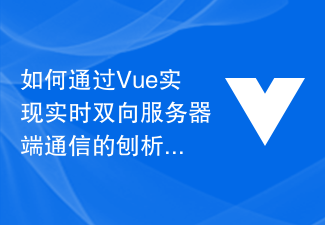
Analysis of how to implement real-time two-way server-side communication through Vue Introduction: In modern web applications, real-time two-way server-side communication is becoming more and more important. It can realize functions such as real-time data updates, real-time chat and collaborative editing. Vue is a popular front-end framework that provides a concise way to build user interfaces. This article will introduce how to use Vue and Socket.io to achieve real-time two-way server-side communication. 1. Understand Socket.ioSocket.io is a web browser-oriented
