How to delete an element from js array
Methods to delete an element from a js array: 1. Use the splice() method; 2. Use the pop() method; 3. Use the shift() method; 4. Use the delete keyword.
The array in JavaScript is a very commonly used data structure that can store multiple elements. Sometimes, when processing an array, we may need to delete a specific element. This article will introduce you to several ways to delete array elements in JavaScript.
1. Use the splice() method:
The splice() method is the most commonly used method in JavaScript to delete array elements. It deletes one or more elements at a specified position from an array and returns the deleted elements. The splice() method requires two parameters: the index position of the element to be deleted and the number of elements to be deleted. Here is an example:
let fruits = ['apple', 'banana', 'orange', 'grape']; fruits.splice(1, 2); console.log(fruits); // 输出:['apple', 'grape']
In the above code, we deleted 2 elements ('banana' and 'orange') starting from index position 1.
2. Use the pop() method:
The pop() method will delete the last element of the array and return the element. This method is suitable for deleting arrays with stack characteristics. Here is an example:
let fruits = ['apple', 'banana', 'orange', 'grape']; fruits.pop(); console.log(fruits); // 输出:['apple', 'banana', 'orange']
In the above code, we delete the last element ('grape') of the array.
3. Use the shift() method:
The shift() method is similar to the pop() method, but it deletes the first element of the array, and Return the element. This method is suitable for deleting arrays with queue characteristics. Here is an example:
let fruits = ['apple', 'banana', 'orange', 'grape']; fruits.shift(); console.log(fruits); // 输出:['banana', 'orange', 'grape']
In the above code, we delete the first element ('apple') of the array.
4. Use the delete keyword:
The delete keyword is an operator in JavaScript, which can delete the element at a specified position in the array. However, when you use the delete keyword to delete array elements, the length of the array will not change, and the value of the deleted element will become undefined. Here is an example:
let fruits = ['apple', 'banana', 'orange', 'grape']; delete fruits[2]; console.log(fruits); // 输出:['apple', 'banana', undefined, 'grape']
In the above code, we delete the element ('orange') at index position 2 in the array and replace it with undefined.
It should be noted that when using the delete keyword to delete an array element, the deleted element is only marked as deleted and will not actually be deleted from the memory. Therefore, in some cases, after deleting the element, Causes some strange behavior in arrays, and it is generally not recommended to use delete to delete array elements.
The above are some common methods for deleting array elements in JavaScript. You can choose the appropriate method to delete array elements based on your specific needs. Hope this article is helpful to you!
The above is the detailed content of How to delete an element from js array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


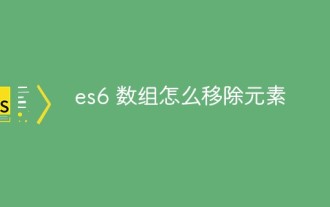
Method: 1. Use shift() to delete the first element, the syntax is "array.shift()"; 2. Use pop() to delete the last element, the syntax is "array.pop()"; 3. Use splice() to delete Elements at any position, the syntax is "array.splice(position, number)"; 4. Use length to delete the last N elements, the syntax is "array.length=original array length-N"; 5. Directly assign the empty array "[ ]" to clear the element; 6. Use delete to delete an element at the specified subscript.
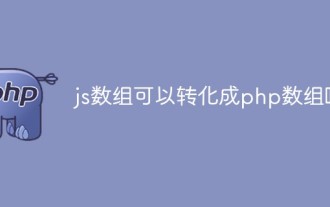
The js array can be converted into a php array. The operation method is: 1. Create a php sample file; 2. Use the syntax "JSON.stringify()" to convert the js array into a string in JSON format; 3. Use the syntax "json_decode()" "Convert the JSON format string to a PHP array. The parameter true is added here, which means that the JSON format string is converted into a PHP associative array.
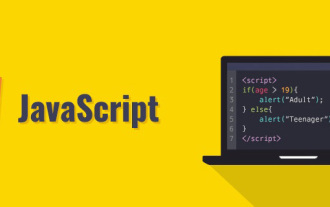
3 conversion methods: 1. Use split() to split a given string into a string array, the syntax is "str.split (separator, maximum length of array)"; 2. Use the expansion operator "... ", iterable string object, convert it into a character array, the syntax "[...str]"; 3. Use Array.from() to convert the string into an array, the syntax "Array.from(str) ".
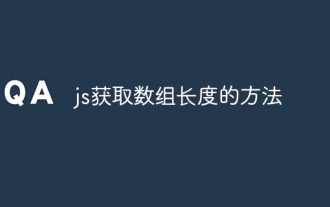
Getting the length of an array in JS is very simple. Each array has a length property, which returns the maximum length of the array, that is, its value is equal to the maximum subscript value plus 1. Since numeric subscripts must be less than 2^32-1, the maximum value of the length attribute is equal to 2^32-1. The following code defines an empty array, and then assigns a value to the element with the index equal to 100, then the length property returns 101. Therefore, the length attribute cannot reflect the actual number of array elements.
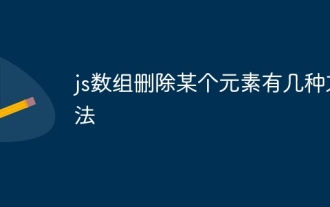
There are 4 ways to delete an element from a js array, namely: 1. Use splice; 2. Use filter; 3. Use the pop method and shift; 4. Use the delete keyword.
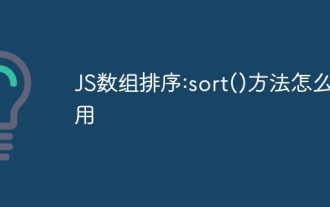
JavaScript's Array.prototype.sort() method is used to sort the elements of an array. This method sorts in place, that is, it modifies the original array rather than returning a new sorted array. By default, the sort() method sorts strings according to their Unicode code point values. This means that it is used primarily for sorting strings and numbers, rather than for sorting objects or other complex data types.
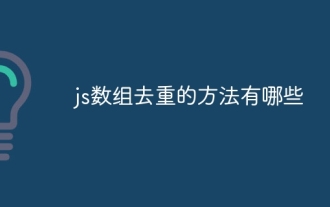
Methods to deduplicate js arrays include using Set, using indexOf, using includes, using filter and using reduce. 1. Use Set, which is characterized by the fact that the elements in the set will not be repeated; 2. Use indexOf to return the first index position of the specified element in the array; 3. Use includes to determine whether an element already exists in the array. 4. Use filter to filter elements; 5. Use reduce to compress elements in an array, etc.
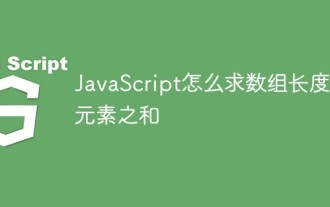
In JavaScript, you can use the length attribute to get the length of the array, the syntax is "array object.length"; you can use the reduce() or reduceRight() function to find the sum of elements, the syntax is "arr.reduce(function f(pre,curr){ return pre+cur})" or "arr.reduceRight(function f(pre,curr){return pre+cur})".