


PHP 5.6 Function Analysis: How to use array_search function to find a specific value in an array
PHP 5.6 Function Analysis: How to use the array_search function to find a specific value in an array
In PHP programming, arrays are a very common and important data structure. To manipulate and process data in arrays, PHP provides many built-in functions. One of them is the array_search function, which helps us find a specific value in an array.
The function of array_search function is to search for a given value in an array and return the key name of the value. If multiple matches are found, it will only return the first matching key name. If no match is found, false is returned.
The following is the syntax and usage of the array_search function:
mixed array_search ( mixed $needle , array $haystack [, bool $strict = false ] )
- $needle: the value to be found
- $haystack: the array to be found
- $strict: Optional parameter, if set to true, strict mode will be used for comparison, the default is false
Next, let us demonstrate the use of the array_search function through some code examples.
Example 1: Find string value
$fruits = array("apple", "orange", "banana", "mango"); $index = array_search("banana", $fruits); if ($index !== false) { echo "找到了,键名为:" . $index; } else { echo "未找到该值"; }
In the above code, first we define an array $fruits containing multiple fruit names. Then, we call the array_search function to find the key name of "banana". If the value is found, we print the key name. Otherwise, the output is that the value was not found.
Example 2: Find numeric values
$numbers = array(1, 5, 3, 8, 2, 7); $index = array_search(5, $numbers); if ($index !== false) { echo "找到了,键名为:" . $index; } else { echo "未找到该值"; }
In the above code, we define an array $numbers that contains multiple numbers. Then, we use the array_search function to find the key name of the number 5. If the value is found, we print the key name. Otherwise, the output is that the value was not found.
Example 3: Using strict mode
$numbers = array(1, 5, "3", 8, 2, 7); $index = array_search(3, $numbers, true); if ($index !== false) { echo "找到了,键名为:" . $index; } else { echo "未找到该值"; }
In the above code, we define an array $numbers containing numbers and strings. Then, we use the array_search function to find the key name of the number 3 and use strict comparison mode for matching. Since the data type of the number 3 is inconsistent with the string "3", the value is not found in the final output.
Through the above examples, we can see the usage and function of the array_search function. It is a very convenient function that can be used to quickly find a specific value in an array.
It should be noted that the array_search function will only return the key name of the first matching item. If we need to find all matching key names in the array, we need to use other methods to achieve it.
To summarize, PHP's array_search function is a very useful function that can quickly find a specific value in an array and return its key name. By using this function properly, we can process and operate the data in the array more efficiently.
The above is the detailed content of PHP 5.6 Function Analysis: How to use array_search function to find a specific value in an array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
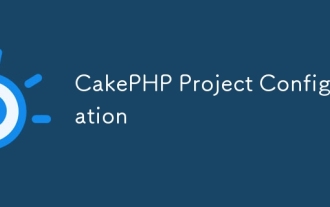
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
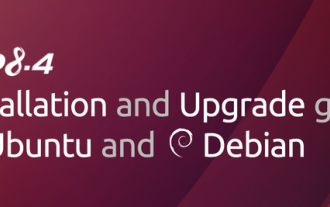
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
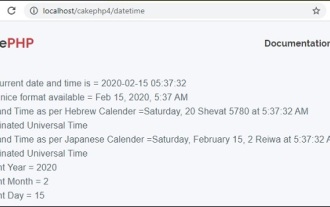
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
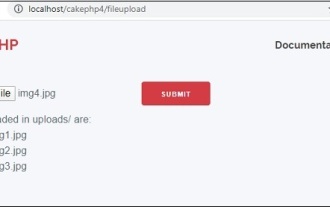
To work on file upload we are going to use the form helper. Here, is an example for file upload.
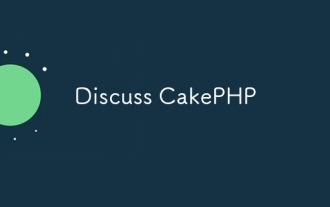
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
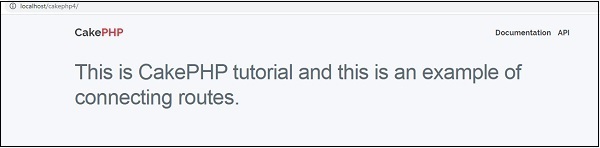
In this chapter, we are going to learn the following topics related to routing ?
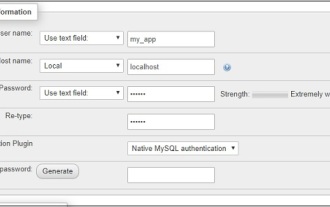
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
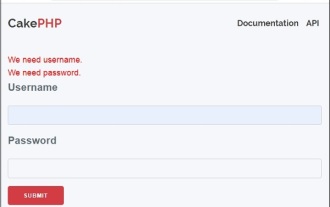
Validator can be created by adding the following two lines in the controller.
