How to use MySQL's join query to optimize complex query operations
How to use MySQL's join query to optimize complex query operations
In application development, we often face the need to query related data in multiple tables, and the join query in MySQL solves this type of problem. Important tool. Join queries can provide the results of complex queries by joining multiple tables, effectively solving the problems of data redundancy and duplication.
However, join queries can cause performance issues because it requires calculations for each joined row combination. When processing large amounts of data, join queries can cause queries to slow down or consume large amounts of system resources. This article introduces some methods of optimizing join queries and provides corresponding code examples.
1. Use INNER JOIN to optimize connection queries
INNER JOIN is the most commonly used type of connection query. It returns matching rows in two tables. When using INNER JOIN, you can optimize query performance through the following two methods.
- Ensure that the index of the table is created correctly
For the join field, an index should be created for it, which will speed up the query and reduce the consumption of system resources. The following is an example:
CREATE INDEX index_name ON table_name (column_name);
- Accurately select the fields to be queried
In INNER JOIN, the query results will include all fields in the connected table. However, sometimes we only need some fields, and we can use the SELECT clause to accurately select the required fields, reducing the overhead of data transmission and processing. The following is an example:
SELECT table1.column1, table1.column2, table2.column3 FROM table1 INNER JOIN table2 ON table1.id = table2.id
2. Use LEFT JOIN/RIGHT JOIN to optimize connection queries
In addition to INNER JOIN, MySQL also supports LEFT JOIN and RIGHT JOIN. These two join types are typically used to query records that exist in one table but not in another table.
When using LEFT JOIN/RIGHT JOIN, you can optimize it through the following methods.
- Simplify the query conditions
When using LEFT JOIN/RIGHT JOIN, the query conditions should be simplified as much as possible. You can consider using EXISTS subquery or other methods instead. Simplifying query conditions can improve query performance and reduce system resource consumption.
- Use the appropriate connection type
When using LEFT JOIN/RIGHT JOIN, you should choose the appropriate connection type according to the query requirements. LEFT JOIN will return all records in the left table, even if there are no matching records in the right table; while RIGHT JOIN will return all records in the right table, even if there are no matching records in the left table.
3. Use subqueries to optimize join queries
In some cases, join queries are very complex and difficult to optimize. At this time, you can consider using subqueries instead of join queries.
Subquery refers to a query statement nested in other query statements. By using subqueries, you can improve query performance by splitting a complex join query into multiple simpler queries. Here is an example:
SELECT column1, column2 FROM table1 WHERE column1 IN (SELECT column1 FROM table2 WHERE column2 = 'value');
When using subqueries, you should pay attention to the following points:
- Query filter conditions should be as accurate as possible to reduce the number of results of the subquery.
- The results of the subquery should be cached for use in the outer query.
To sum up, using join query can easily handle the query requirements of multi-table association. However, in order to improve query performance, we can adopt appropriate optimization strategies, such as creating indexes, accurately selecting fields, simplifying query conditions, etc. In addition, we can also consider using left joins, right joins or subqueries instead of join queries. By rationally applying these optimization methods, query speed and system performance can be significantly improved.
Reference code:
-- 创建索引 CREATE INDEX index_name ON table_name (column_name); -- 使用INNER JOIN优化连接查询 SELECT table1.column1, table1.column2, table2.column3 FROM table1 INNER JOIN table2 ON table1.id = table2.id; -- 使用LEFT JOIN优化连接查询 SELECT table1.column1, table1.column2, table2.column3 FROM table1 LEFT JOIN table2 ON table1.id = table2.id WHERE table2.id IS NULL; -- 使用子查询优化连接查询 SELECT column1, column2 FROM table1 WHERE column1 IN (SELECT column1 FROM table2 WHERE column2 = 'value');
The above is an introduction and code example on how to use MySQL's join query to optimize complex query operations. I hope this article can help you optimize query operations in practical applications.
The above is the detailed content of How to use MySQL's join query to optimize complex query operations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


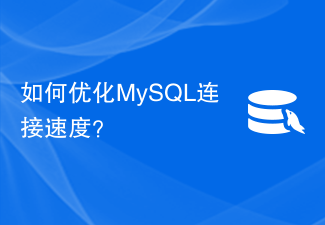
How to optimize MySQL connection speed? Overview: MySQL is a widely used relational database management system that is commonly used for data storage and management in a variety of applications. During development, optimization of MySQL connection speed is critical to improving application performance. This article will introduce some common methods and techniques for optimizing MySQL connection speed. Table of Contents: Use connection pools to adjust connection parameters and optimize network settings. Use indexing and caching to avoid long idle connections. Configure appropriate hardware resources. Summary: Use connection pools.
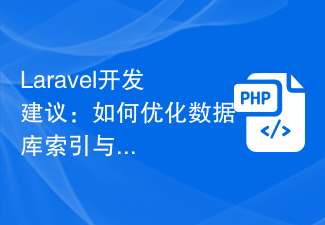
Laravel development suggestions: How to optimize database indexes and queries Introduction: In Laravel development, database queries are an inevitable link. Optimizing query performance is crucial to improving application response speed and user experience. This article will introduce how to improve the performance of Laravel applications by optimizing database indexes and queries. 1. Understand the role of database index. Database index is a data structure that can quickly locate the required data to improve query performance. An index is usually on one or more columns in a table
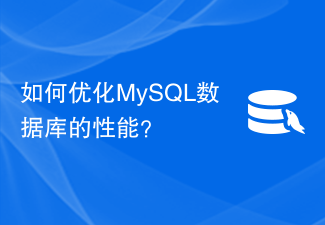
How to optimize the performance of MySQL database? In the modern information age, data has become an important asset for businesses and organizations. As one of the most commonly used relational database management systems, MySQL is widely used in all walks of life. However, as the amount of data increases and the load increases, the performance problems of the MySQL database gradually become apparent. In order to improve the stability and response speed of the system, it is crucial to optimize the performance of the MySQL database. This article will introduce some common MySQL database performance optimization methods to help readers
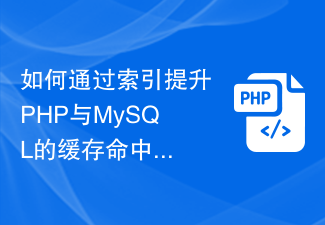
How to improve the cache hit rate and database query efficiency of PHP and MySQL through indexes? Introduction: PHP and MySQL are a commonly used combination when developing websites and applications. However, in order to optimize performance and improve user experience, we need to focus on the efficiency of database queries and cache hit rates. Among them, indexing is the key to improving query speed and cache efficiency. This article will introduce how to improve the cache hit rate and database query efficiency of PHP and MySQL through indexing, and give specific code examples. 1. Why use
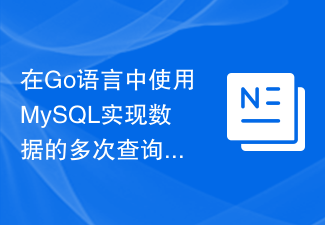
With the rapid development of Internet technology, data processing has become an important means for enterprises to achieve business goals. As the core of data storage and processing, databases also need to be continuously optimized to cope with the growing data volume and access requirements. This article will introduce the method of using MySQL to optimize multiple queries of data in Go language to improve query performance and usage efficiency. 1. The problem of multiple queries In actual business, we often need to query the database multiple times to obtain the required data, such as querying order information and related product information.
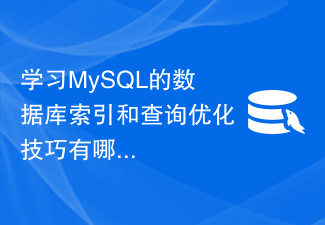
What are the database indexing and query optimization techniques for learning MySQL? In database processing, indexing and query optimization are very important. MySQL is a commonly used relational database management system with powerful query and optimization functions. This article will introduce some methods to learn MySQL database indexing and query optimization techniques, and illustrate them with code examples. 1. The role and use of indexes Indexes are a data structure used in databases to improve query performance. It can speed up data retrieval and reduce the execution of query statements
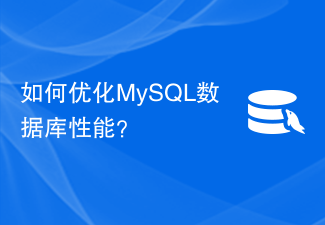
How to optimize MySQL database performance? MySQL is currently one of the most popular relational database management systems, but when dealing with large-scale data and complex queries, performance issues often become the number one worry for developers and database administrators. This article will introduce some methods and techniques for optimizing MySQL database performance to help you improve the response speed and efficiency of the database. Use the correct data type When designing a data table, choosing the appropriate data type can greatly improve the performance of the database. Make sure to use the smallest data type to store the number
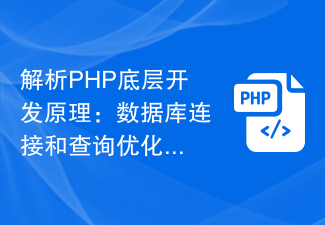
As a popular programming language, PHP is widely used in web development. In the underlying development process of PHP, database connection and query have always been important links. This article will delve into the database connection and query optimization in the underlying development principles of PHP. Database connection is an essential part of web applications. Generally speaking, the connection between PHP and the database is achieved through the use of database extension modules. PHP provides many extension modules, such as MySQL, SQLite, PostgreSQL, etc., which can
