


How to implement the observer pattern using Event Manager in the Phalcon framework
How to use the event manager (Event Manager) to implement the observer pattern in the Phalcon framework
Introduction:
The event manager (Event Manager) is one of the powerful and flexible core functions in the Phalcon framework . By using event managers, you can easily implement the Observer pattern to achieve loose coupling between objects in your application.
This article will introduce you to how to use the event manager in the Phalcon framework to implement the observer pattern and provide corresponding code examples.
Step 1: Install the Phalcon framework
First, make sure you have correctly installed and configured the Phalcon framework. If the installation has not been completed, please refer to Phalcon official documentation for installation.
Step 2: Create an event listener
In the Phalcon framework, you can inherit the PhalconEventsListener
class and implement its beforeNotify
or afterNotify
Method to create event listener.
The following is a simple example:
use PhalconEventsEvent; use PhalconMvcUserComponent; class MyListener extends Component { public function beforeNotify(Event $event, $source, $data) { echo "执行前,源对象:" . get_class($source) . ",数据:" . $data . "<br>"; } public function afterNotify(Event $event, $source, $data) { echo "执行后,源对象:" . get_class($source) . ",数据:" . $data . "<br>"; } }
In this example, the MyListener
class inherits from Phalcon’s basic component class Component
and implements beforeNotify
and afterNotify
methods. These methods will be executed before and after the event is triggered and output corresponding information.
Step 3: Bind event listener
Next, you need to bind the event listener to one or more events. In the Phalcon framework, this can be achieved through the attach
method of the event manager.
The following is the sample code:
use PhalconEventsManager; $eventsManager = new Manager(); $myListener = new MyListener(); $eventsManager->attach( 'notify:before', $myListener ); $eventsManager->attach( 'notify:after', $myListener );
In this example, we create an event manager $eventsManager
and instantiate the MyListener
class As event listener $myListener
. Then, bind the $myListener
listeners via the $eventsManager->attach
method to the objects named notify:before
and notify:after
event.
Step 4: Trigger the event
Finally, you can trigger the event at the appropriate location to perform the corresponding action. In the Phalcon framework, events can be triggered through the fire
method of the event manager.
The following is the sample code:
$eventsManager->fire( 'notify:before', $someObject, 'Some Data' ); $eventsManager->fire( 'notify:after', $someObject, 'Some Data' );
In this example, we triggered notify:before
and respectively on the event manager $eventsManager
notify:after
event. $someObject
is the source object that triggered the event, and 'Some Data'
is the data passed to the event listener.
After executing the above code, you will see the following output in the browser:
执行前,源对象:SomeObject,数据:Some Data 执行后,源对象:SomeObject,数据:Some Data
Summary:
Through the event manager of the Phalcon framework, you can easily implement the observer pattern, Achieve loose coupling between objects. In this article, we introduce the steps of how to use event listeners, bind event listeners to events, and trigger events, and provide corresponding code examples. I hope this article can help you better understand and apply the event manager functionality in the Phalcon framework.
The above is the detailed content of How to implement the observer pattern using Event Manager in the Phalcon framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


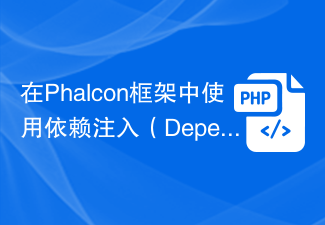
Introduction to the method of using dependency injection (DependencyInjection) in the Phalcon framework: In modern software development, dependency injection (DependencyInjection) is a common design pattern aimed at improving the maintainability and testability of the code. As a fast and low-cost PHP framework, the Phalcon framework also supports the use of dependency injection to manage and organize application dependencies. This article will introduce you how to use the Phalcon framework
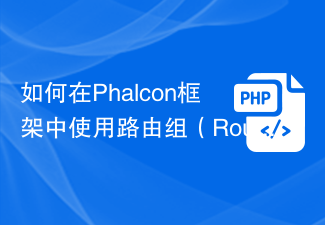
How to use RouteGroups in the Phalcon framework. In the Phalcon framework, routes are used to map URLs to specific controllers and actions. When we need to perform the same processing on a group of related URLs, we can use route groups (RouteGroups) to simplify our code. The main purpose of routing groups is to route URLs with the same prefix to the same set of controllers and actions. This helps us build applications with consistent URL structures
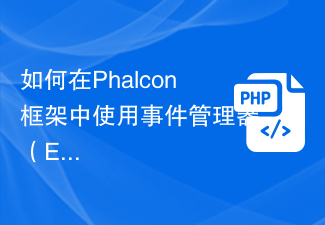
How to use the event manager (EventManager) function in the Phalcon framework Introduction: The event manager (EventManager) is a powerful component in the Phalcon framework. It can help us elegantly decouple business logic and improve code maintainability and flexibility. This article will introduce how to use the event manager function in the Phalcon framework and demonstrate its use through code examples. 1. Create an event manager in Phalcon, we can
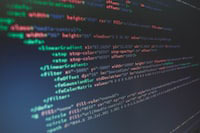
Introduction PHP design patterns are a set of proven solutions to common challenges in software development. By following these patterns, developers can create elegant, robust, and maintainable code. They help developers follow SOLID principles (single responsibility, open-closed, Liskov replacement, interface isolation and dependency inversion), thereby improving code readability, maintainability and scalability. Types of Design Patterns There are many different design patterns, each with its own unique purpose and advantages. Here are some of the most commonly used PHP design patterns: Singleton pattern: Ensures that a class has only one instance and provides a way to access this instance globally. Factory Pattern: Creates an object without specifying its exact class. It allows developers to conditionally
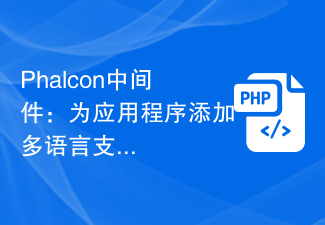
Phalcon middleware: Adding multi-language support and localization processing to applications As the process of globalization accelerates, more and more applications need to support multi-language and localization processing. In the Phalcon framework, we can add multi-language support and localization processing functions by using middleware. This article will introduce how to use middleware to achieve multi-language support and localization processing in Phalcon applications. First, we need to define a middleware in the Phalcon application that detects the language of the user
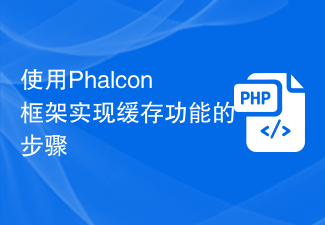
Steps to implement caching function using Phalcon framework Introduction: In web application development, caching function is one of the important means to improve performance. Phalcon is a high-performance PHP framework that provides rich caching functions. This article will introduce the steps to implement the caching function using the Phalcon framework and provide corresponding code examples. 1. Install the Phalcon framework and download the Phalcon framework: Visit the Phalcon official website (https://phalcon.io/en-u

The Phalcon framework is a PHP framework based on C extensions, which has faster speed and lower memory footprint than other PHP frameworks. In this article, we will introduce how to use Phalcon framework in PHP. Installing the Phalcon Framework Before using the Phalcon framework, we need to ensure that the Phalcon extension is installed. If it is not installed yet, please follow the steps below to install it: 1) Go to Phalcon official website (https://phalconphp.com
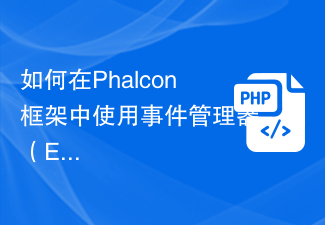
How to use the event manager (EventManager) to implement the observer pattern in the Phalcon framework Introduction: The event manager (EventManager) is one of the powerful and flexible core functions in the Phalcon framework. By using event managers, you can easily implement the Observer pattern to achieve loose coupling between objects in your application. This article will introduce you to how to use the event manager in the Phalcon framework to implement the observer pattern and provide corresponding code examples. step one
