How to implement a RESTful API using Flask
How to use Flask to implement RESTful API
Flask is a lightweight web framework written in Python, which provides a simple and easy way to develop web applications. One of the important features is that you can use Flask to build RESTful APIs. REST (Representational State Transfer) is a network architecture style that abstracts network resources into a limited set of states and operates these states through URIs.
This article will introduce you how to use Flask to implement a RESTful API and provide some code examples to help you understand better.
Step 1: Create a Flask application
First, we need to install Flask. You can install Flask from the command line using the following command:
1 |
|
After the installation is complete, we can start creating a Flask application. Create a file called app.py in your project folder and add the following code in the file:
1 2 3 4 5 6 |
|
The above code creates a Flask app called app and runs it in debug mode . You can start the app locally by running python app.py
.
Step 2: Define resources and routes
RESTful API mainly accesses and operates resources through URI. In Flask, we can achieve this by defining routes and view functions. The following is a simple example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
|
The above code creates a RESTful API with resources as tasks. /api/tasks
represents the task list resource, the GET method is used to obtain all tasks, and the POST method is used to create new tasks. When receiving a POST request, the code checks whether the title field is included in the request body, and returns an error response if not. If the request is legitimate, the code will create a new task based on the data in the request body and add it to the task list, and then return the details of the new task.
Step 3: Test the API
The API defined in step 2 can be tested using various tools, such as Postman or cURL. The following example is using cURL to test our API:
1 2 3 4 5 |
|
The above commands are used to send GET and POST requests to test the API. You can customize the request based on your actual situation.
Summary
It is very simple to implement a RESTful API using Flask. In this article, we briefly introduce how to use Flask to create a RESTful API and provide a simple example. We can further extend and optimize the code based on specific business needs. I hope this article helped you better understand how to use Flask to build RESTful APIs.
The above is the detailed content of How to implement a RESTful API using Flask. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




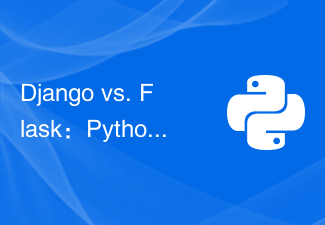
Django and Flask are both leaders in Python Web frameworks, and they both have their own advantages and applicable scenarios. This article will conduct a comparative analysis of these two frameworks and provide specific code examples. Development Introduction Django is a full-featured Web framework, its main purpose is to quickly develop complex Web applications. Django provides many built-in functions, such as ORM (Object Relational Mapping), forms, authentication, management backend, etc. These features allow Django to handle large
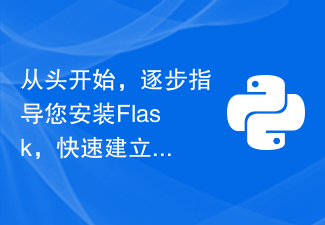
Starting from scratch, I will teach you step by step how to install Flask and quickly build a personal blog. As a person who likes writing, it is very important to have a personal blog. As a lightweight Python Web framework, Flask can help us quickly build a simple and fully functional personal blog. In this article, I will start from scratch and teach you step by step how to install Flask and quickly build a personal blog. Step 1: Install Python and pip Before starting, we need to install Python and pi first
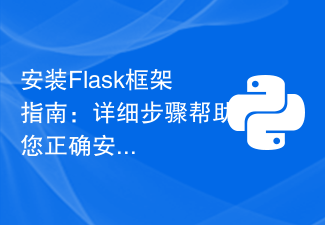
Flask framework installation tutorial: Teach you step by step how to correctly install the Flask framework. Specific code examples are required. Introduction: Flask is a simple and flexible Python Web development framework. It's easy to learn, easy to use, and packed with powerful features. This article will lead you step by step to correctly install the Flask framework and provide detailed code examples for reference. Step 1: Install Python Before installing the Flask framework, you first need to make sure that Python is installed on your computer. You can start from P
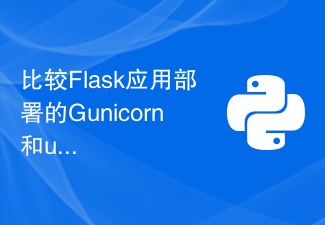
Flask application deployment: Comparison of Gunicorn vs suWSGI Introduction: Flask, as a lightweight Python Web framework, is loved by many developers. When deploying a Flask application to a production environment, choosing the appropriate Server Gateway Interface (SGI) is a crucial decision. Gunicorn and uWSGI are two common SGI servers. This article will describe them in detail.
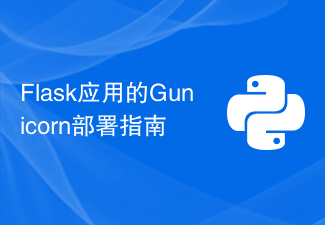
How to deploy Flask application using Gunicorn? Flask is a lightweight Python Web framework that is widely used to develop various types of Web applications. Gunicorn (GreenUnicorn) is a Python-based HTTP server used to run WSGI (WebServerGatewayInterface) applications. This article will introduce how to use Gunicorn to deploy Flask applications, with
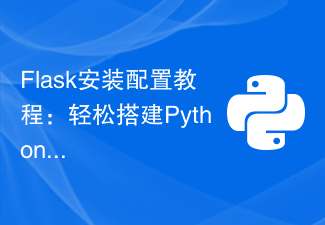
Flask installation and configuration tutorial: A tool to easily build Python Web applications, specific code examples are required. Introduction: With the increasing popularity of Python, Web development has become one of the necessary skills for Python programmers. To carry out web development in Python, we need to choose a suitable web framework. Among the many Python Web frameworks, Flask is a simple, easy-to-use and flexible framework that is favored by developers. This article will introduce the installation of Flask framework,
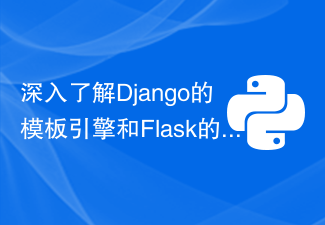
In-depth understanding of Django's template engine and Flask's Jinja2 requires specific code examples. Introduction: Django and Flask are two commonly used and popular web frameworks in Python. They both provide powerful template engines to handle the rendering of dynamic web pages. Django uses its own template engine, while Flask uses Jinja2. This article will take an in-depth look at Django’s template engine and Flask’s Jinja2, and provide some concrete code examples to illustrate their use.
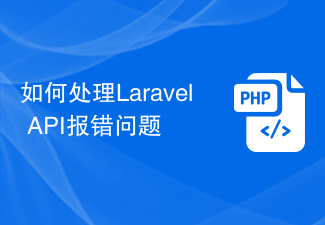
Title: How to deal with Laravel API error problems, specific code examples are needed. When developing Laravel, API errors are often encountered. These errors may come from various reasons such as program code logic errors, database query problems, or external API request failures. How to handle these error reports is a key issue. This article will use specific code examples to demonstrate how to effectively handle Laravel API error reports. 1. Error handling in Laravel
