How to use Go language for code optimization practice
How to use Go language for code optimization practice
Introduction:
In the development process, the performance and efficiency of the code are crucial. Optimizing code can improve the running speed of the program, reduce resource consumption and improve user experience. This article will introduce how to use Go language for code optimization practice, and provide some sample code to help readers better understand.
1. Reduce memory allocation
In the Go language, memory allocation is a costly operation. Therefore, reducing memory allocation can improve the performance of your program. Here are some ways to reduce memory allocation:
- Use value types instead of pointer types
type User struct { Name string Age int } func main() { u := User{ Name: "Alice", Age: 20, } // 使用值类型而不是指针类型 updateUser(u) fmt.Println(u) } func updateUser(u User) { u.Name = "Bob" }
- Use sync.Pool
var bufPool = sync.Pool{ New: func() interface{} { // 创建一个新的缓冲区 return make([]byte, 1024) }, } func main() { buf := bufPool.Get().([]byte) // 使用缓冲区 // ... // 使用完之后放回缓冲池 bufPool.Put(buf) }
2. Use concurrent programming
Go language inherently supports concurrent programming, and you can use goroutine and channel as needed to improve the concurrency performance of the program. Here are some ways to use concurrent programming:
- Using goroutine for parallel computing
func main() { nums := []int{1, 2, 3, 4, 5} resultChan := make(chan int, len(nums)) for _, num := range nums { go square(num, resultChan) } sum := 0 for i := 0; i < len(nums); i++ { sum += <-resultChan } fmt.Println(sum) } func square(num int, resultChan chan<- int) { time.Sleep(100 * time.Millisecond) // 模拟计算耗时 resultChan <- num * num }
- Use sync.WaitGroup to wait for concurrent tasks to complete
func main() { var wg sync.WaitGroup wg.Add(2) go func() { defer wg.Done() // 执行任务1 }() go func() { defer wg.Done() // 执行任务2 }() wg.Wait() // 等待所有任务完成 }
3. Use appropriate data structures and algorithms
Choosing appropriate data structures and algorithms can also significantly improve program performance. Here are some examples of using appropriate data structures and algorithms:
- Use map instead of slice for lookup operations
func main() { s := []int{1, 2, 3, 4, 5} m := make(map[int]bool) for _, num := range s { m[num] = true } fmt.Println(m[3]) // 输出:true }
- Use the sort package for sorting
func main() { s := []int{5, 3, 1, 4, 2} sort.Ints(s) fmt.Println(s) // 输出:[1 2 3 4 5] }
Conclusion:
By reducing memory allocation, using concurrent programming and choosing appropriate data structures and algorithms, we can effectively optimize the performance of Go language code. However, code optimization is not static and needs to be adjusted and tested on a case-by-case basis. In practice, we should comprehensively consider various factors based on the characteristics of the code and actual needs to obtain the best performance and efficiency.
Reference materials:
- Go language official documentation: https://golang.org/doc/
- Go language concurrency model: https://blog. golang.org/concurrency-is-not-parallelism
The above is the detailed content of How to use Go language for code optimization practice. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


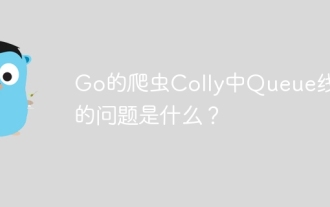
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
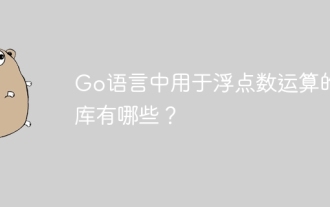
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
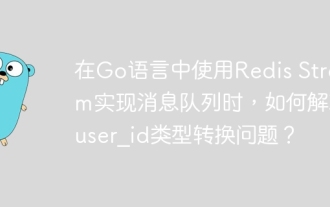
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
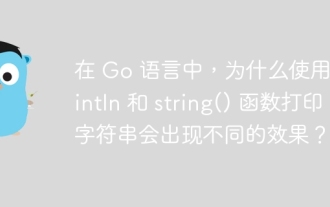
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
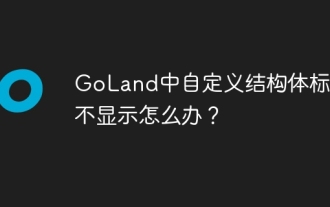
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
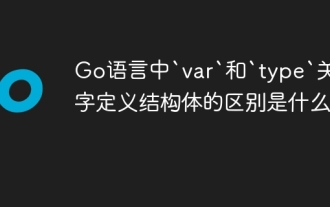
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
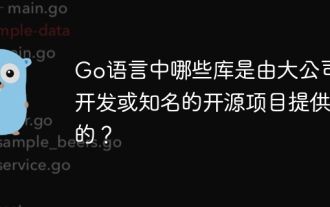
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
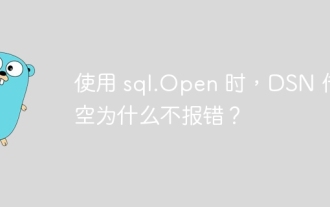
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
