How to use Go language for error handling
How to use Go language for error handling
Error handling is an essential part of programming. It can help us better handle error situations that may occur in the program. Error handling is a very important topic in Go language. This article will introduce how to use Go language for error handling and provide some practical code examples.
- Error handling basics
In the Go language, errors are usually passed through return values. A function can return multiple values, one of which can be of type error. If the function executes successfully, the value of error is nil; if the function fails, the corresponding error message is returned.
Here is a simple example that demonstrates how to return an error in Go language:
package main import ( "errors" "fmt" ) func divide(a, b int) (int, error) { if b == 0 { return 0, errors.New("division by zero error") } return a / b, nil } func main() { result, err := divide(10, 0) if err != nil { fmt.Println("Error:", err) return } fmt.Println("Result:", result) }
In the above code, the divide
function is used to calculate two integers quotient. If the divisor is 0, a non-nil error is returned.
- Custom error types
In addition to using the errors.New
function to create basic error types, we can also customize error types so that Better describe the specific circumstances of the error.
The following is an example showing how to customize an error type:
package main import ( "fmt" ) type MyError struct { Msg string Code int } func (e *MyError) Error() string { return fmt.Sprintf("Error: %s (Code: %d)", e.Msg, e.Code) } func process() error { return &MyError{"something went wrong", 500} } func main() { err := process() if err != nil { fmt.Println(err) } }
In the above code, MyError
is a custom error type that contains error messages and error codes. By overriding the Error
method, we can provide a custom string representation for the error type.
- Chained calls for error handling
In actual development, we may encounter situations where multiple functions need to handle the same error. At this time, we can use chain calls to handle errors.
Here is an example that demonstrates how to use chained calls to handle errors:
package main import ( "errors" "fmt" ) func process1() error { return errors.New("error in process1") } func process2() error { err := process1() if err != nil { return fmt.Errorf("error in process2: %w", err) } return nil } func process3() { err := process2() if err != nil { fmt.Println(err) } } func main() { process3() }
In the above code, the process1
and process2
functions will return an error. In the process2
function, we use the fmt.Errorf
function to encapsulate the error returned by process1
to better describe where the error occurred.
- Use defer for error handling
In the Go language, you can use the defer
statement to delay the execution of a piece of code, which is very useful in error handling. practical. By using defer
, we can ensure that some code will be executed before the function returns, regardless of whether an error occurred.
Here is an example showing how to use defer
for error handling:
package main import ( "errors" "fmt" ) func process() error { file, err := openFile("file.txt") if err != nil { return err } defer file.Close() // 处理文件操作 return nil } func openFile(fileName string) (*File, error) { // 打开文件操作 // ... if err != nil { return nil, fmt.Errorf("failed to open file: %w", err) } return file, nil } func main() { err := process() if err != nil { fmt.Println(err) } }
In the above code, the process
function is called openFile
function to open a file and close the file through the defer
statement before the function returns. In this way, regardless of whether an error occurs, we can guarantee that the file will be closed before the function returns.
Summary:
By studying this article, we learned how to use Go language for error handling. We learned the basics of error handling, mastered custom error types, error handling chain calls, and how to use defer
for error handling. Error handling is very important for writing robust programs, and we should actively use error handling techniques in actual development.
The above is the detailed content of How to use Go language for error handling. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


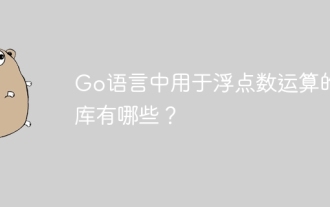
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
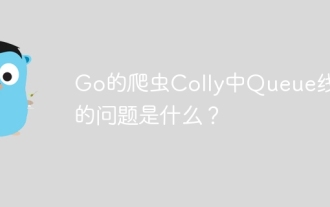
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
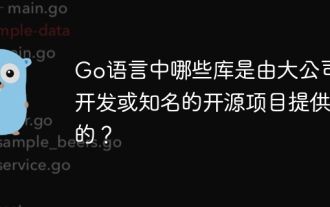
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
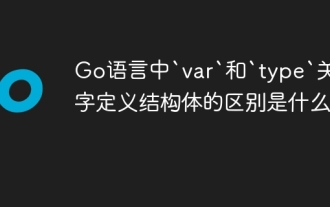
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
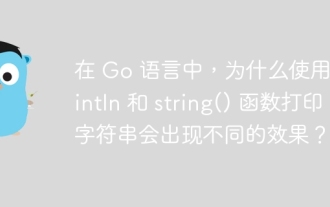
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
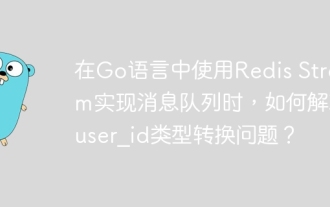
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
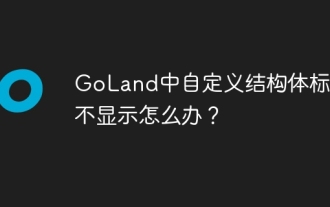
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
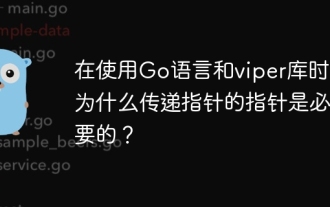
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
