


How to write automatic data cleaning function of CMS system in Python
How to use Python to write the automatic data cleaning function of the CMS system
In modern CMS (Content Management System) systems, the accumulation of data is inevitable. Over time, huge amounts of data can cause system performance to degrade, and the accumulation of useless data can take up server storage space. Therefore, in order to ensure the efficient operation of the system, we need an automatic data cleaning function to regularly clean up useless data.
Python is a powerful programming language that provides many libraries and tools for processing data and automating tasks. In this article, we will use Python to write the automatic data cleaning function of the CMS system and provide code examples.
First, we need to determine the type of data to be cleaned. Common useless data types include expired member accounts, expired articles, invalid comments, etc. Therefore, before actually writing the code, you need to do some system analysis to determine the type of data to be cleaned and the corresponding cleaning strategy.
Next, we can start writing code. The following is an example that shows how to use Python to write a function to clean up expired member accounts:
import datetime def clean_expired_accounts(): # 在这里编写清理过期会员账号的代码 # 获取当前日期和时间 current_date = datetime.datetime.now().date() # 查询数据库,找到过期的会员账号 expired_accounts = Member.objects.filter(expiration_date__lt=current_date) # 删除过期的会员账号 expired_accounts.delete() # 记录清理日志 log_message = f"{len(expired_accounts)} expired member accounts have been cleaned." write_to_log(log_message)
In this example, we use Python's datetime library to get the current date and time. Then, we query the database to find the expired member account and delete it using the delete() method. Finally, we log the results of the cleanup operation.
In addition, we can also write code for other cleaning functions. The following is an example of cleaning up expired articles:
def clean_expired_articles(): # 在这里编写清理过期文章的代码 # 获取当前日期和时间 current_date = datetime.datetime.now().date() # 查询数据库,找到过期的文章 expired_articles = Article.objects.filter(expiration_date__lt=current_date) # 删除过期的文章 expired_articles.delete() # 记录清理日志 log_message = f"{len(expired_articles)} expired articles have been cleaned." write_to_log(log_message)
In this example, we use similar logic to cleaning up expired member accounts to clean up expired articles.
In addition to regularly cleaning expired data, we can also write codes for other cleaning functions according to needs, such as cleaning invalid comments, cleaning unused images, etc. It is important that we write corresponding cleaning strategies based on the actual situation and ensure that the data cleaning process is safe and reliable.
To summarize, Python provides us with powerful tools to write the automatic data cleaning function of the CMS system. By properly planning the cleaning strategy and writing corresponding code, we can effectively clean up useless data and improve system performance and availability.
I hope the code examples and ideas provided in this article can help you write the automatic data cleaning function of the CMS system. I wish your CMS system always operates efficiently!
The above is the detailed content of How to write automatic data cleaning function of CMS system in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


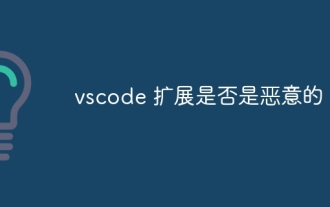
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
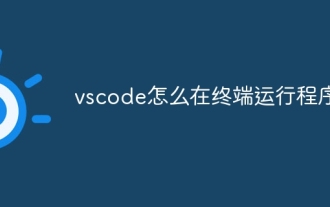
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
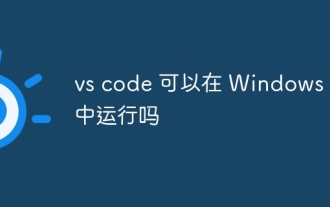
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
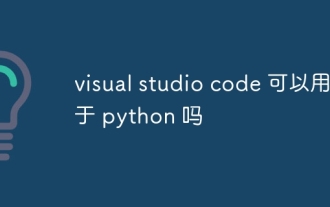
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
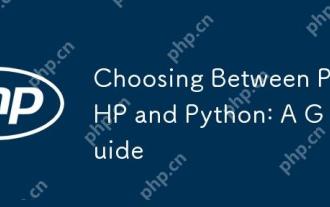
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
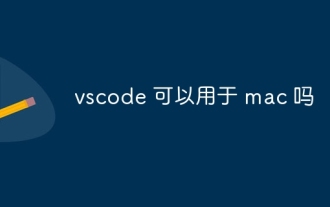
VS Code is available on Mac. It has powerful extensions, Git integration, terminal and debugger, and also offers a wealth of setup options. However, for particularly large projects or highly professional development, VS Code may have performance or functional limitations.
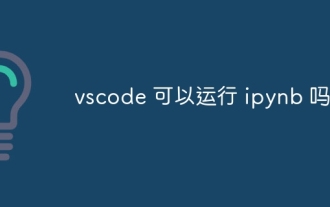
The key to running Jupyter Notebook in VS Code is to ensure that the Python environment is properly configured, understand that the code execution order is consistent with the cell order, and be aware of large files or external libraries that may affect performance. The code completion and debugging functions provided by VS Code can greatly improve coding efficiency and reduce errors.
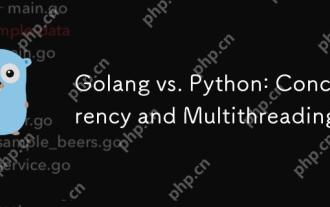
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
