


How to use Python to build the plug-in management function of the CMS system
How to use Python to build the plug-in management function of the CMS system
With the rapid development of the Internet, the CMS system (Content Management System, content management system) has become the first choice of many website developers and managers. The CMS system can help users easily create and manage website content, making website construction and maintenance easier and more efficient. However, different websites may require different functions and features, which requires a CMS system that can easily add and manage various plug-ins. This article will introduce how to use Python to build the plug-in management function of the CMS system, accompanied by code examples.
1. Design Ideas
Before building the plug-in management function of the CMS system, we need to first clarify the requirements and functions of plug-in management. A basic plug-in management system should include the following functions:
- Adding and uninstalling plug-ins: users can easily add plug-ins by uploading or specifying paths, and can also uninstall unnecessary plug-ins. .
- Enabling and disabling plug-ins: Users can enable or disable specific plug-ins as needed so that they can be used at different times and scenarios.
- Configuration and management of plug-ins: Users can configure and manage plug-ins through the CMS system interface, including modifying plug-in settings, customizing plug-in functions, etc.
Based on the above requirements, we can design a simple plug-in management system. The system mainly consists of two parts: the plug-in manager and the plug-in itself. The plug-in manager is responsible for adding, uninstalling, enabling, and disabling plug-ins, while the plug-in itself is responsible for the actual function implementation. The plug-in manager communicates with the plug-in through a certain interface to realize the configuration and management of the plug-in.
2. Implementation steps
- Create a plug-in manager: We can create a PluginManager class, which contains methods such as add, uninstall, enable and disable. The sample code is as follows:
class PluginManager: def __init__(self): self.plugins = [] def add_plugin(self, plugin): self.plugins.append(plugin) def remove_plugin(self, plugin): if plugin in self.plugins: self.plugins.remove(plugin) def enable_plugin(self, plugin_name): for plugin in self.plugins: if plugin.name == plugin_name: plugin.enable() def disable_plugin(self, plugin_name): for plugin in self.plugins: if plugin.name == plugin_name: plugin.disable()
- Create a plug-in base class: We can create a Plugin base class, define the basic properties and methods of the plug-in in this class, and provide some interfaces for the plug-in manager transfer. The sample code is as follows:
class Plugin: def __init__(self, name): self.name = name self.enabled = False def enable(self): self.enabled = True # 在这里实现插件的启用逻辑 def disable(self): self.enabled = False # 在这里实现插件的禁用逻辑 def configure(self): # 在这里实现插件的配置逻辑 def run(self): if self.enabled: # 在这里实现插件的功能逻辑
- Create specific plug-in classes: For different plug-ins, we can create specific plug-in classes and inherit the Plugin base class. The sample code is as follows:
class HelloWorldPlugin(Plugin): def __init__(self): super().__init__("HelloWorld") def run(self): if self.enabled: print("Hello, World!") class CounterPlugin(Plugin): def __init__(self): super().__init__("Counter") self.counter = 0 def run(self): if self.enabled: self.counter += 1 print("Current count:", self.counter)
- Use the plug-in manager: In actual use, we can use the PluginManager to manage operations such as adding, uninstalling, enabling and disabling plug-ins. The sample code is as follows:
# 创建插件管理器 plugin_manager = PluginManager() # 创建并添加插件 hello_world_plugin = HelloWorldPlugin() counter_plugin = CounterPlugin() plugin_manager.add_plugin(hello_world_plugin) plugin_manager.add_plugin(counter_plugin) # 启用插件 plugin_manager.enable_plugin("HelloWorld") plugin_manager.enable_plugin("Counter") # 运行插件 for plugin in plugin_manager.plugins: plugin.run()
3. Summary
Through the above steps, we can use Python to build a simple plug-in management function for the CMS system. The plug-in manager is responsible for adding, uninstalling, enabling, and disabling plug-ins, while the plug-in itself is responsible for the actual function implementation. Through the interface between the plug-in manager and the plug-in, we can easily configure and manage the plug-in. I hope this article can provide some reference and help for the plug-in management function when using Python to build a CMS system.
The above is the detailed content of How to use Python to build the plug-in management function of the CMS system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


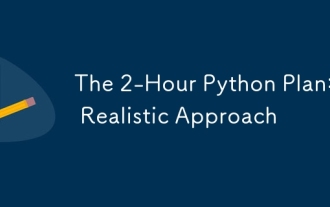
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
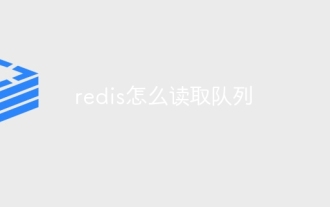
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
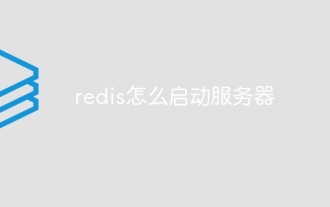
The steps to start a Redis server include: Install Redis according to the operating system. Start the Redis service via redis-server (Linux/macOS) or redis-server.exe (Windows). Use the redis-cli ping (Linux/macOS) or redis-cli.exe ping (Windows) command to check the service status. Use a Redis client, such as redis-cli, Python, or Node.js, to access the server.
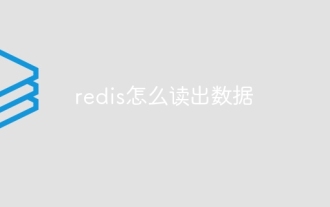
To read data from Redis, you can follow these steps: 1. Connect to the Redis server; 2. Use get(key) to get the value of the key; 3. If you need string values, decode the binary value; 4. Use exists(key) to check whether the key exists; 5. Use mget(keys) to get multiple values; 6. Use type(key) to get the data type; 7. Redis has other read commands, such as: getting all keys in a matching pattern, using cursors to iterate the keys, and sorting the key values.
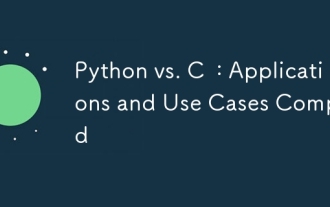
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
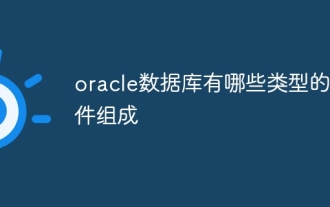
Oracle database file structure includes: data file: storing actual data. Control file: Record database structure information. Redo log files: record transaction operations to ensure data consistency. Parameter file: Contains database running parameters to optimize performance. Archive log file: Backup redo log file for disaster recovery.

There are several ways to find keys in Redis: Use the SCAN command to iterate over all keys by pattern or condition. Use GUI tools such as Redis Explorer to visualize the database and filter keys by name or schema. Write external scripts to query keys using the Redis client library. Subscribe to keyspace notifications to receive alerts when key changes.
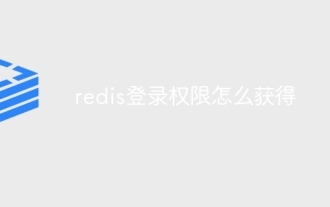
To obtain Redis login permission, you need to perform the following steps: 1. Create a username and password; 2. Allow remote connections; 3. Restart the Redis server; 4. Connect using the Redis CLI or programming language.
