


How to use parallel computing to speed up the running of Python programs
How to use parallel computing to accelerate the running of Python programs
With the continuous improvement of computer performance, we are increasingly faced with the need to process large-scale data and complex computing tasks. As a simple and easy-to-use programming language, Python is also widely used in data processing, scientific computing and other fields. However, due to the interpreted characteristics of Python, speed often becomes a bottleneck limiting program performance when processing large-scale data and complex computing tasks.
In order to make full use of the computer's multi-core processing capabilities, we can use parallel computing to speed up the running of Python programs. Parallel computing means that multiple tasks are executed simultaneously at the same time, and a large computing task is divided into several subtasks for parallel calculation.
In Python, there are a variety of libraries that can implement parallel computing, such as multiprocessing, concurrent.futures, etc. Below we will take the multiprocessing library as an example to introduce how to use parallel computing to speed up the running of Python programs.
First, we need to import the multiprocessing library:
import multiprocessing
Below, we take the calculation of the Fibonacci sequence as an example to demonstrate how to use parallel computing to accelerate program running. The Fibonacci sequence refers to a sequence in which each number is the sum of the previous two numbers, such as 0, 1, 1, 2, 3, 5...
Let’s first take a look at the common serial algorithm used to calculate the Fibonacci sequence:
def fibonacci(n): if n <= 1: return n else: return fibonacci(n-1) + fibonacci(n-2) result = fibonacci(30) print(result)
In the above code, we define a recursive function fibonacci()
To calculate the nth number of Fibonacci sequence. Then, we call fibonacci(30)
to calculate the 30th Fibonacci number and print the result.
Next, we use the multiprocessing library to calculate the Fibonacci sequence in parallel:
def fibonacci(n): if n <= 1: return n else: return fibonacci(n-1) + fibonacci(n-2) def fibonacci_parallel(n): pool = multiprocessing.Pool() result = pool.map(fibonacci, range(n+1)) pool.close() pool.join() return result[n] result = fibonacci_parallel(30) print(result)
In the above code, we first define the fibonacci()
function, and Same as the normal serial algorithm before. Then, we define the fibonacci_parallel()
function, where we use multiprocessing.Pool()
to create a process pool, and then use the pool.map()
method To calculate the first n numbers of the Fibonacci sequence in parallel. Finally, we close the process pool and use pool.join()
to wait for the end of all child processes and return the nth Fibonacci number.
Through the improvement of the above code, we allocate the calculation tasks to multiple sub-processes in parallel, making full use of the computer's multi-core processing capabilities and greatly speeding up the calculation of the Fibonacci sequence.
In addition to using the multiprocessing library, you can also use the concurrent.futures library to implement parallel computing. The following is a sample code using the concurrent.futures library:
import concurrent.futures def fibonacci(n): if n <= 1: return n else: return fibonacci(n-1) + fibonacci(n-2) def fibonacci_parallel(n): with concurrent.futures.ProcessPoolExecutor() as executor: futures = [executor.submit(fibonacci, i) for i in range(n+1)] result = [future.result() for future in concurrent.futures.as_completed(futures)] return result[n] result = fibonacci_parallel(30) print(result)
In the above code, we first imported the concurrent.futures library. Then, we defined the fibonacci()
function and the fibonacci_parallel()
function, similar to the previous example code. In the fibonacci_parallel()
function, we use concurrent.futures.ProcessPoolExecutor()
to create a process pool, and then use the executor.submit()
method to submit the calculation task and returns a future object. Finally, we use the concurrent.futures.as_completed()
method to get the calculation result and return the nth Fibonacci number.
To sum up, using parallel computing is an effective way to speed up the running of Python programs. By properly allocating tasks to multiple sub-processes or threads and making full use of the computer's multi-core processing capabilities, we can significantly improve the running speed of the program. In practical applications, we can select libraries suitable for parallel computing based on the characteristics of specific data processing or computing tasks, and perform appropriate parameter tuning to achieve better performance improvements.
(Note: In order to better demonstrate the effect of parallel computing, the Fibonacci sequence calculation task in the above example code is relatively simple. In actual applications, the code and parameters may need to be optimized according to specific needs. )
The above is the detailed content of How to use parallel computing to speed up the running of Python programs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


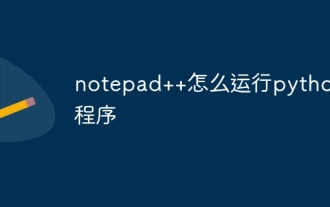
Using Notepad++ to run a Python program requires the following steps: 1. Install the Python plug-in; 2. Create a Python file; 3. Set the run options; 4. Run the program.
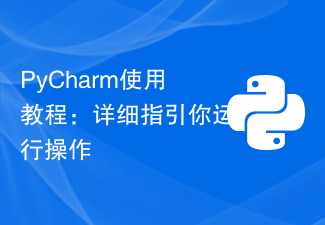
PyCharm is a very popular Python integrated development environment (IDE). It provides a wealth of functions and tools to make Python development more efficient and convenient. This article will introduce you to the basic operation methods of PyCharm and provide specific code examples to help readers quickly get started and become proficient in operating the tool. 1. Download and install PyCharm First, we need to go to the PyCharm official website (https://www.jetbrains.com/pyc
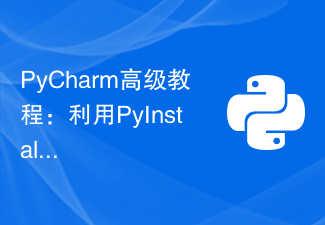
PyCharm is a powerful Python integrated development environment that provides a wealth of functions and tools to help developers improve efficiency. Among them, PyInstaller is a commonly used tool that can package Python code into an executable file (EXE format) to facilitate running on machines without a Python environment. In this article, we will introduce how to use PyInstaller in PyCharm to package Python code into EXE format, and provide specific
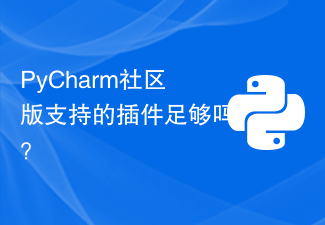
Does PyCharm Community Edition support enough plugins? Need specific code examples As the Python language becomes more and more widely used in the field of software development, PyCharm, as a professional Python integrated development environment (IDE), is favored by developers. PyCharm is divided into two versions: professional version and community version. The community version is provided for free, but its plug-in support is limited compared to the professional version. So the question is, does PyCharm Community Edition support enough plug-ins? This article will use specific code examples to
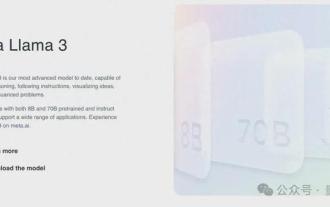
Llama3 is here! Just now, Meta’s official website was updated and the official announced Llama 38 billion and 70 billion parameter versions. And it is an open source SOTA after its launch: Meta official data shows that the Llama38B and 70B versions surpass all opponents in their respective parameter scales. The 8B model outperforms Gemma7B and Mistral7BInstruct on many benchmarks such as MMLU, GPQA, and HumanEval. The 70B model has surpassed the popular closed-source fried chicken Claude3Sonnet, and has gone back and forth with Google's GeminiPro1.5. As soon as the Huggingface link came out, the open source community became excited again. The sharp-eyed blind students also discovered immediately
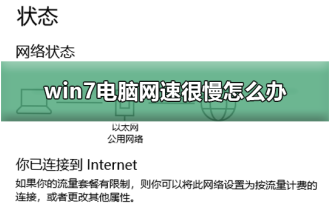
Many friends who use win7 system computers find that the Internet speed is extremely slow when using the computer. What is happening? It may be that there are certain restrictions on the network in your network settings. Today I will teach you how to remove network restrictions and make the network speed extremely fast. Just select the advanced settings and change the value to "20MHz/ 40MHzauto" is enough. Let’s take a look at the specific tutorials. Methods to improve the network speed of win7 computer 1. The editor takes the win7 system as an example to illustrate. Right-click the "Network" icon on the right side of the desktop taskbar and select "Network and Sharing Center" to open it. 2. Click "Change Adapter Settings" in the newly appeared interface, then right-click "Local Area Connection" and select "Properties" to open. 3. In the open "Local
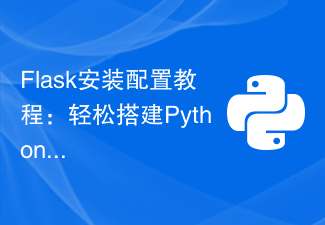
Flask installation and configuration tutorial: A tool to easily build Python Web applications, specific code examples are required. Introduction: With the increasing popularity of Python, Web development has become one of the necessary skills for Python programmers. To carry out web development in Python, we need to choose a suitable web framework. Among the many Python Web frameworks, Flask is a simple, easy-to-use and flexible framework that is favored by developers. This article will introduce the installation of Flask framework,
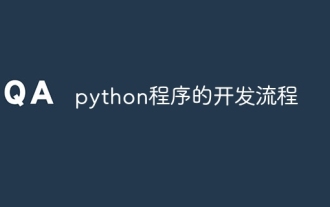
The Python program development process includes the following steps: Requirements analysis: clarify business needs and project goals. Design: Determine architecture and data structures, draw flowcharts or use design patterns. Writing code: Program in Python, following coding conventions and documentation comments. Testing: Writing unit and integration tests, conducting manual testing. Review and Refactor: Review code to find flaws and improve readability. Deploy: Deploy the code to the target environment. Maintenance: Fix bugs, improve functionality, and monitor updates.
