How to write custom menu functions for CMS systems in Python
How to write the custom menu function of the CMS system in Python
When developing and designing a CMS (content management system), the custom menu function is a very important part. It allows users to customize the menu according to their needs and preferences for quick access to various features and pages. In this article, we will write a simple CMS system using Python and add custom menu functionality.
First, we need to create a menu class to store and manage menu item information. Each menu item contains a name and corresponding page path. We can use a dictionary to represent a collection of menu items, where the key is the menu name and the value is the page path. Here is the sample code for the menu class:
class Menu: def __init__(self): self.menu_items = {} def add_menu_item(self, name, path): self.menu_items[name] = path def remove_menu_item(self, name): if name in self.menu_items: del self.menu_items[name] def get_menu_items(self): return self.menu_items
Next, we need to write a function to display the menu. This function will perform the corresponding operation based on the user's selection. Here is a simple sample code:
def show_menu(menu): print("===== 自定义菜单 =====") menu_items = menu.get_menu_items() for index, (name, path) in enumerate(menu_items.items(), start=1): print(f"{index}. {name}") print("====================") choice = int(input("请选择一个菜单项: ")) if 1 <= choice <= len(menu_items): selected_menu_item = list(menu_items.keys())[choice - 1] print(f"你选择了菜单项 '{selected_menu_item}',页面路径为 '{menu_items[selected_menu_item]}'") else: print("无效的选择!")
Now, we can use this code in the main program to create a CMS system and add custom menu functionality. The following is a sample code:
def main(): menu = Menu() menu.add_menu_item("首页", "/") menu.add_menu_item("文章列表", "/articles") menu.add_menu_item("用户管理", "/users") show_menu(menu) if __name__ == "__main__": main()
In this example, we first create a Menu object and add three menu items using the add_menu_item() method. Then, we call the show_menu() function to display the menu and perform the corresponding actions based on the user selection.
This is just a simple example, you can extend and modify the code according to your actual needs. For example, you can add more menu items, improve the style of menu display, perform additional actions when selecting menu items, and more.
To summarize, we wrote a simple CMS system using Python and added custom menu functions. Through this example, you can learn how to use object-oriented programming and basic user interaction to implement this functionality. I wish you success in developing a CMS system!
The above is the detailed content of How to write custom menu functions for CMS systems in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


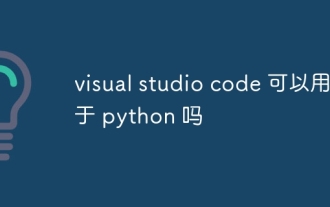
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
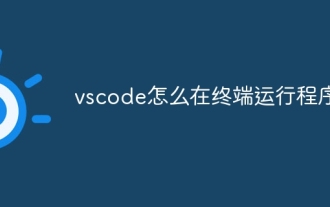
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
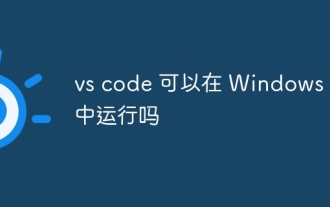
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
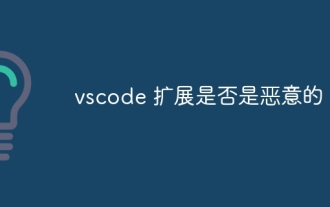
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
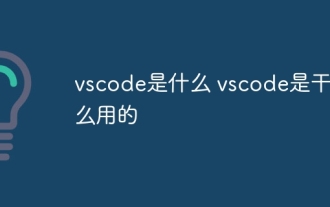
VS Code is the full name Visual Studio Code, which is a free and open source cross-platform code editor and development environment developed by Microsoft. It supports a wide range of programming languages and provides syntax highlighting, code automatic completion, code snippets and smart prompts to improve development efficiency. Through a rich extension ecosystem, users can add extensions to specific needs and languages, such as debuggers, code formatting tools, and Git integrations. VS Code also includes an intuitive debugger that helps quickly find and resolve bugs in your code.
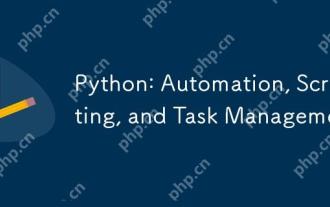
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
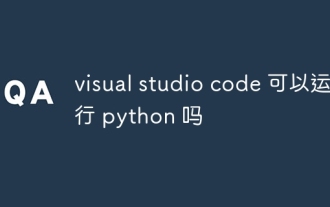
VS Code not only can run Python, but also provides powerful functions, including: automatically identifying Python files after installing Python extensions, providing functions such as code completion, syntax highlighting, and debugging. Relying on the installed Python environment, extensions act as bridge connection editing and Python environment. The debugging functions include setting breakpoints, step-by-step debugging, viewing variable values, and improving debugging efficiency. The integrated terminal supports running complex commands such as unit testing and package management. Supports extended configuration and enhances features such as code formatting, analysis and version control.
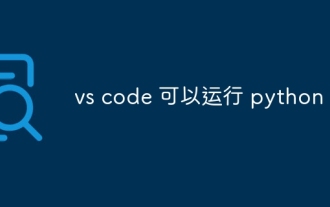
Yes, VS Code can run Python code. To run Python efficiently in VS Code, complete the following steps: Install the Python interpreter and configure environment variables. Install the Python extension in VS Code. Run Python code in VS Code's terminal via the command line. Use VS Code's debugging capabilities and code formatting to improve development efficiency. Adopt good programming habits and use performance analysis tools to optimize code performance.
