


PHP Baidu Translation API implements Italian to Chinese translation steps analysis
PHP Baidu Translation API implements Italian to Chinese translation steps analysis
Introduction:
With the development of globalization and the increasingly frequent international exchanges, communication between languages has become particularly important. When it comes to language translation, Baidu Translation API is a powerful and reliable solution. This article will introduce how to use PHP to write code to implement Italian to Chinese translation function.
Step 1: Preparation
First, we need to create and register an API account on the Baidu Developer Platform, and obtain the APP ID and secret key of the application for subsequent identity verification.
Step 2: Introduce the necessary files
Before you start writing code, you need to introduce the SDK file of Baidu Translation API. You can install it by adding the following code in the composer.json file:
{ "require": { "baidu-aip/aip-sdk": "^2.9" } }
Then use require
or require_once
to introduce the SDK file in the code:
require 'vendor/autoload.php'; use AipAipTranslate;
Step 3: Set authentication information
In the code, we need to use the APP ID and secret key of the API account previously created on the Baidu Developer Platform for authentication. Set them as variables:
$appId = 'YourAPPID'; $apiKey = 'YourAPIKey'; $secretKey = 'YourSecretKey';
Next, by instantiating the AipTranslate
class and passing in the authentication information:
$translate = new AipTranslate($appId, $apiKey, $secretKey);
Step 4: Perform the translation
Now we can start translating. Use the $translate->translate()
function to perform the translation and set the target language to 'zh' for Chinese:
$result = $translate->translate('我爱你', 'it', 'zh');
The above code will convert the Italian phrase "I love You" is translated into Chinese and the result is saved in the $result
variable.
Step 5: Processing results
Finally, we can get the translation result through the $result
variable and print it out:
echo $result['trans_result'][0]['dst'];
The above code will return Translate the results and output them to the browser.
Complete sample code:
require 'vendor/autoload.php'; use AipAipTranslate; $appId = 'YourAPPID'; $apiKey = 'YourAPIKey'; $secretKey = 'YourSecretKey'; $translate = new AipTranslate($appId, $apiKey, $secretKey); $result = $translate->translate('我爱你', 'it', 'zh'); echo $result['trans_result'][0]['dst'];
In actual development, relevant functions can be customized as needed to better manage and control API calls.
Conclusion:
This article provides steps and sample code for writing code in PHP to implement the Italian to Chinese translation function. By using Baidu Translation API, we can easily achieve communication and translation between languages, providing a more convenient and efficient solution for globalization.
The above is the detailed content of PHP Baidu Translation API implements Italian to Chinese translation steps analysis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
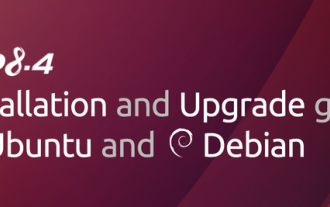
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
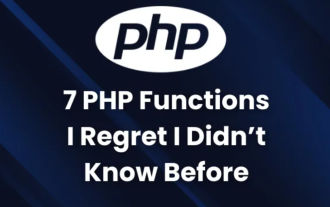
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
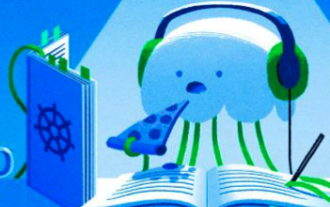
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
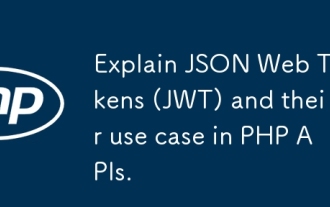
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
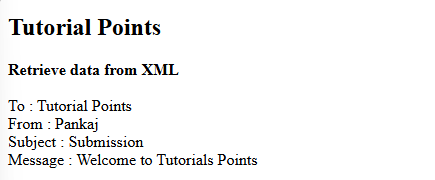
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
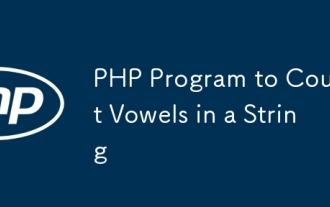
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
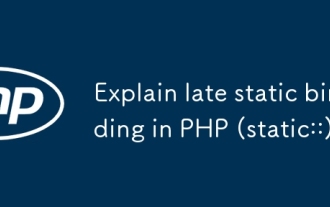
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
