How to use Java to implement the document conversion function of CMS system
How to use Java to implement the document conversion function of the CMS system
In the content management system (CMS), the document conversion function is an important function. It allows users to convert documents in different formats into other formats for easy sharing, editing and reading. In this article, we will introduce how to use the Java programming language to implement the document conversion function of the CMS system and provide relevant code examples.
1. Understand the document conversion process
Before we start writing code, we need to understand the basic process of document conversion. Usually, the document conversion process includes the following steps:
- Select the document to be converted: The user selects the document to be converted from the CMS system.
- Identify document format: Identify the format of the document based on its extension or other characteristics.
- Convert document: According to the format of the document, use the corresponding conversion tool to convert the document into the target format.
- Save the converted document: Save the converted document to the CMS system for use by users.
2. Choose the appropriate conversion tool
In Java, there are many open source conversion tools to choose from. Some common tools include Apache POI, iText, PDFBox, etc. These tools provide rich APIs and functions that can be used to convert various types of documents.
For example, the Apache POI library can be used to convert Microsoft Office documents (such as Word, Excel, and PowerPoint), while iText and PDFBox can be used to handle the conversion of PDF documents.
According to the requirements of the CMS system, select the appropriate tool and configure it accordingly according to the format of the document.
3. Writing Java Code Example
The following is a simple Java code example that shows how to use the Apache POI library to convert a Word document to PDF format:
import org.apache.poi.xwpf.usermodel.XWPFDocument; import org.apache.poi.xwpf.usermodel.XWPFWordExtractor; import com.itextpdf.text.Document; import com.itextpdf.text.Paragraph; import com.itextpdf.text.pdf.PdfWriter; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; public class DocumentConverter { public static void convertToPDF(String inputFilePath, String outputFilePath) { try { // 读取Word文档 FileInputStream fis = new FileInputStream(new File(inputFilePath)); XWPFDocument document = new XWPFDocument(fis); // 提取文本内容 XWPFWordExtractor extractor = new XWPFWordExtractor(document); String text = extractor.getText(); // 创建PDF文档 Document pdfDoc = new Document(); PdfWriter.getInstance(pdfDoc, new FileOutputStream(outputFilePath)); pdfDoc.open(); // 添加文本到PDF文档 Paragraph paragraph = new Paragraph(text); pdfDoc.add(paragraph); // 关闭文档 pdfDoc.close(); fis.close(); System.out.println("转换成功!"); } catch (Exception e) { e.printStackTrace(); } } public static void main(String[] args) { String inputFilePath = "input.docx"; String outputFilePath = "output.pdf"; convertToPDF(inputFilePath, outputFilePath); } }
In In the above example, we first read the Word document through FileInputStream, and then use XWPFWordExtractor to extract the text content. Next, we create a PDF document, add text content to the PDF, and finally save the PDF to the specified output path.
You can further adjust the code according to your own needs and the requirements of the CMS system to adapt to different document conversion needs.
Conclusion
By using the Java programming language and appropriate conversion tools, we can easily implement the document conversion function of the CMS system. In this article, we learned the basic process of document conversion and provided a simple code example to help you get started. I hope this article can be helpful to you, and I wish you more success in development!
The above is the detailed content of How to use Java to implement the document conversion function of CMS system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




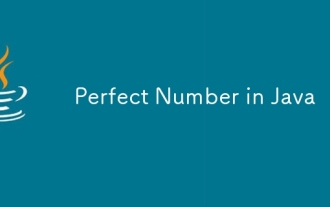
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
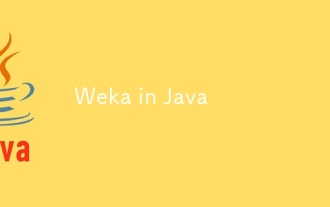
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
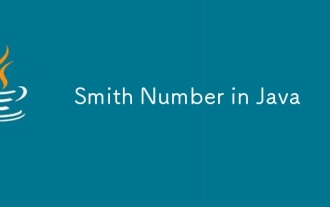
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
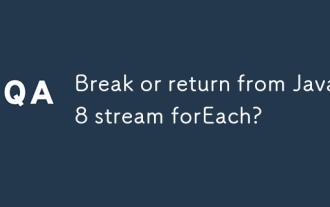
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
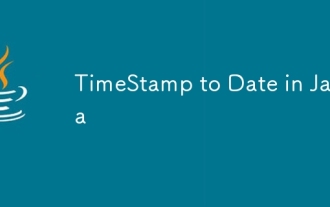
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
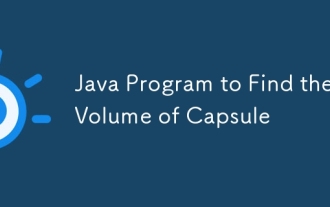
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
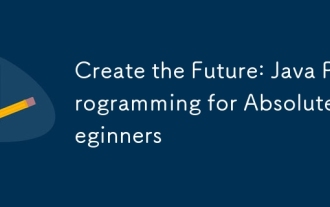
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
