


Workerman network programming practice: building a reliable real-time data synchronization system
Workerman Network Programming Practice: Building a Reliable Instant Data Synchronization System
With the popularity of the Internet and mobile devices, instant communication has become more and more important. Realizing instant messaging and data synchronization between different devices and platforms has become a common need among developers. In this article, we will explore how to build a reliable real-time data synchronization system using the Workerman network programming framework.
- Introduction to Workerman
Workerman is a high-performance event-driven programming framework based on PHP, which can quickly develop network applications. It uses non-blocking I/O and multi-process architecture, and supports TCP, UDP, WebSocket and other protocols. Workerman's high performance and scalability make it ideal for building real-time communication applications. - Installation and Configuration
First, we need to install Workerman. Workerman can be installed through composer through the command line:
composer require workerman/workerman
After the installation is completed, we can initialize Workerman through the following code:
<?php require_once __DIR__ . '/vendor/autoload.php'; use WorkermanWorker; $worker = new Worker(); $worker->listen('tcp://0.0.0.0:2345'); $worker->onMessage = function ($connection, $data) { // 这里处理收到的消息 }; Worker::runAll();
The above code creates a Worker object and listens at 2345 TCP connection on the port. Messages from the client are processed through the onMessage callback function. We can implement our own business logic in the callback function.
- Data synchronization system design
In order to build a reliable real-time data synchronization system, we need the following components:
- Database: used to store data.
- Cache system: used to cache data and improve reading and writing speed.
- Communication server: Responsible for real-time data synchronization between the client and the server.
- Client library: Provides developers with a convenient interface for data synchronization on the client side.
- Code Example
Below we take a simple chat application as an example to demonstrate how to use Workerman to build an instant data synchronization system.
Server code:
<?php require_once __DIR__ . '/vendor/autoload.php'; use WorkermanWorker; $worker = new Worker(); $worker->listen('websocket://0.0.0.0:8000'); $worker->onMessage = function ($connection, $data) { // 处理收到的消息 $data = json_decode($data, true); // 存储消息到数据库 saveMessageToDatabase($data); // 缓存消息 cacheMessage($data); // 向所有客户端广播消息 broadcastMessage($data); }; $worker->onClose = function ($connection) { // 处理客户端断开连接 removeClient($connection); }; function saveMessageToDatabase($data) { // 将消息存储到数据库中 } function cacheMessage($data) { // 缓存消息 } function broadcastMessage($data) { // 向所有客户端广播消息 } function removeClient($connection) { // 处理客户端断开连接 } Worker::runAll();
Client code:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Chat</title> </head> <body> <input type="text" id="message" placeholder="输入消息"> <button onclick="sendMessage()">发送</button> <script src="http://localhost:8000/socket.io/socket.io.js"></script> <script> var socket = io('http://localhost:8000'); socket.on('connect', function() { console.log('Connected to server'); }); socket.on('message', function(data) { console.log('Received message:', data); }); function sendMessage() { var message = document.getElementById('message').value; socket.emit('message', message); } </script> </body> </html>
The above code communicates through the Websocket protocol. The server uses the WebSocket class provided by Workerman to create a Websocket server, and the client uses the socket.io library to communicate with the server.
- Summary
Through the introduction of this article, we have learned how to use the Workerman network programming framework to build a reliable real-time data synchronization system. Using Workerman, we can easily create high-performance web applications. I hope this article will be helpful to you and stimulate your interest in network programming.
The above is the detailed content of Workerman network programming practice: building a reliable real-time data synchronization system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


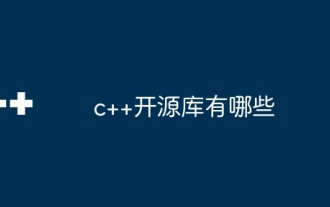
C++ provides a rich set of open source libraries covering the following functions: data structures and algorithms (Standard Template Library) multi-threading, regular expressions (Boost) linear algebra (Eigen) graphical user interface (Qt) computer vision (OpenCV) machine learning (TensorFlow) Encryption (OpenSSL) Data compression (zlib) Network programming (libcurl) Database management (sqlite3)

The C++ standard library provides functions to handle DNS queries in network programming: gethostbyname(): Find host information based on the host name. gethostbyaddr(): Find host information based on IP address. dns_lookup(): Asynchronously resolves DNS.
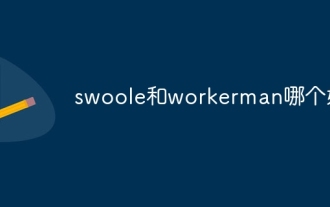
Swoole and Workerman are both high-performance PHP server frameworks. Known for its asynchronous processing, excellent performance, and scalability, Swoole is suitable for projects that need to handle a large number of concurrent requests and high throughput. Workerman offers the flexibility of both asynchronous and synchronous modes, with an intuitive API that is better suited for ease of use and projects that handle lower concurrency volumes.

Commonly used protocols in Java network programming include: TCP/IP: used for reliable data transmission and connection management. HTTP: used for web data transmission. HTTPS: A secure version of HTTP that uses encryption to transmit data. UDP: For fast but unstable data transfer. JDBC: used to interact with relational databases.
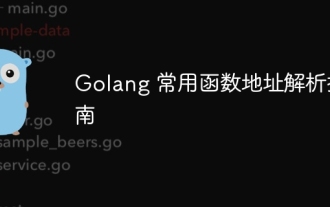
The key functions for parsing addresses in the Go language include: net.ParseIP(): Parse IPv4 or IPv6 addresses. net.ParseCIDR(): Parse CIDR tags. net.ResolveIPAddr(): Resolve hostname or IP address into IP address. net.ResolveTCPAddr(): Resolve host names and ports into TCP addresses. net.ResolveUDPAddr(): Resolve host name and port into UDP address.
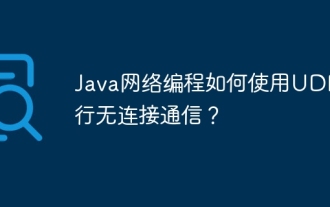
UDP (User Datagram Protocol) is a lightweight connectionless network protocol commonly used in time-sensitive applications. It allows applications to send and receive data without establishing a TCP connection. Sample Java code can be used to create a UDP server and client, with the server listening for incoming datagrams and responding, and the client sending messages and receiving responses. This code can be used to build real-world use cases such as chat applications or data collection systems.
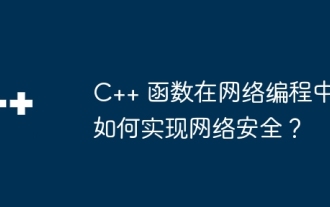
C++ functions can achieve network security in network programming. Methods include: 1. Using encryption algorithms (openssl) to encrypt communication; 2. Using digital signatures (cryptopp) to verify data integrity and sender identity; 3. Defending against cross-site scripting attacks ( htmlcxx) to filter and sanitize user input.

Java entry-to-practice guide: including introduction to basic syntax (variables, operators, control flow, objects, classes, methods, inheritance, polymorphism, encapsulation), core Java class libraries (exception handling, collections, generics, input/output streams , network programming, date and time API), practical cases (calculator application, including code examples).
