


PHP and Manticore Search development: implementing a highly customizable search interface
PHP and Manticore Search Development: Implementing a Highly Customizable Search Interface
Introduction:
In Web development, search function is a very important and common requirement. In order to improve search performance and user experience, we need to use a highly customizable search engine. In this article, I will describe how to develop a powerful and highly customizable search interface using PHP and Manticore Search.
Manticore Search is an upgraded version based on the open source search engine Sphinx, which provides higher performance and richer features, such as full-text search, distributed indexing and flexible customization options. As a commonly used web development language, PHP can be seamlessly integrated with Manticore Search to achieve flexible and customizable search functions.
Step 1: Install Manticore Search
First, we need to install Manticore Search on the server. You can download the installation package through the official website (https://manticoresearch.com/) and install and configure it according to the official documents. After the installation is complete, we can start the Manticore Search service through the terminal command line.
Step 2: Create an index
Before using Manticore Search, we need to create an index to store our data. Index creation can be done using the command line tools provided by Manticore Search or using PHP code. Here is an example of creating an index using PHP code:
<?php require_once 'vendor/autoload.php'; use ManticoresearchDocumentsBuilder; $index = 'my_index'; $client = new ManticoresearchClientClient(); $client->setHost('localhost'); $client->setPort(9308); $builder = new DocumentsBuilder($client); $builder->index($index); $data = [ [ 'id' => 1, 'title' => 'Manticore Search', 'content' => 'Manticore Search is a powerful search engine', ], [ 'id' => 2, 'title' => 'PHP', 'content' => 'PHP is a popular programming language for web development', ], // ... more data items ]; foreach ($data as $item) { $builder->create([ 'id' => $item['id'], 'title' => $item['title'], 'content' => $item['content'], ]); } $builder->commit();
With the above example code, we can create an index named my_index
and add some data to it.
Step 3: Implement the search function
After creating the index, we can use PHP code to call the API of Manticore Search to implement the search function. The following is an example of implementing a basic search function:
<?php require_once 'vendor/autoload.php'; use ManticoresearchClientClient; $index = 'my_index'; $query = 'Manticore Search'; $client = new Client(); $client->setHost('localhost'); $client->setPort(9308); $search = $client->search($index); $result = $search->search($query); foreach ($result['hits']['hits'] as $hit) { echo 'ID: ' . $hit['_id'] . '<br>'; echo 'Title: ' . $hit['_source']['title'] . '<br>'; echo 'Content: ' . $hit['_source']['content'] . '<br>'; echo '<br>'; }
Through the above example code, we can use the search
method to search and obtain search results. We can then iterate through the search results and output relevant information.
Step 4: Customize the search interface
In order to provide a better user experience, we can customize the style and functions of the search interface as needed. The following is an example of using HTML and CSS to customize the search interface:
<!DOCTYPE html> <html> <head> <style> .search-box { width: 300px; margin-bottom: 10px; } .search-results { list-style: none; padding: 0; } .search-results li { padding: 10px; background-color: #f5f5f5; margin-bottom: 5px; } </style> </head> <body> <div class="search-box"> <input type="text" id="search-input" placeholder="Search..."> <input type="button" id="search-button" value="Search"> </div> <ul class="search-results"></ul> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { $('#search-button').click(function() { var query = $('#search-input').val(); $.ajax({ url: 'search.php', type: 'GET', data: {query: query}, success: function(response) { $('.search-results').empty(); response.forEach(function(result) { var li = $('<li></li>'); li.text(result.title); $('.search-results').append(li); }); } }); }); }); </script> </body> </html>
The above sample code demonstrates a simple search interface where users can enter keywords and click the search button. The search function uses the jQuery library to asynchronously load search results and display the results on the page.
Conclusion:
Through the combination of PHP and Manticore Search, we can achieve a highly customizable search interface. In this article, we learned how to install Manticore Search, create an index, implement basic search functions, and how to customize the style and functionality of the search interface through HTML and CSS. Using these methods and sample codes, we can customize a suitable search interface according to project needs to improve user experience and search results.
Reference link:
- https://manticoresearch.com/
- https://github.com/manticoresoftware/manticoresearch-php
The above is the detailed content of PHP and Manticore Search development: implementing a highly customizable search interface. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
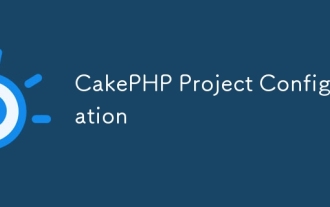
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
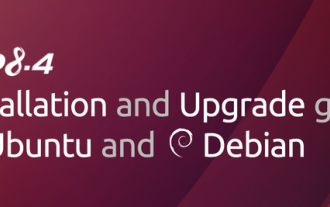
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
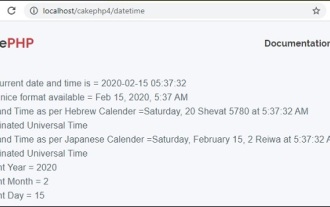
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
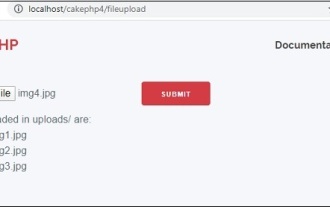
To work on file upload we are going to use the form helper. Here, is an example for file upload.
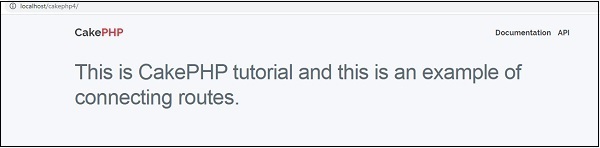
In this chapter, we are going to learn the following topics related to routing ?
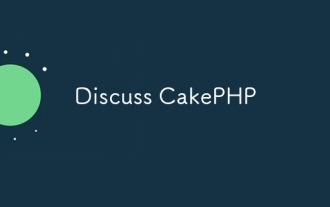
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
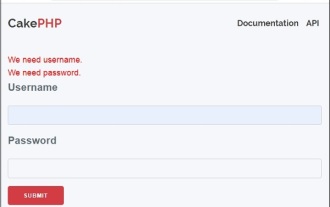
Validator can be created by adding the following two lines in the controller.
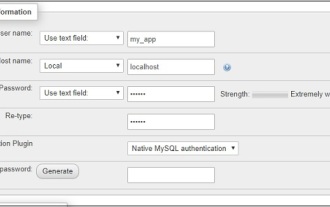
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
