


How to use Python to implement the user access analysis function of CMS system
How to use Python to implement the user access analysis function of the CMS system
CMS system (Content Management System) is a software system used to manage website content. In the process of building and maintaining a website, understanding and analyzing user access behavior is crucial to improving user experience and website effectiveness. As a powerful programming language, Python can help us implement the user access analysis function of the CMS system.
This article will introduce how to use Python to implement the user access analysis function of the CMS system, and attach code examples.
1. Data collection and processing
The first step is to collect and process user access data. User access data usually includes user ID, access time, accessed pages and other information. We can use Python's web framework (such as Flask or Django) to build a CMS system and embed the access record code in the corresponding page.
Sample code:
from flask import Flask, request from datetime import datetime app = Flask(__name__) @app.route('/') def home(): user_id = request.args.get('user_id') page = request.url access_time = datetime.now().strftime("%Y-%m-%d %H:%M:%S") # 将用户访问记录存储到数据库或文件中 record = f"{user_id},{access_time},{page} " with open('access_log.txt', 'a') as f: f.write(record) return 'Welcome to CMS home page!' if __name__ == '__main__': app.run()
In the above example, we used the Flask framework to build the homepage of a simple CMS system. When a user visits the homepage, we obtain the user ID, access time, visited page and other information, and record it into a text file named access_log.txt.
2. User access analysis
The next step is the analysis of user access data. We can use Python's data analysis library (such as pandas) to process and analyze the collected access data.
Sample code:
import pandas as pd df = pd.read_csv('access_log.txt', names=['user_id', 'access_time', 'page']) # 统计每个用户的访问次数 visit_count = df['user_id'].value_counts() # 统计独立访问用户数 unique_users = df['user_id'].nunique() # 统计每个页面的访问次数 page_count = df['page'].value_counts() print("用户访问统计:") print(visit_count) print(" 独立访问用户数:", unique_users) print(" 页面访问统计:") print(page_count)
In the above example, we used the pandas library to read the access_log.txt file and collect statistics on user access data. We count the number of visits per user, the number of unique visitors, and the number of visits to each page.
3. Data visualization
The last step is to visualize the user access data. We can use Python's data visualization library (such as matplotlib or seaborn) to display statistical results in charts.
Sample code:
import matplotlib.pyplot as plt # 绘制用户访问次数的柱状图 plt.figure(figsize=(10, 6)) visit_count.plot(kind='bar', rot=0) plt.xlabel('User ID') plt.ylabel('Visit Count') plt.title('User Visit Count') plt.show() # 绘制页面访问次数的饼图 plt.figure(figsize=(10, 6)) page_count.plot(kind='pie', autopct='%1.1f%%') plt.ylabel('') plt.title('Page Visit Count') plt.show()
In the above example, we used the matplotlib library to draw a histogram of the number of user visits and a pie chart of the number of page visits.
Through the above steps, we can realize the user access analysis function of the CMS system. By collecting and processing user access data, and conducting data analysis and visualization, we can better understand user access behavior and make further optimization and improvements based on the analysis results.
Summary:
This article introduces how to use Python to implement the user access analysis function of the CMS system. We first collect and process user access data, then use the data analysis library to perform data analysis, and finally use the data visualization library to display the results in charts. Through these steps, we can better understand user access behavior and make further optimization and improvements based on the analysis results.
The above is the detailed content of How to use Python to implement the user access analysis function of CMS system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


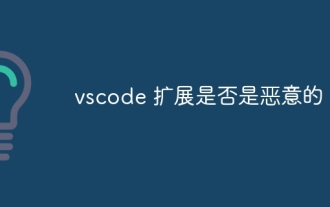
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
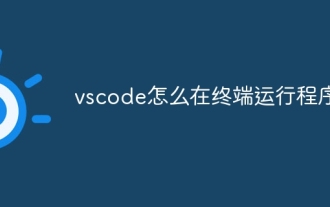
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
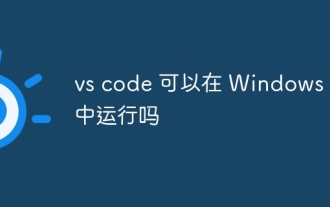
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
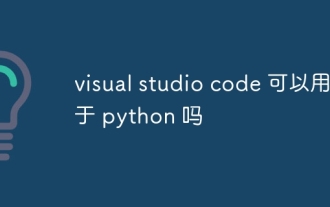
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
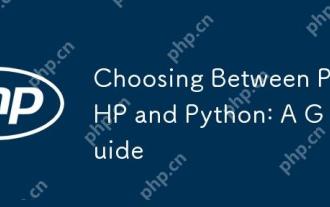
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
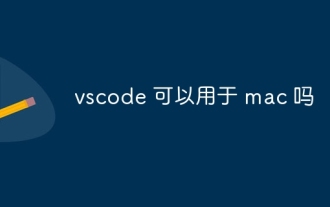
VS Code is available on Mac. It has powerful extensions, Git integration, terminal and debugger, and also offers a wealth of setup options. However, for particularly large projects or highly professional development, VS Code may have performance or functional limitations.
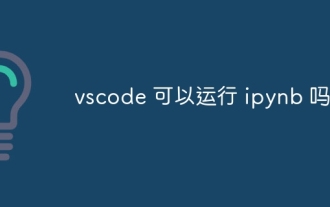
The key to running Jupyter Notebook in VS Code is to ensure that the Python environment is properly configured, understand that the code execution order is consistent with the cell order, and be aware of large files or external libraries that may affect performance. The code completion and debugging functions provided by VS Code can greatly improve coding efficiency and reduce errors.
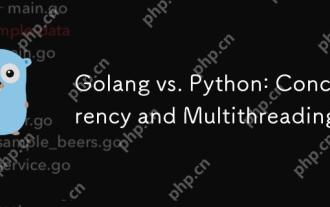
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
