


In-depth analysis of the multi-process model of swoole development function
In-depth analysis of the multi-process model of Swoole development function
Introduction:
In high concurrency situations, the traditional single-process and single-thread model often cannot meet the needs, so the multi-process model has become a a common solution. Swoole is a multi-process-based PHP extension that provides a simple, easy-to-use, efficient and stable multi-process development framework. This article will deeply explore the implementation principles of the Swoole multi-process model and analyze it with code examples.
- Introduction to Swoole multi-process model
In Swoole, we can create sub-processes through theswoole_process
class to implement the multi-process model. Each child process has an independent memory space and can perform its own tasks. The main process is responsible for managing the life cycle of the child process, distributing tasks, and handling the exit of the child process. Child processes can exchange data via IPC (inter-process communication) or shared memory. - Advantages of the Swoole multi-process model
Compared with the traditional model, the Swoole multi-process model has the following advantages:
(1) Share the pressure of the main process: child processes can accept and process requests , reduce the burden on the main process and improve the concurrency capability of the system.
(2) Fast response: Swoole's multi-process model can handle multiple requests at the same time, improving the system's response speed.
(3) Better utilization of hardware resources: On a multi-core CPU machine, each sub-process can be bound to a different CPU core to improve the operating efficiency of the system. - Implementation of Swoole multi-process model
The following is a sample code that uses Swoole to implement a multi-process model:
<?php $worker_num = 4; // 创建 4 个子进程 $workers = []; // 创建子进程 for ($i = 0; $i < $worker_num; $i++) { $process = new swoole_process('process_callback'); $pid = $process->start(); $workers[$pid] = $process; // 将子进程对象保存起来 } // 子进程逻辑处理函数 function process_callback(swoole_process $worker) { // 子进程逻辑代码 // ... } // 主进程监听子进程退出事件 foreach ($workers as $pid => $process) { swoole_event_add($process->pipe, function ($pipe) use ($process) { $data = $process->read(); // 读取子进程发送过来的数据 // 对数据进行处理 // ... }); } // 主进程等待子进程退出 swoole_process::wait();
In the above code, we first create the specified number of Subprocesses, then create these subprocesses through the swoole_process
class, and save the subprocess objects. Each child process will execute the logic code of the process_callback
function.
Next, the main process listens to the pipe events of the sub-process through the swoole_event_add
method. When the sub-process has data written to the pipe, the main process will receive the notification and read it in the callback function. Get the data sent by the child process. The main process can perform corresponding processing according to the content of the data.
Finally, the main process waits for all child processes to exit through the swoole_process::wait()
method.
- Summary
In this article, we have an in-depth exploration of the implementation principles of the Swoole multi-process model and give code examples. By using Swoole's multi-process model, we can effectively improve the concurrency and response speed of the system, make better use of hardware resources, and provide an effective solution for high-concurrency scenarios.
It should be noted that when using Swoole's multi-process model, we need to fully understand the mechanism of inter-process communication to avoid data conflicts or competition. In addition, you also need to pay attention to controlling the number of child processes to avoid wasting system resources caused by too many child processes.
I hope this article will be helpful in understanding the Swoole multi-process model and provide readers with a reference for better developing high-concurrency and high-performance systems.
The above is the detailed content of In-depth analysis of the multi-process model of swoole development function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
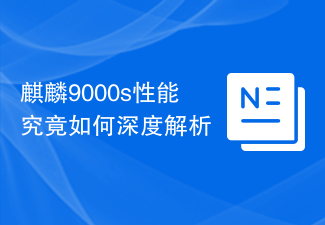
An in-depth analysis of the performance of Kirin 9000s. As competition in the smartphone market becomes increasingly fierce, mobile phone manufacturers have launched new products with unique characteristics. Among them, Huawei, as the leader in the Chinese mobile phone market, has been committed to making breakthroughs in the chip field. Recently, Huawei released the Kirin 9000s chip, which attracted widespread attention. This chip is hailed as one of Huawei's most powerful chips at present, so what is its performance? Below we will conduct an in-depth analysis of Kirin 9000s. First of all, it is worth mentioning that Kirin 9000s uses 5
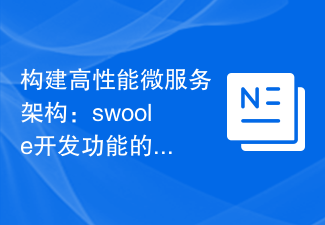
Building a high-performance microservice architecture: Best practices for Swoole development functions With the rapid development of the Internet and mobile Internet, high-performance microservice architecture has become a need for many enterprises. As a high-performance PHP extension, Swoole can provide asynchronous, coroutine and other functions, making it the best choice for building high-performance microservice architecture. This article will introduce how to use Swoole to develop a high-performance microservice architecture and provide corresponding code examples. Install and configure the Swoole extension. First, you need to install Swool on the server.

The implementation principle of message queue and asynchronous communication of Swoole development function With the rapid development of Internet technology, developers' needs for high performance and high concurrency are becoming more and more urgent. As a development framework, Swoole is favored by more and more developers because of its excellent performance and rich functions. This article will introduce the implementation principles of message queue and asynchronous communication in Swoole, and explain it in detail with code examples. First, let's first understand what message queue and asynchronous communication are. Message queue is a decoupled communication mechanism that can
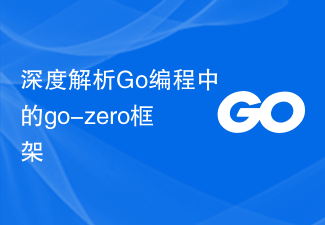
With the continuous development of the Internet, the Go language is gradually becoming more and more popular among developers. If you are a Go developer, then you will definitely be familiar with the go-zero framework. It is a lightweight framework for microservices and is recognized by more and more developers. Today, this article will provide an in-depth analysis of the go-zero framework. 1. Introduction to go-zero framework go-zero is a high-performance and simple microservice framework. It is developed on the basis of Go language. The framework mainly revolves around microservice architecture
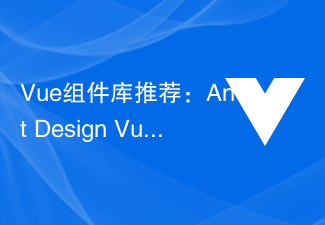
Vue component library recommendation: AntDesignVue in-depth analysis introduction Vue.js has become one of the most popular JavaScript frameworks today. It is easy to learn, efficient and fast, allowing developers to quickly build high-quality web applications. However, with the popularity of Vue.js, many excellent Vue component libraries have emerged. Among them, AntDesignVue is undoubtedly one of the most popular. AntDesignVue is a software created by the Alibaba team
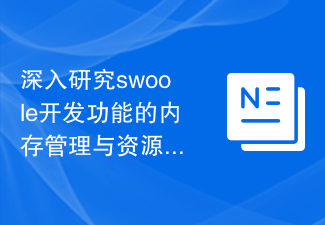
In-depth study of memory management and resource optimization of swoole development functions. With the rapid development of the Internet, the demand for high concurrency and low latency is becoming more and more urgent. As a high-performance PHP network communication engine, Swoole provides developers with more efficient solutions. When using Swoole to develop functions, memory management and resource optimization are key issues that need to be considered. This article takes a deep dive into how to effectively manage memory and optimize resources, along with corresponding code examples. 1. Memory management to avoid memory leaks and memory leaks
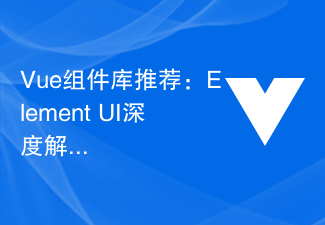
Recommended Vue component library: In-depth analysis of ElementUI Introduction: In Vue development, using component libraries can greatly improve development efficiency and reduce the amount of repetitive work. ElementUI, as an excellent Vue component library, is widely used in various projects. This article will provide an in-depth analysis of ElementUI and provide developers with detailed usage instructions and specific code examples. Introduction to ElementUI ElementUI is a desktop component library based on Vue.js, developed by
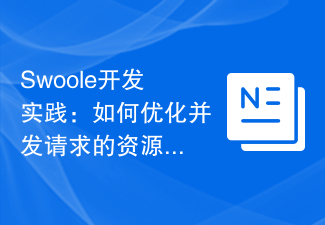
Swoole is a high-performance network communication library based on PHP for developing asynchronous and concurrent network applications. Because of its high-performance characteristics, Swoole has become one of the preferred technologies for many Internet companies. In actual development, how to optimize the resource consumption of concurrent requests has become a challenge that many engineers must face. The following will be combined with code examples to introduce how to use Swoole to optimize the resource consumption of concurrent requests. 1. Use coroutines to improve concurrency. Swoole provides powerful coroutine functions.
