


How to handle PHP database connection timeout errors and generate corresponding error messages
How to handle PHP database connection timeout errors and generate corresponding error messages
During the PHP development process, database connection timeout errors are often encountered. This error is usually caused by database connection problems or when performing database operations takes a long time. In order to better handle this type of error and provide users with corresponding error information, we can handle it through the following steps.
Step 1: Set the database connection timeout
When connecting to the database in PHP, you can use the methods provided by extensions such as mysqli
or PDO
to set the connection timeout. The following is an example using the mysqli
extension:
$db_host = "localhost"; //数据库主机 $db_username = "root"; //数据库用户名 $db_password = "password"; //数据库密码 $db_name = "mydatabase"; //数据库名 $connection_timeout = 10; //连接超时时间(单位:秒) $mysqli = new mysqli($db_host, $db_username, $db_password, $db_name); $mysqli->options(MYSQLI_OPT_CONNECT_TIMEOUT, $connection_timeout);
In the above code, the mysqli->options
method sets the connection timeout to 10 seconds.
Step 2: Capture the connection timeout exception
Next, we need to capture the connection timeout exception and generate the corresponding error message. Exceptions can be caught using the try-catch
statement. The following is a sample code:
try { $mysqli = new mysqli($db_host, $db_username, $db_password, $db_name); $mysqli->options(MYSQLI_OPT_CONNECT_TIMEOUT, $connection_timeout); } catch (mysqli_sql_exception $e) { $error_message = "数据库连接超时:" . $e->getMessage(); //生成错误日志,发送邮件等操作 }
In the above code, the code in the try
block will try to connect to the database, and if the connection times out, a mysqli_sql_exception
exception will be thrown. In the catch
block, we can obtain the exception object $e
and generate the corresponding error message.
Step 3: Handling connection timeout errors
After catching the connection timeout exception, we can handle this type of error according to actual needs. Generally, we need to generate error logs, send emails, or display appropriate error messages to users. The following is a simple sample code:
try { $mysqli = new mysqli($db_host, $db_username, $db_password, $db_name); $mysqli->options(MYSQLI_OPT_CONNECT_TIMEOUT, $connection_timeout); } catch (mysqli_sql_exception $e) { $error_message = "数据库连接超时:" . $e->getMessage(); //生成错误日志 error_log($error_message, 3, "error.log"); //发送邮件 $to = "admin@example.com"; $subject = "数据库连接超时"; $message = $error_message; $headers = "From: webmaster@example.com"; mail($to, $subject, $message, $headers); //显示错误信息给用户 echo "很抱歉,数据库连接超时,请稍后再试!"; }
In the above sample code, we use the error_log
function to write error information to the error log file, and the mail
function to write the error message to the error log file. The information is sent to the administrator and the error message is displayed to the user using the echo
statement.
Through the above steps, we can better handle PHP database connection timeout errors and generate corresponding error messages. This can improve the user experience and make it easier for us to troubleshoot and fix problems.
The above is the detailed content of How to handle PHP database connection timeout errors and generate corresponding error messages. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


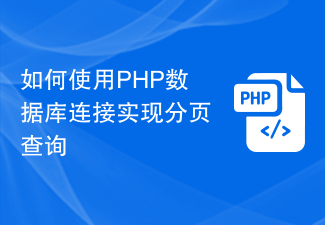
How to use PHP database connection to implement paging query. When developing web applications, it often involves the need to query the database and perform paging display. As a commonly used server-side scripting language, PHP has powerful database connection functions and can easily implement paging queries. This article will introduce in detail how to use PHP database connection to implement paging query, and attach corresponding code examples. Prepare the database Before we start, we need to prepare a database containing the data to be queried. Here we take the MySQL database as an example,
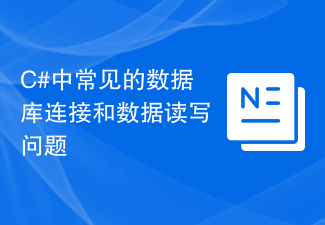
Common database connection and data reading and writing problems in C# require specific code examples. In C# development, database connection and data reading and writing are frequently encountered problems. Correct handling of these problems is the key to ensuring code quality and performance. This article will introduce some common database connection and data reading and writing problems, and provide specific code examples to help readers better understand and solve these problems. Database connection issues 1.1 Connection string errors When connecting to the database, a common error is that the connection string is incorrect. The connection string contains the connection to the database
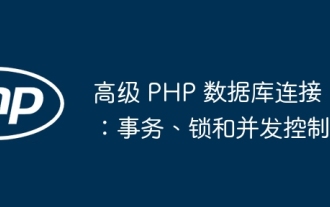
Advanced PHP database connections involve transactions, locks, and concurrency control to ensure data integrity and avoid errors. A transaction is an atomic unit of a set of operations, managed through the beginTransaction(), commit(), and rollback() methods. Locks prevent simultaneous access to data via PDO::LOCK_SHARED and PDO::LOCK_EXCLUSIVE. Concurrency control coordinates access to multiple transactions through MySQL isolation levels (read uncommitted, read committed, repeatable read, serialized). In practical applications, transactions, locks and concurrency control are used for product inventory management on shopping websites to ensure data integrity and avoid inventory problems.
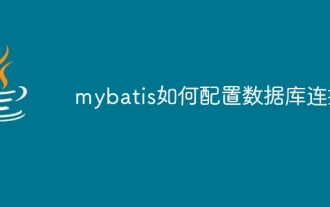
How to configure database connection in mybatis: 1. Specify the data source; 2. Configure the transaction manager; 3. Configure the type processor and mapper; 4. Use environment elements; 5. Configure aliases. Detailed introduction: 1. Specify the data source. In the "mybatis-config.xml" file, you need to configure the data source. The data source is an interface, which provides a database connection; 2. Configure the transaction manager to ensure the normality of database transactions. For processing, you also need to configure the transaction manager; 3. Configure the type processor and mapper, etc.
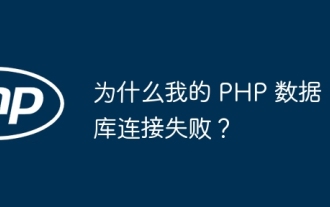
Reasons for a PHP database connection failure include: the database server is not running, incorrect hostname or port, incorrect database credentials, or lack of appropriate permissions. Solutions include: starting the server, checking the hostname and port, verifying credentials, modifying permissions, and adjusting firewall settings.
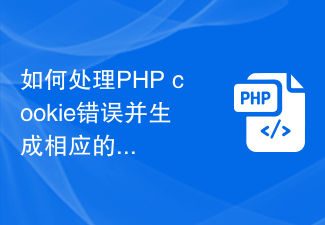
How to handle PHP cookie errors and generate corresponding error messages. In the PHP development process, using cookies is a common way to store and obtain user-related information. However, sometimes we may encounter some problems, such as incorrect cookie values or failure to generate cookies. In this case, we need to handle errors appropriately and generate corresponding error messages to ensure that our program can run normally. Here are several common PHP cookie errors and how to deal with them,
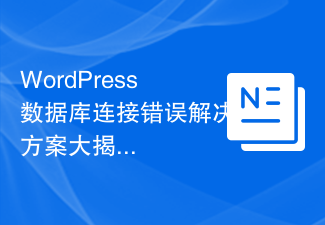
WordPress is currently one of the most popular website building platforms in the world, but during use, you sometimes encounter database connection errors. This kind of error will cause the website to be unable to be accessed normally, causing trouble to the website administrator. This article will reveal how to solve WordPress database connection errors and provide specific code examples to help readers solve this problem more quickly. Problem Analysis WordPress database connection errors are usually caused by the following reasons: Incorrect database username or password data
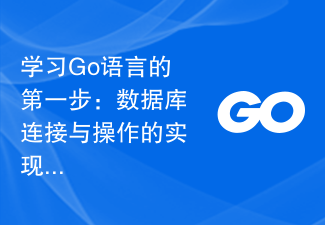
Learn Go language from scratch: How to implement database connection and operation, specific code examples are required 1. Introduction Go language is an open source programming language, developed by Google, and widely used to build high-performance, reliable server-side software . In Go language, using a database is a very common requirement. This article will introduce how to implement database connection and operation in Go language, and give specific code examples. 2. Choose the appropriate database driver. In the Go language, there are many third-party database drivers to choose from, such as My
