


In-depth interpretation: How to optimize the efficiency of PHP and regular expressions in processing collected data
In-depth interpretation: How to optimize the efficiency of PHP and regular expressions in processing collected data
Overview:
In the process of web crawlers and data collection, regular expressions are a commonly used tool. To extract the required data from web content. However, large-scale data collection operations may face efficiency issues. This article will introduce how to improve the efficiency of data collection by optimizing the use of PHP and regular expressions.
1. Data cleaning before using regular expressions
Before regular expression matching, some processing can be done on the original data to improve the efficiency of subsequent matching. The following are some commonly used data cleaning methods:
- Removing HTML tags:
When collecting web page content, it is often necessary to extract text information rather than web page tags. You can use PHP's strip_tags() function to remove HTML tags and reduce the matching content of regular expressions.
Sample code:
$html = "<div><p>Hello, World!</p></div>"; $text = strip_tags($html); echo $text; // 输出:Hello, World!
- Remove whitespace characters:
When matching regular expressions, whitespace characters will take up additional processing time. You can use PHP's trim() function to remove whitespace characters before and after a string to improve matching efficiency.
Sample code:
$string = " This is a test string. "; $string = trim($string); echo $string; // 输出:This is a test string.
- Encoding conversion:
Before regular expression matching, the encoding of the original data can be converted to an encoding suitable for matching to avoid Matching failure or garbled characters. Encoding conversion can be performed using PHP's iconv() function.
Sample code:
$string = "中文"; $string = iconv("UTF-8", "GB2312//IGNORE", $string); echo $string; // 输出:中文
2. Use appropriate regular expression patterns
The choice of regular expression patterns is crucial to improving efficiency. Here are some ways to optimize regular expressions:
- Use non-greedy mode:
The default mode of regular expressions is greedy mode, which matches as many characters as possible. But in practical applications, it is often necessary to match only the shortest string. Greedy mode can be changed to non-greedy mode using the "?" modifier.
Sample code:
$string = "123456"; preg_match("/d+?/", $string, $matches); print_r($matches); // 输出:Array([0] => 1)
- Using delimiters:
When writing regular expressions, you can use delimiters to enclose patterns. Commonly used delimiters include "/", "#", "~", etc. Using delimiters can improve the readability of regular expressions and reduce the use of escape characters.
Sample code:
$string = "Hello, World!"; preg_match("#Hello#", $string, $matches); print_r($matches); // 输出:Array([0] => Hello)
- Avoid using backtracking:
Backtracking in regular expressions means that when a match fails, the engine will try other possible matches. Under certain circumstances, backtracking can cause regular expressions to become less efficient. You can avoid using backtracking by writing regular expressions appropriately.
Sample code:
$string = "123abc"; preg_match("/d{3}[a-z]{3}/", $string, $matches); // 正确 print_r($matches); // 输出:Array([0] => 123abc) $string = "123ab"; preg_match("/d{3}[a-z]{3}/", $string, $matches); // 错误,会回溯 print_r($matches); // 输出:Array()
3. Use PHP functions to replace regular expressions
In some simple data processing scenarios, using PHP’s built-in string functions may be more efficient than regular expressions. Expressions are more efficient. The following are some commonly used string functions:
- strpos(): Find the first occurrence in a string.
- substr(): Intercept part of the string.
- str_replace(): Replace part of the string.
Sample code:
$string = "Hello, World!"; $pos = strpos($string, ","); // 查找逗号的位置 echo $pos; // 输出:6 $substring = substr($string, 0, 5); // 截取前五个字符 echo $substring; // 输出:Hello $newString = str_replace("Hello", "Hi", $string); // 替换字符串 echo $newString; // 输出:Hi, World!
Conclusion:
By optimizing PHP and regular expressions, we can improve the efficiency of data collection. Cleaning data before using regular expressions, choosing appropriate regular expression patterns, and using PHP's built-in string functions instead of regular expressions are all effective ways to optimize performance. In practical applications, it can be adjusted and optimized according to specific situations to achieve better efficiency and accuracy.
The above is the detailed content of In-depth interpretation: How to optimize the efficiency of PHP and regular expressions in processing collected data. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




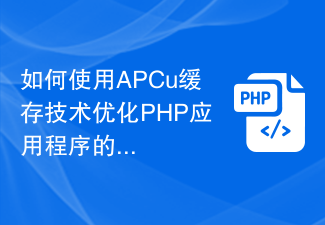
At present, PHP has become one of the most popular programming languages in Internet development, and the performance optimization of PHP programs has also become one of the most pressing issues. When handling large-scale concurrent requests, a delay of one second can have a huge impact on the user experience. Today, APCu (AlternativePHPCache) caching technology has become one of the important methods to optimize PHP application performance. This article will introduce how to use APCu caching technology to optimize the performance of PHP applications. 1. APC
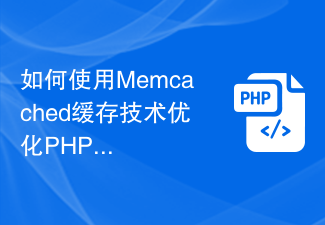
With the development of the Internet, PHP applications have become more and more common in the field of Internet applications. However, high concurrent access by PHP applications can lead to high CPU usage on the server, thus affecting the performance of the application. In order to optimize the performance of PHP applications, Memcached caching technology has become a good choice. This article will introduce how to use Memcached caching technology to optimize the CPU usage of PHP applications. Introduction to Memcached caching technology Memcached is a
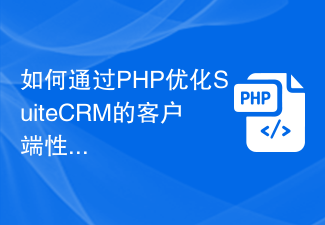
Overview of How to Optimize SuiteCRM's Client-Side Performance with PHP: SuiteCRM is a powerful open source customer relationship management (CRM) system, but performance issues can arise when handling large amounts of data and concurrent users. This article will introduce some methods to optimize SuiteCRM client performance through PHP programming techniques, and attach corresponding code examples. Using appropriate data queries and indexes Database queries are one of the core operations of a CRM system. In order to improve query performance, appropriate data query
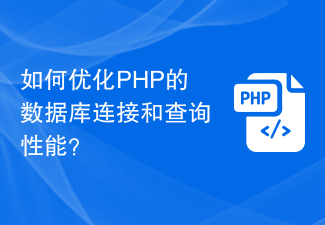
How to optimize PHP's database connection and query performance? The database is an indispensable part of web development, and PHP, as a widely used server-side scripting language, its connection to the database and query performance are crucial to the performance of the entire system. This article will introduce some tips and suggestions for optimizing PHP database connection and query performance. Use persistent connections: In PHP, a database connection is established every time a database query is executed. Persistent connections can reuse the same database connection in multiple queries, thereby reducing
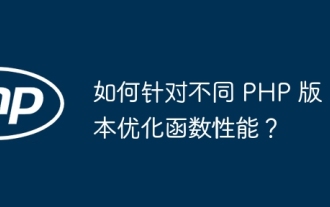
Methods to optimize function performance for different PHP versions include: using analysis tools to identify function bottlenecks; enabling opcode caching or using an external caching system; adding type annotations to improve performance; and selecting appropriate string concatenation and sorting algorithms according to the PHP version.
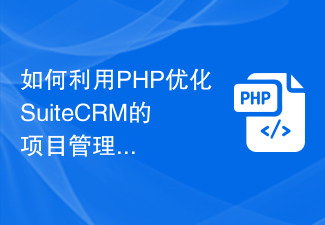
How to use PHP to optimize the project management functions of SuiteCRM SuiteCRM is a powerful open source customer relationship management (CRM) system that provides a wide range of functions and customizability. In terms of project management, SuiteCRM provides some basic functions, such as task assignment, progress tracking, and file sharing. However, sometimes we need to optimize project management capabilities based on specific business needs. In this article, we’ll cover how to leverage the PHP programming language to extend and optimize SuiteCRM’s
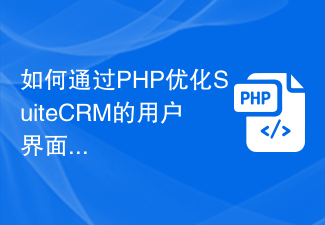
How to Optimize SuiteCRM’s User Interface with PHP SuiteCRM is a popular open source CRM (customer relationship management) software that provides powerful functionality and flexible customizability. However, when using SuiteCRM, you sometimes find that the user interface (UI) performs poorly or does not meet specific needs. At this time, we can optimize the user interface of SuiteCRM by using the PHP programming language to improve performance and meet specific needs. This article will introduce some optimizations for SuiteC
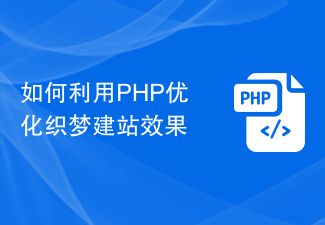
How to use PHP to optimize the effect of DreamWeaver's website building. In today's rise of the Internet, it is increasingly important to build an efficient and high-quality website. DedeCMS is a powerful website building system, but sometimes its default functions may not fully meet our needs. In this article, we will explore how to use PHP to optimize the effect of Dreamweaver website building, and provide some specific code examples. 1. Optimize website speed. Website speed is one of the important factors for user experience and SEO ranking. Website speed can be improved by optimizing PHP code.
