


Code generation for order tracking function in PHP inventory management system
Code generation for the order tracking function in the PHP inventory management system
Note: This article takes a simple inventory management system as an example to show how to use PHP code to implement the order tracking function.
Order tracking is one of the very important functions in the inventory management system. It can help us track the status of orders and update inventory in a timely manner. The following is a code example of an order tracking function based on PHP:
- First, create a table named "orders" in the database to store order information. The table structure is as follows:
CREATE TABLE `orders` ( `id` int(11) NOT NULL AUTO_INCREMENT, `order_number` varchar(50) NOT NULL, `status` enum('pending','processing','shipped','delivered','cancelled') NOT NULL, `created_at` datetime NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
- Create a class named "OrderTracking" in the PHP code to handle operations related to order tracking. The sample code is as follows:
class OrderTracking { private $db; public function __construct($db) { $this->db = $db; } // 创建新订单 public function createOrder($orderNumber) { $status = 'pending'; $createdAt = date('Y-m-d H:i:s', time()); $sql = "INSERT INTO orders (order_number, status, created_at) VALUES ('$orderNumber', '$status', '$createdAt')"; $this->db->query($sql); return $this->db->insert_id; } // 更新订单状态 public function updateOrderStatus($orderId, $status) { $sql = "UPDATE orders SET status = '$status' WHERE id = $orderId"; $this->db->query($sql); } // 获取订单状态 public function getOrderStatus($orderId) { $sql = "SELECT status FROM orders WHERE id = $orderId"; $result = $this->db->query($sql); $row = $result->fetch_assoc(); return $row['status']; } }
In the above code, we created an "OrderTracking" class, which contains three methods:
- The "createOrder" method is used to create new order and insert it into the database. The status of each new order defaults to "pending" and the creation time is the current time.
- The "updateOrderStatus" method is used to update the status of the order. The status of a specified order can be updated by passing in the order ID and new status.
- The "getOrderStatus" method is used to get the status of the specified order.
- When using the order tracking function, we need to first instantiate the "OrderTracking" class and pass in the database connection object. The sample code is as follows:
// 假设我们已经建立了数据库连接对象$db $orderTracking = new OrderTracking($db); // 创建新订单 $orderNumber = 'ORD-20210101-001'; $orderId = $orderTracking->createOrder($orderNumber); // 更新订单状态 $orderTracking->updateOrderStatus($orderId, 'processing'); // 获取订单状态 $status = $orderTracking->getOrderStatus($orderId); echo '订单状态:' . $status;
In the above sample code, we first create a new order and obtain the ID of the order. Then we update the order status to "processing". Finally, we use the "getOrderStatus" method to get the latest status of the order and output it.
Through the above code examples, we can see that it is very simple to use PHP to write the order tracking function in the inventory management system. By creating a class containing related methods and using database operation statements, we can easily implement functions such as order creation, status update, and status acquisition. This will greatly improve the efficiency and accuracy of your inventory management system.
The above is the detailed content of Code generation for order tracking function in PHP inventory management system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
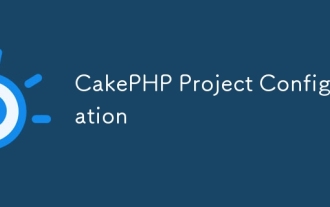
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
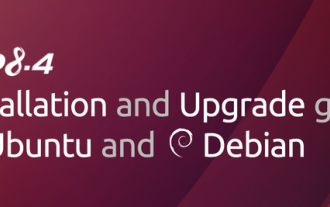
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
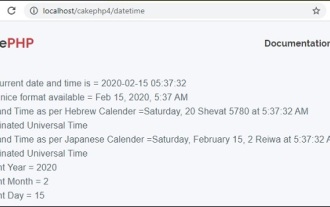
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
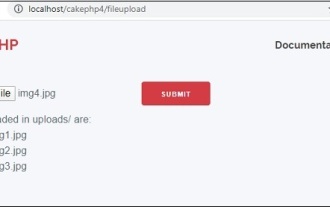
To work on file upload we are going to use the form helper. Here, is an example for file upload.
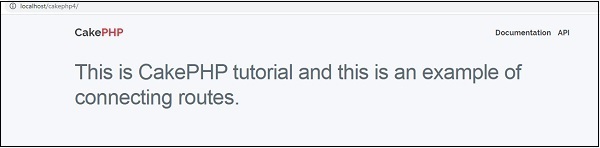
In this chapter, we are going to learn the following topics related to routing ?
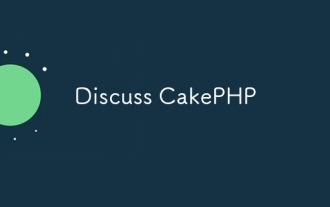
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
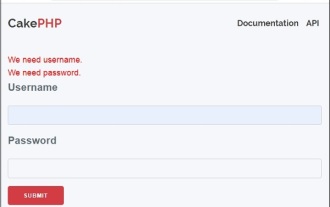
Validator can be created by adding the following two lines in the controller.
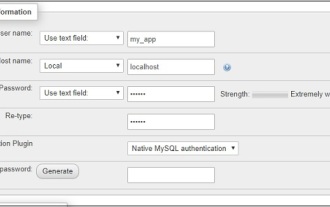
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
