


A practical guide for handling PHP code logic errors and generating corresponding error prompts
Practical guide for handling logic errors in PHP code and generating corresponding error prompts
Introduction:
When developing PHP applications, logic errors are unavoidable. These errors can cause application instability and functionality issues. However, by properly handling and generating corresponding error messages, we can locate and fix these problems faster. This article will introduce some practical guidelines to help you deal with logic errors in PHP code and generate corresponding error prompts.
1. Error reporting settings
In the PHP configuration file, there is an important setting called error reporting (error_reporting). By setting different error reporting levels, we can control whether PHP displays and reports errors. During development, it is recommended to set the error reporting level to the highest level so that logic errors can be discovered and resolved in a timely manner.
Sample code:
error_reporting(E_ALL); ini_set('display_errors', 1);
The above code sets the error reporting level to display all errors and displays the errors on the page. This ensures that problems are discovered in a timely manner and makes it easy to locate errors.
2. Error handling
When a logical error occurs in the PHP code, we should use the error handling function to capture and handle the error. This prevents errors from displaying directly on the page, providing a potential vulnerability for attackers.
Sample code:
set_error_handler(function ($errno, $errstr, $errfile, $errline) { // 处理错误逻辑 error_log("PHP Error: $errstr in $errfile on line $errline"); // 显示友好的错误提示 echo "Oops! Something went wrong. Please try again later."; });
The above code redirects all PHP errors to a custom error handling function. Inside the function, error information can be recorded or a customized error prompt can be generated based on the actual situation. This avoids exposing sensitive information to attackers.
3. Exception handling
In addition to error handling, PHP also supports exception handling mechanism. By throwing and catching exceptions, we can better control and handle logical errors in our code.
Sample code:
try { // 代码逻辑 } catch (Exception $e) { // 处理异常 error_log("PHP Exception: " . $e->getMessage() . " in " . $e->getFile() . " on line " . $e->getLine()); // 显示友好的错误提示 echo "Oops! Something went wrong. Please try again later."; }
The above code captures and redirects exceptions to a custom exception handling code block. When handling exceptions, we can record exception information or generate custom error prompts. This protects the security and stability of your application.
Conclusion:
Handling PHP code logic errors and generating corresponding error prompts is a very important part of the development process. By properly setting up error reporting, using error handling functions, and exception handling mechanisms, we can better protect the stability and security of our applications. Hopefully the practical guidance provided in this article will help you better handle logic errors in your PHP code.
Reference:
- PHP Manual: Error Reporting - https://www.php.net/manual/en/function.error-reporting.php
- PHP Manual: set_error_handler - https://www.php.net/manual/en/function.set-error-handler.php
- PHP Manual: Exceptions - https://www.php.net/ manual/en/language.exceptions.php
The above is the detailed content of A practical guide for handling PHP code logic errors and generating corresponding error prompts. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
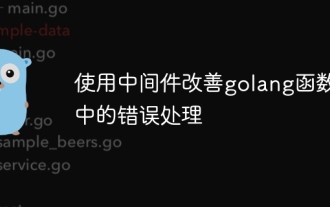
Use middleware to improve error handling in Go functions: Introducing the concept of middleware, which can intercept function calls and execute specific logic. Create error handling middleware that wraps error handling logic in a custom function. Use middleware to wrap handler functions so that error handling logic is performed before the function is called. Returns the appropriate error code based on the error type, улучшениеобработкиошибоквфункциях Goспомощьюпромежуточногопрограммногообеспечения.Оно позволяетнамсосредоточитьсянаобработкеошибо
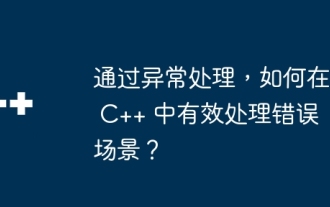
In C++, exception handling handles errors gracefully through try-catch blocks. Common exception types include runtime errors, logic errors, and out-of-bounds errors. Take file opening error handling as an example. When the program fails to open a file, it will throw an exception and print the error message and return the error code through the catch block, thereby handling the error without terminating the program. Exception handling provides advantages such as centralization of error handling, error propagation, and code robustness.
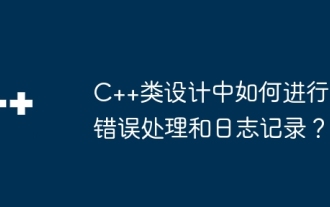
Error handling and logging in C++ class design include: Exception handling: catching and handling exceptions, using custom exception classes to provide specific error information. Error code: Use an integer or enumeration to represent the error condition and return it in the return value. Assertion: Verify pre- and post-conditions, and throw an exception if they are not met. C++ library logging: basic logging using std::cerr and std::clog. External logging libraries: Integrate third-party libraries for advanced features such as level filtering and log file rotation. Custom log class: Create your own log class, abstract the underlying mechanism, and provide a common interface to record different levels of information.
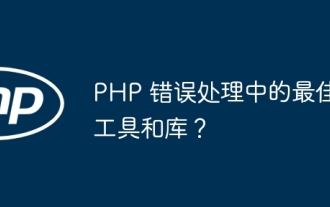
The best error handling tools and libraries in PHP include: Built-in methods: set_error_handler() and error_get_last() Third-party toolkits: Whoops (debugging and error formatting) Third-party services: Sentry (error reporting and monitoring) Third-party libraries: PHP-error-handler (custom error logging and stack traces) and Monolog (error logging handler)
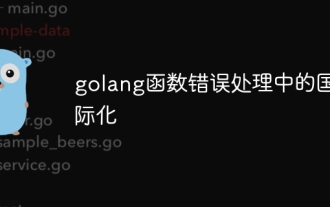
GoLang functions can perform error internationalization through the Wrapf and Errorf functions in the errors package, thereby creating localized error messages and appending them to other errors to form higher-level errors. By using the Wrapf function, you can internationalize low-level errors and append custom messages, such as "Error opening file %s".
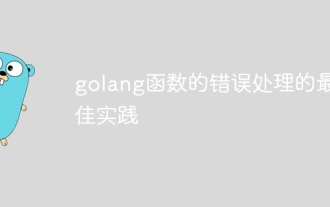
Best practices for error handling in Go include: using the error type, always returning an error, checking for errors, using multi-value returns, using sentinel errors, and using error wrappers. Practical example: In the HTTP request handler, if ReadDataFromDatabase returns an error, return a 500 error response.
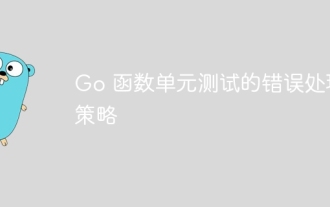
In Go function unit testing, there are two main strategies for error handling: 1. Represent the error as a specific value of the error type, which is used to assert the expected value; 2. Use channels to pass errors to the test function, which is suitable for testing concurrent code. In a practical case, the error value strategy is used to ensure that the function returns 0 for negative input.
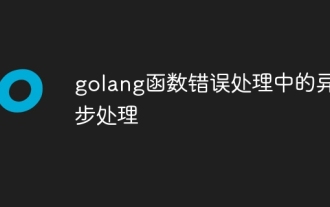
In Go functions, asynchronous error handling uses error channels to asynchronously pass errors from goroutines. The specific steps are as follows: Create an error channel. Start a goroutine to perform operations and send errors asynchronously. Use a select statement to receive errors from the channel. Handle errors asynchronously, such as printing or logging error messages. This approach improves the performance and scalability of concurrent code because error handling does not block the calling thread and execution can be canceled.
