


Core tuning methods to solve the bottleneck of Go language website access speed
Core tuning methods to solve the bottleneck of Go language website access speed
With the development of the Internet, website access speed has become more and more important to user experience. As an efficient and easy-to-write programming language, Go language has gradually been widely used in Web development. However, even websites written in Go may still face access speed bottlenecks. This article will introduce the core tuning methods to solve the bottleneck of Go language website access speed, and provide relevant code examples.
1. Use concurrent processing to improve speed
Go language inherently supports concurrency. By using goroutine and channel, efficient concurrent processing can be achieved. In website development, concurrent processing can be used to improve access speed. The following is a sample code using goroutine:
func handleRequest(w http.ResponseWriter, r *http.Request) { // 处理请求的逻辑 } func main() { http.HandleFunc("/", handleRequest) http.ListenAndServe(":8080", nil) }
By placing specific request processing logic in goroutine, multiple concurrent requests can be processed at the same time, thereby improving the response speed of the website.
2. Reduce memory allocation
In the Go language, memory allocation is a relatively slow operation. Frequent memory allocation may cause bottlenecks in website access speed. To improve access speed, memory allocation can be minimized. The following is a sample code for using sync.Pool to reuse objects:
type MyObject struct { // 对象的字段 } var myObjectPool = sync.Pool{ New: func() interface{} { return &MyObject{} }, } func handleRequest(w http.ResponseWriter, r *http.Request) { obj := myObjectPool.Get().(*MyObject) defer myObjectPool.Put(obj) // 处理请求的逻辑 } func main() { http.HandleFunc("/", handleRequest) http.ListenAndServe(":8080", nil) }
By using sync.Pool to cache objects that need to be frequently created and destroyed, the number of memory allocations can be reduced, thereby improving the access speed of the website. .
3. Optimize database access
In website development, database access is often a time-consuming operation. In order to improve the access speed of the website, the performance of database access can be optimized. The following is a sample code that uses a connection pool to optimize database access:
var db *sql.DB func handleRequest(w http.ResponseWriter, r *http.Request) { // 使用数据库连接执行操作 } func main() { var err error db, err = sql.Open("mysql", "username:password@tcp(localhost:3306)/dbname") if err != nil { log.Fatal(err) } defer db.Close() db.SetMaxIdleConns(10) db.SetMaxOpenConns(100) http.HandleFunc("/", handleRequest) http.ListenAndServe(":8080", nil) }
By setting the maximum number of idle connections and the maximum number of open connections in the connection pool, database connection resources can be reasonably utilized, thereby improving the performance of database access.
To sum up, by using concurrent processing, reducing memory allocation and optimizing database access, the bottleneck problem of Go language website access speed can be effectively solved. The sample code provided above is for reference only. The specific tuning method should be flexibly selected and adjusted according to the actual situation.
(Note: The above codes and methods are for reference only. The specific implementation may vary depending on the environment and needs. It is recommended to use it with caution in actual projects.)
The above is the detailed content of Core tuning methods to solve the bottleneck of Go language website access speed. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Many friends need a browser to download, but many friends who use edge report that the download speed is too slow, so how to improve the download speed? Let’s take a look at how to improve it. The download speed of the edge browser is slow: 1. Open the edge browser and enter the URL "about:flags". 2. After completion, enter "Developer Settings". 3. Pull down and check "Allow background tabs to be in low power mode" and "Allow limits on the rendering pipeline to improve battery life. This flag is locked to false by forcevsyncpaintbeat." 4. Continue to scroll down to "Network" and set "Enable TCP Quick Open" to "Always Enable".
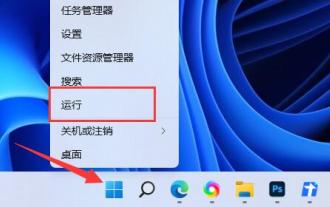
Some friends always feel that the Internet speed is very slow, and they find that their win11 download speed is limited. They don't know how to solve it. In fact, we only need to modify the Internet speed limit policy in the Group Policy Editor. The download speed of win11 is limited: The first step is to right-click the start menu and open "Run". The second step is to enter "gpedit.msc" and click "OK" to open the group policy. Step 3: Expand "Administrative Templates" under "Computer Configuration" Step 4: Click "Network" on the left, double-click "QoS Packet Scheduler" on the right Step 5: Check "Enabled" and set the bandwidth limit below Change it to "0" and finally click "OK" to save. In addition to the system speed limit, in fact some download software also has speed limit, so it is not necessarily the system speed limit.

Analysis of MyBatis' caching mechanism: The difference and application of first-level cache and second-level cache In the MyBatis framework, caching is a very important feature that can effectively improve the performance of database operations. Among them, first-level cache and second-level cache are two commonly used caching mechanisms in MyBatis. This article will analyze the differences and applications of first-level cache and second-level cache in detail, and provide specific code examples to illustrate. 1. Level 1 Cache Level 1 cache is also called local cache. It is enabled by default and cannot be turned off. The first level cache is SqlSes
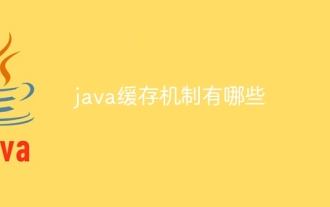
Java cache mechanisms include memory cache, data structure cache, cache framework, distributed cache, cache strategy, cache synchronization, cache invalidation mechanism, compression and encoding, etc. Detailed introduction: 1. Memory cache, Java's memory management mechanism will automatically cache frequently used objects to reduce the cost of memory allocation and garbage collection; 2. Data structure cache, Java's built-in data structures, such as HashMap, LinkedList, HashSet, etc. , with efficient caching mechanisms, these data structures use internal hash tables to store elements and more.
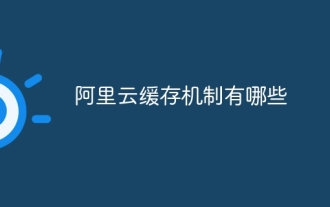
Alibaba Cloud caching mechanisms include Alibaba Cloud Redis, Alibaba Cloud Memcache, distributed cache service DSC, Alibaba Cloud Table Store, CDN, etc. Detailed introduction: 1. Alibaba Cloud Redis: A distributed memory database provided by Alibaba Cloud that supports high-speed reading and writing and data persistence. By storing data in memory, it can provide low-latency data access and high concurrency processing capabilities; 2. Alibaba Cloud Memcache: the cache system provided by Alibaba Cloud, etc.
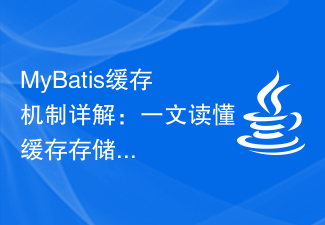
Detailed explanation of MyBatis caching mechanism: One article to understand the principle of cache storage Introduction When using MyBatis for database access, caching is a very important mechanism, which can effectively reduce access to the database and improve system performance. This article will introduce the caching mechanism of MyBatis in detail, including cache classification, storage principles and specific code examples. 1. Cache classification MyBatis cache is mainly divided into two types: first-level cache and second-level cache. The first-level cache is a SqlSession-level cache. When
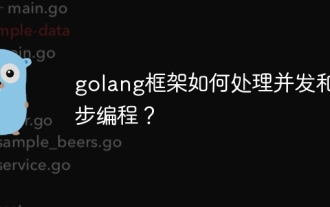
The Go framework uses Go's concurrency and asynchronous features to provide a mechanism for efficiently handling concurrent and asynchronous tasks: 1. Concurrency is achieved through Goroutine, allowing multiple tasks to be executed at the same time; 2. Asynchronous programming is implemented through channels, which can be executed without blocking the main thread. Task; 3. Suitable for practical scenarios, such as concurrent processing of HTTP requests, asynchronous acquisition of database data, etc.
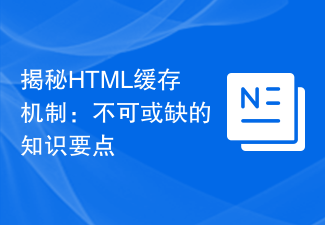
The secret of HTML caching mechanism: essential knowledge points, specific code examples are required In web development, performance has always been an important consideration. The HTML caching mechanism is one of the keys to improving the performance of web pages. This article will reveal the principles and practical skills of the HTML caching mechanism, and provide specific code examples. 1. Principle of HTML caching mechanism During the process of accessing a Web page, the browser requests the server to obtain the HTML page through the HTTP protocol. HTML caching mechanism is to cache HTML pages in the browser
