How to perform data encryption and decryption for Java function development
How to perform data encryption and decryption for Java function development
Data encryption and decryption play an important role in the field of information security. In Java development, there are many ways to implement data encryption and decryption functions. This article will introduce the method of data encryption and decryption using Java programming language and provide code examples.
1. Symmetric encryption algorithm
The symmetric encryption algorithm uses the same key to encrypt and decrypt data. Commonly used symmetric encryption algorithms include DES, 3DES, AES, etc.
Code example:
import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; import java.security.SecureRandom; import java.util.Base64; public class SymmetricEncryptionExample { public static void main(String[] args) throws Exception { String plaintext = "Hello, World!"; String secretKey = "0123456789abcdef"; // Generate Key SecretKey key = new SecretKeySpec(secretKey.getBytes(), "AES"); // Create Cipher Cipher cipher = Cipher.getInstance("AES"); // Encrypt cipher.init(Cipher.ENCRYPT_MODE, key); byte[] encryptedBytes = cipher.doFinal(plaintext.getBytes()); String encryptedText = Base64.getEncoder().encodeToString(encryptedBytes); System.out.println("Encrypted Text: " + encryptedText); // Decrypt cipher.init(Cipher.DECRYPT_MODE, key); byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(encryptedText)); String decryptedText = new String(decryptedBytes); System.out.println("Decrypted Text: " + decryptedText); } }
2. Asymmetric encryption algorithm
The asymmetric encryption algorithm uses a pair of keys, the public key is used for encryption, and the private key is used for decryption. In Java, the commonly used asymmetric encryption algorithm is RSA.
Code example:
import javax.crypto.Cipher; import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.PrivateKey; import java.security.PublicKey; import java.util.Base64; public class AsymmetricEncryptionExample { public static void main(String[] args) throws Exception { String plaintext = "Hello, World!"; // Generate Key Pair KeyPairGenerator keyGenerator = KeyPairGenerator.getInstance("RSA"); keyGenerator.initialize(2048); KeyPair keyPair = keyGenerator.generateKeyPair(); PublicKey publicKey = keyPair.getPublic(); PrivateKey privateKey = keyPair.getPrivate(); // Create Cipher Cipher cipher = Cipher.getInstance("RSA"); // Encrypt cipher.init(Cipher.ENCRYPT_MODE, publicKey); byte[] encryptedBytes = cipher.doFinal(plaintext.getBytes()); String encryptedText = Base64.getEncoder().encodeToString(encryptedBytes); System.out.println("Encrypted Text: " + encryptedText); // Decrypt cipher.init(Cipher.DECRYPT_MODE, privateKey); byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(encryptedText)); String decryptedText = new String(decryptedBytes); System.out.println("Decrypted Text: " + decryptedText); } }
3. Hash algorithm
The hash algorithm can convert data of any length into a fixed-length hash value. Commonly used hash algorithms There are MD5, SHA-1, SHA-256, etc.
Code examples:
import java.security.MessageDigest; public class HashAlgorithmExample { public static void main(String[] args) throws Exception { String plaintext = "Hello, World!"; // Create MessageDigest MessageDigest md = MessageDigest.getInstance("SHA-256"); // Hash byte[] hashedBytes = md.digest(plaintext.getBytes()); StringBuilder stringBuilder = new StringBuilder(); for (byte hashedByte : hashedBytes) { stringBuilder.append(Integer.toHexString(0xFF & hashedByte)); } String hashedText = stringBuilder.toString(); System.out.println("Hashed Text: " + hashedText); } }
Summary:
This article introduces the methods and code examples of using Java to develop and implement data encryption and decryption. In actual development, appropriate encryption algorithms and key lengths are selected according to different needs to ensure data security.
The above is the detailed content of How to perform data encryption and decryption for Java function development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


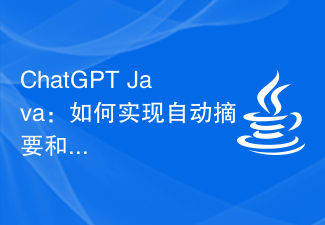
ChatGPTJava: How to implement automatic summarization and extraction of key information from articles, specific code examples are required. Summary and key information extraction are very important tasks in information retrieval and text processing. To implement automatic summarization and extract key information of articles in Java, you can use natural language processing (NLP) libraries and related algorithms. This article will introduce how to use Lucene and StanfordCoreNLP to implement these functions, and give specific code examples. 1. Automatic summarization Automatic summarization is extracted from text
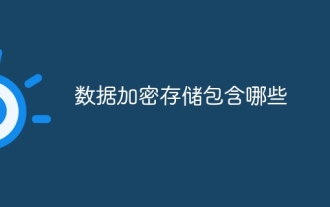
Data encryption storage includes symmetric encryption, asymmetric encryption, hash algorithm, digital signature and other methods. Detailed introduction: 1. Symmetric encryption. During the encryption process, the original data and key are calculated by the encryption algorithm to generate encrypted ciphertext; 2. Asymmetric encryption. During the asymmetric encryption process, the data encrypted with the public key only Can be decrypted with the private key and vice versa; 3. Hash algorithm, which can verify the integrity and consistency of the data during data transmission and storage; 4. Digital signature, etc.
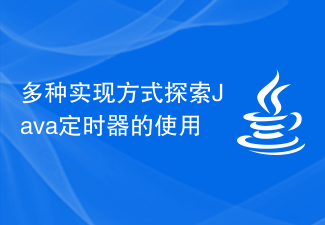
To explore the various implementation methods of Java timers, specific code examples are required. In modern software development, timers are a very common and important function. It can perform a task at a specified time interval or a specific time, and is often used in scenarios such as heartbeat detection, scheduled task scheduling, and data refresh. In Java development, there are many ways to implement timers. This article will explore some of the common ways and provide corresponding code examples. Use the java.util.Timer class that comes with Java
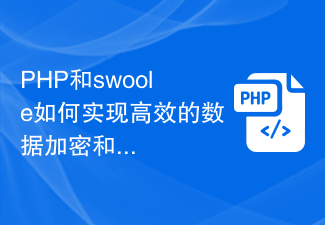
PHP is a very popular programming language that is widely used in the field of web development. Swoole is a high-performance PHP language extension that can provide features such as asynchronous IO and coroutines, which greatly improves PHP's concurrency capabilities. In some scenarios, we need to encrypt and decrypt data, so how to use PHP and swoole to achieve efficient data encryption and decryption? First, let's introduce the choice of encryption algorithm. In practical applications, commonly used encryption algorithms include symmetric encryption algorithms and asymmetric encryption algorithms.
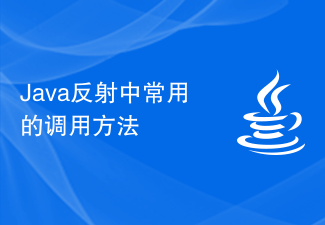
Commonly used calling methods in Java reflection require specific code examples Introduction: Java reflection is a powerful language feature of Java, which allows us to dynamically obtain class information and operate class attributes, methods, constructors, etc. at runtime . In Java, by using reflection, we can achieve many functions, such as dynamically creating objects, calling methods, and modifying private properties. This article will introduce the commonly used calling methods in reflection in Java and provide specific code examples. Get the Class object before using reflection
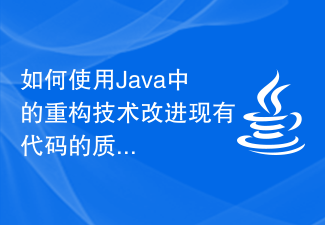
How to use refactoring techniques in Java to improve the quality and design of existing code? Introduction: In software development, code quality and design directly affect the maintainability and scalability of the software. Refactoring technology is an effective means to help improve code quality and design. This article will introduce how to use refactoring technology in Java to improve the quality and design of existing code, and demonstrate the application of refactoring technology through code examples. 1. Understand the concept of refactoring. Refactoring refers to modifying the internal structure of the code without changing the external behavior of the code.
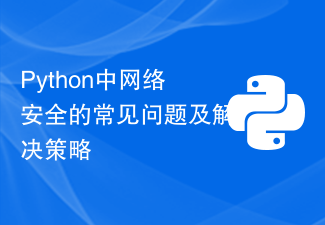
Common problems and solution strategies for network security in Python Network security is one of the important issues that cannot be ignored in today's information age. With the popularity and widespread application of the Python language, network security has also become a challenge that Python developers need to face and solve. This article will introduce common network security issues in Python and provide corresponding solution strategies and code examples. 1. Network security issues SQL injection attack SQL injection attack means that the attacker inserts malicious SQL code into the parameters entered by the user, thereby
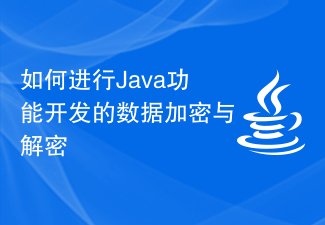
How to perform data encryption and decryption in Java function development Data encryption and decryption play an important role in the field of information security. In Java development, there are many ways to implement data encryption and decryption functions. This article will introduce the method of data encryption and decryption using Java programming language and provide code examples. 1. Symmetric encryption algorithm Symmetric encryption algorithm uses the same key to encrypt and decrypt data. Commonly used symmetric encryption algorithms include DES, 3DES, AES, etc. Code example: importja
