


Workerman Development Pitfall Guide: Experience Summary and Sharing on Solving Common Problems in Network Applications
Workerman Development Pitfall Guide: Summary and Sharing of Experience in Solving Common Problems in Network Applications
Introduction:
In the process of network application development, we often encounter some difficult problems. This article will provide some experience summaries and sharing on solving these problems based on actual experience. We will use Workerman as the development framework and provide relevant code examples.
1. Understanding and Optimizing Event Loop
Workerman is a development framework based on Event Loop. Understanding the principles of Event Loop is very helpful for solving problems. In network applications, we often face high concurrency and large data volumes. In response to this situation, we can optimize through the following points:
- Use multi-process or multi-thread
Workerman supports multi-process or multi-thread mode, which can be set by setting the number of worker processes or threads. Improve processing capabilities. The sample code is as follows:
Worker::$count = 4; // 设置4个worker进程
- Load Balancing
If the application load is too large, you can consider using load balancing to share the pressure. Load balancing can be achieved through tools such as Nginx. The sample configuration is as follows:
upstream backend { server 127.0.0.1:8080; server 127.0.0.1:8081; server 127.0.0.1:8082; server 127.0.0.1:8083; } server { listen 80; server_name example.com; location / { proxy_pass http://backend; } }
2. Stability and performance optimization of TCP connection
- Heartbeat mechanism
In network applications, the stability of TCP connection is very important. important. In order to keep the connection active, we can detect the health of the connection by using a heartbeat mechanism. The sample code is as follows:
use WorkermanConnectionTcpConnection; TcpConnection::$defaultMaxLifetime = 60; // 设置连接最大空闲时间(单位:秒) class MyWorker extends Worker { public function onConnect($connection) { $connection->heartbeat = time(); } public function onMessage($connection, $data) { $connection->heartbeat = time(); // 处理业务逻辑 } public function onCheckHeartbeat($connection) { $maxLifetime = TcpConnection::$defaultMaxLifetime; if (time() - $connection->heartbeat > $maxLifetime) { $connection->close(); } } }
- Packet sticking and unpacking problems
In network communication, due to the unreliability of data transmission, packet sticking and unpacking problems will occur. To solve this problem, we can use fixed-length packets for communication. The sample code is as follows:
use WorkermanConnectionTcpConnection; class MyWorker extends Worker { public function onMessage($connection, $data) { $packLength = 4; // 数据包长度(单位:字节) $recvBuffer = $connection->getRecvBuffer(); while (strlen($recvBuffer) > $packLength) { $packet = substr($recvBuffer, 0, $packLength); // 获取一个完整数据包 $recvBuffer = substr($recvBuffer, $packLength); // 移除已处理的数据包 // 处理数据包 } $connection->setRecvBuffer($recvBuffer); } }
3. The use and optimization of asynchronous non-blocking IO
- Asynchronous task processing
In network applications, some tasks may take time Longer, in order to avoid blocking the execution of other tasks, we can use asynchronous non-blocking IO to handle these tasks. The sample code is as follows:
use WorkermanWorker; class MyWorker extends Worker { public function onMessage($connection, $data) { // 异步任务处理 $this->asyncTask($data, function($result) use ($connection) { // 处理异步任务结果 }); } private function asyncTask($data, $callback) { // 创建异步任务并进行处理 $task = new AsyncTask($data); $task->execute($callback); } }
- Data buffering and batch processing
In network applications, data buffering and batch processing are effective means to improve performance. You can perform batch processing by setting the interval. The sample code is as follows:
use WorkermanWorker; use WorkermanLibTimer; class MyWorker extends Worker { private $buffer = []; public function onMessage($connection, $data) { $this->buffer[] = $data; Timer::add(0.01, function() use ($connection) { $this->handleBuffer($connection); }); } private function handleBuffer($connection) { // 批量处理数据 // ... $this->buffer = []; } }
Summary:
This article mainly introduces common problems and optimization solutions in the process of using Workerman to develop network applications, and provides relevant code examples. I hope these experience summaries and sharing can help readers avoid some pitfalls during the development process. Of course, web application development is an evolving process, and different scenarios and needs may require different solutions. I hope readers can accumulate more experience in practice and continuously optimize and improve their applications.
The above is the detailed content of Workerman Development Pitfall Guide: Experience Summary and Sharing on Solving Common Problems in Network Applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


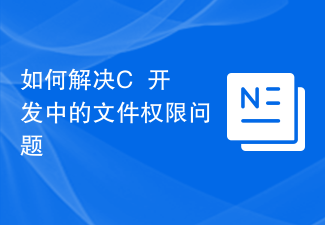
How to solve file permission issues in C++ development During the C++ development process, file permission issues are a common challenge. In many cases, we need to access and operate files with different permissions, such as reading, writing, executing and deleting files. This article will introduce some methods to solve file permission problems in C++ development. 1. Understand file permissions Before solving file permissions problems, we first need to understand the basic concepts of file permissions. File permissions refer to the file's owner, owning group, and other users' access rights to the file. In Li
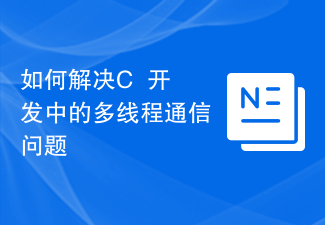
How to solve the multi-threaded communication problem in C++ development. Multi-threaded programming is a common programming method in modern software development. It allows the program to perform multiple tasks at the same time during execution, improving the concurrency and responsiveness of the program. However, multi-threaded programming will also bring some problems, one of the important problems is the communication between multi-threads. In C++ development, multi-threaded communication refers to the transmission and sharing of data or messages between different threads. Correct and efficient multi-thread communication is crucial to ensure program correctness and performance. This article
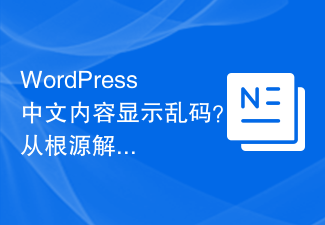
WordPress is a powerful open source content management system that is widely used in website construction and blog publishing. However, in the process of using WordPress, sometimes you encounter the problem of Chinese content displaying garbled characters, which brings troubles to user experience and SEO optimization. Starting from the root cause, this article introduces the possible reasons why WordPress Chinese content displays garbled characters, and provides specific code examples to solve this problem. 1. Cause analysis Database character set setting problem: WordPress uses a database to store the website
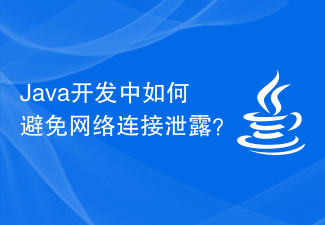
How to solve the problem of network connection leakage in Java development. With the rapid development of information technology, network connection is becoming more and more important in Java development. However, the problem of network connection leakage in Java development has gradually become prominent. Network connection leaks can lead to system performance degradation, resource waste, system crashes, etc. Therefore, solving the problem of network connection leaks has become crucial. Network connection leakage means that the network connection is not closed correctly in Java development, resulting in the failure of connection resources to be released, thus preventing the system from working properly. solution network
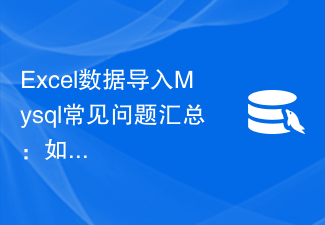
Summary of frequently asked questions about importing Excel data into Mysql: How to solve the problem of field type mismatch? Importing data is a very common operation in database management, and Excel, as a common data processing tool, is usually used for data collection and organization. However, when importing Excel data into a Mysql database, you may encounter field type mismatch problems. This article will discuss this issue and provide some solutions. First, let’s understand the origin of the problem of field type mismatch.
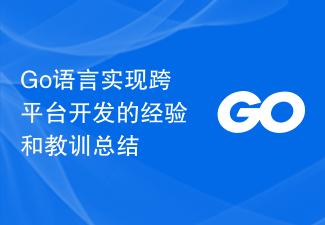
Summary of experience and lessons learned in implementing cross-platform development with Go language Introduction: With the rapid development of the mobile Internet, cross-platform development has become the first choice for many developers. As an open source programming language, Go language is loved by developers for its simplicity, efficiency and cross-platform features. In this article, we will summarize some experiences and lessons learned in the process of using Go language for cross-platform development and illustrate it through code examples. 1. Understand the characteristics and limitations of the target platform. Before starting cross-platform development, it is very important to understand the characteristics and limitations of the target platform. different
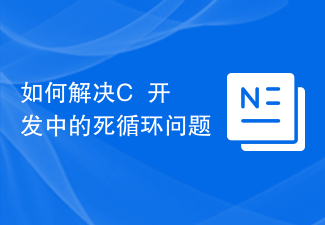
How to solve the infinite loop problem in C++ development. In C++ development, the infinite loop is a very common but very difficult problem. When a program falls into an infinite loop, it will cause the program to fail to execute normally, and may even cause the system to crash. Therefore, solving infinite loop problems is one of the essential skills in C++ development. This article will introduce some common methods to solve the infinite loop problem. Checking Loop Conditions One of the most common causes of endless loops is incorrect loop conditions. When the loop condition is always true, the loop will continue to execute, resulting in an infinite loop.
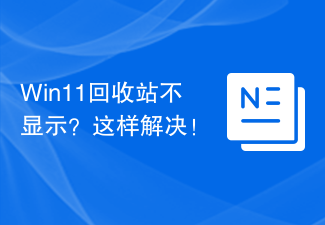
Win11 Recycle Bin not showing? This is the solution! Recently, many Win11 system users have reported a common problem: the recycle bin icon disappears on the desktop and cannot be displayed normally. This not only prevents users from finding ways to recover files after deleting them, but also brings inconvenience to daily use. Well, if you also face this problem, don’t worry. In this article, we will introduce you to several solutions to help you restore the disappeared Recycle Bin icon in Win11 system. Method 1: Confirm that the Recycle Bin is not hidden. First, we need to ensure that the Recycle Bin
