


How to handle PHP data type errors and generate related error prompts
How to handle PHP data type errors and generate related error prompts
In PHP programming, we often encounter problems with data type errors, such as calculating strings as integers, or treating arrays as Used as a string. These errors may cause the program to fail or produce incorrect results. Therefore, we need to detect and handle data type errors in time. This article will introduce how to handle data type errors in PHP and provide relevant code examples.
1. Data type detection function
PHP provides a series of functions to determine the data type of variables. These functions can be used to detect the data type and perform corresponding processing. The following are several commonly used data type detection functions:
- is_int(): Determine whether the variable is an integer type
- is_float(): Determine whether the variable is a floating point type
- is_string(): Determine whether the variable is of string type
- is_array(): Determine whether the variable is of array type
- is_bool(): Determine whether the variable is of Boolean type
Use these functions for data type detection and generate relevant error prompts when errors occur, so that problems can be discovered and repaired in a timely manner.
2. How to generate error prompts
In PHP, we can use two methods to generate error prompts, namely throwing exceptions and using error handling functions.
- Throw exception
PHP's exception mechanism allows us to throw an exception when an error occurs, thus interrupting the execution of the program and generating corresponding error information. When an exception is thrown, we can add customized error information to facilitate problem location. The following is a sample code that uses the exception mechanism to generate an error message:
function divide($num1, $num2) { if (!is_numeric($num1) || !is_numeric($num2)) { throw new Exception('参数必须为数字'); } if ($num2 == 0) { throw new Exception('除数不能为0'); } return $num1 / $num2; } try { echo divide(10, 'a'); } catch (Exception $e) { echo '错误信息:' . $e->getMessage(); }
In the above code, we define a divide()
function to calculate the division of two numbers. Inside the function, we first use the is_numeric()
function to determine whether the incoming parameter is a numeric type, and if not, an exception will be thrown. Additionally, if the divisor is 0, an exception is also thrown. In the try-catch
code block, we can catch and handle exceptions and output custom error messages.
- Error handling function
In addition to using the exception mechanism, PHP also provides a series of error handling functions to generate corresponding error messages when an error occurs. Among them, the most commonly used functions are trigger_error()
and error_log()
. The following is a sample code that uses an error handling function to generate an error message:
function divide($num1, $num2) { if (!is_numeric($num1) || !is_numeric($num2)) { trigger_error('参数必须为数字', E_USER_ERROR); return false; } if ($num2 == 0) { trigger_error('除数不能为0', E_USER_ERROR); return false; } return $num1 / $num2; } divide(10, 'a');
In the above code, we still use the divide()
function to calculate the division of two numbers. Within the function, when the parameter is not a numeric type or the divisor is 0, we use the trigger_error()
function to generate the corresponding error message and specify the error level as E_USER_ERROR
. In this way, when the program execution reaches this point, the execution of the program will be interrupted and the corresponding error message will be displayed.
3. How to handle data type errors
When a data type error occurs, we can handle it accordingly according to the specific situation. The following are several common processing methods:
- Forcing data type: In some cases, we can solve the problem of wrong data type by forcing the data type. For example, use the
intval()
function to convert a string to an integer, or thefloatval()
function to convert a string to a floating point number. - Detect and fix errors: In some cases, we can resolve data type errors by detecting the errors and fixing them accordingly. For example, when using a string as an integer, you can use the
is_numeric()
function to determine whether the string is a numeric type and process it accordingly. - Throw an exception or generate an error prompt: For unrepairable data type errors, we can use the exception mechanism or error handling function to generate corresponding error information so that problems can be discovered and repaired in a timely manner.
To sum up, for data type errors in PHP, we can use the data type detection function to discover and handle such errors. At the same time, by using the exception mechanism or error handling function, we can generate relevant error information to facilitate timely location and repair of problems. When writing PHP programs, we should pay attention to detecting the data types of incoming parameters and processing them accordingly to ensure the correctness and stability of the program.
The above is the detailed content of How to handle PHP data type errors and generate related error prompts. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


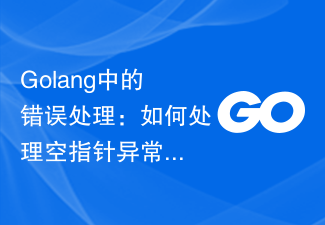
Error handling in Golang: How to handle null pointer exception? When programming in Golang, we often encounter null pointer exceptions. Null pointer exception means that when we try to operate on a null pointer object, it will cause the program to crash or an unpredictable error will occur. In order to avoid the occurrence of this exception, we need to handle null pointer exceptions reasonably. This article will introduce some methods of handling null pointer exceptions and illustrate them with code examples. 1. Use nil to judge. In Golang, nil represents a null pointer.
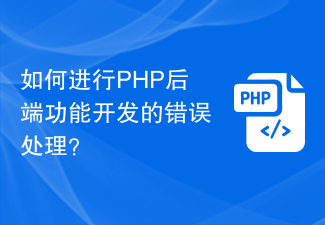
How to handle errors in PHP backend function development? As a PHP backend developer, we often encounter various errors during the development process. Good error handling is an important factor in ensuring system stability and user experience. In this article, I will share some error handling methods and techniques on how to develop PHP back-end functions, and provide corresponding code examples. Setting the error reporting level PHP provides an error reporting level parameter that can be set to define the types of errors to be reported. Use error_repo
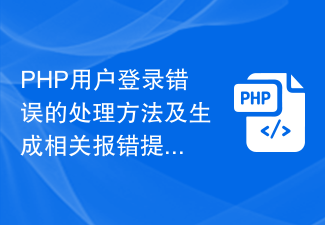
How to handle PHP user login errors and generate related error prompts. In the process of website development, user login is a common functional module. Users may enter incorrect login information for various reasons, or there may be server-side errors that cause login failure. For these error situations, we need to handle them appropriately and provide corresponding error prompts to users to improve user experience. This article will introduce how to handle user login errors in PHP, and demonstrate how to generate relevant error prompts. Error Type Classification User Login Error Can
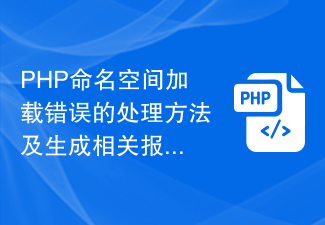
How to handle PHP namespace loading errors and generate related error prompts. In PHP development, namespace is a very important concept. It can help us organize and manage code and avoid naming conflicts. However, when using namespaces, sometimes some loading errors occur. These errors may be caused by incorrect namespace definitions or incorrect paths to loaded files. This article will introduce some common namespace loading errors, give corresponding processing methods, and how to generate relevant error prompts. 1. Namespace determination
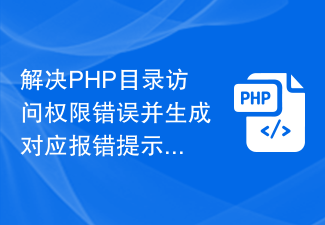
Overview of methods to solve PHP directory access permission errors and generate corresponding error prompts: In PHP development, we often need to read, write, delete and other operations on files or directories on the server. However, when doing these operations, sometimes we encounter a "Permission denied" error, which means that we do not have sufficient permissions to perform this operation. In order to avoid these errors, we can use some methods to solve directory access permission errors and generate corresponding error prompts. method one:

Reasons for PHP startup failure and how to deal with it PHP is a widely used server-side scripting language. Its flexibility and powerful functions make it the first choice for many websites and applications. However, sometimes we encounter the problem of PHP startup failure when deploying or using PHP, which may be due to a variety of reasons. In this article, we will introduce some common reasons for PHP startup failure and how to deal with them, and provide specific code examples to solve these problems. 1. The reason for PHP startup failure is PHP configuration error: PHP
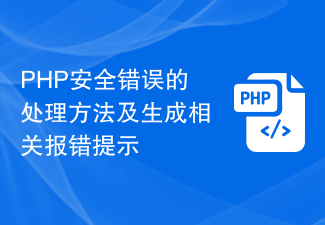
How to handle PHP security errors and generate related error prompts. As a commonly used server-side scripting language for development, PHP is widely used in the development and application of Internet technology. However, due to the flexibility and ease of use of PHP, it also brings some security risks to developers. This article will discuss how to handle PHP security errors and how to generate relevant error prompts. 1. Filter user input data In actual development, user input data is the most likely place to cause security problems, such as SQL injection, cross-site scripting attacks, etc. in order to avoid
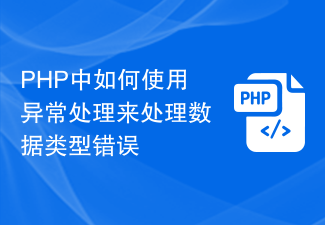
PHP is a commonly used server-side scripting language used to develop dynamic websites and web applications. When performing data processing, data type errors are often encountered. In order to handle these errors, PHP provides an exception handling mechanism. This article will share how to use exception handling to handle data type errors, with code examples. Exception handling is a mechanism for handling errors while a program is running. When an error occurs, an exception can be thrown to notify the code that an abnormal condition has occurred during execution. In PHP, exceptions are thrown via the slang
