PHP form processing: form reset and data clearing
PHP form processing: form reset and data clearing
In web development, forms are a very important part, used to collect data entered by users. After the user submits the form, we usually process the form data and perform some necessary operations. However, in actual development, we often encounter situations where we need to reset the form or clear the form data.
This article will introduce how to use PHP to implement the form reset and data clearing functions, and provide corresponding code examples.
- Form Reset
First, we need to understand the concept of form reset. After the user fills out the form, they may want to refill the form or restore the form to its original state. At this time, we can use the form reset function to achieve this.
In HTML, the form reset function can be implemented through the <input type="reset">
tag. When the user clicks the reset button, all input fields in the form are reset to their default values.
The following is a simple HTML form example:
<form action="submit.php" method="post"> <label for="name">姓名:</label> <input type="text" name="name" id="name" value=""><br> <label for="email">邮箱:</label> <input type="email" name="email" id="email" value=""><br> <input type="submit" value="提交"> <input type="reset" value="重置"> </form>
In the above example, when the user clicks the "Reset" button, the "Name" and "Email" fields in the form will be reset. Set to blank.
In the PHP code, we can detect whether the user clicked the reset button and perform some actions when the user submits the form. The following is an example:
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { // 处理表单数据 // ... if (isset($_POST["reset"])) { // 重置表单 // ... } } ?>
In the above example, we use isset($_POST["reset"])
to determine whether the user clicked the reset button. When the user clicks the reset button, we can perform some custom actions, such as setting the value of the input field to empty.
- Data clearing
Sometimes, after the user submits the form, we may need to clear the form data and reload the page. In this case, we can use PHP's header
function to clear data and redirect the page.
The following is an example that demonstrates how to clear the form data and redirect to another page:
<?php if ($_SERVER["REQUEST_METHOD"] == "POST") { // 处理表单数据 // ... if (isset($_POST["submit"])) { // 清除表单数据 $_POST = array(); // 重定向到另一个页面 header("Location: index.php"); exit; } } ?>
In the above example, the form data is cleared when the user clicks the "Submit" button , and the page will redirect to the index.php
page.
In addition to the methods in the above examples, we can also use JavaScript to clear form data. The following is an example of clearing data using JavaScript:
<form action="submit.php" method="post"> <label for="name">姓名:</label> <input type="text" name="name" id="name" value=""><br> <label for="email">邮箱:</label> <input type="email" name="email" id="email" value=""><br> <input type="submit" value="提交" onclick="clearForm()"> </form> <script> function clearForm() { document.getElementById("name").value = ""; document.getElementById("email").value = ""; } </script>
In the above example, when the user clicks the submit button, the clearForm()
function will be called, which will The field's value is set to empty.
Summary:
In web development, form processing is a basic task. This article introduces how to use PHP to implement form reset and data clearing functions, and provides corresponding code examples. By mastering this knowledge, we can better handle form data and provide a better user experience.
The above is the detailed content of PHP form processing: form reset and data clearing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

PHP form processing: form data sorting and ranking In web development, forms are a common user input method. After we collect form data from users, we usually need to process and analyze the data. This article will introduce how to use PHP to sort and rank form data to better display and analyze user-submitted data. 1. Form data sorting When we collect form data submitted by users, we may find that the order of the data does not necessarily meet our requirements. For those that need to be displayed or divided according to specific rules
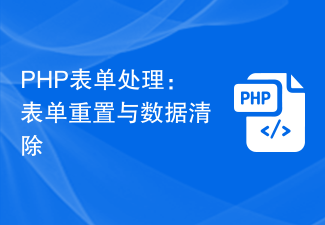
PHP form processing: form reset and data clearing In web development, forms are a very important part and are used to collect data entered by users. After the user submits the form, we usually process the form data and perform some necessary operations. However, in actual development, we often encounter situations where we need to reset the form or clear the form data. This article will introduce how to use PHP to implement form reset and data clearing functions, and provide corresponding code examples. Form reset First, we need to understand the concept of form reset. when user
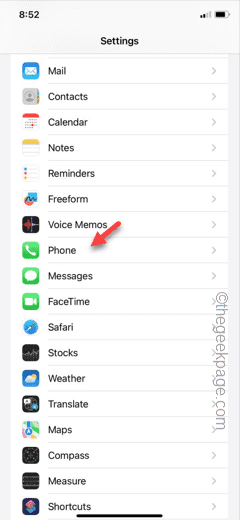
Can't enable Wi-Fi calling on iPhone? Call quality is improved and you can communicate even from remote locations where cellular networks are not as strong. Wi-Fi Calling also improves standard call and video call quality. So, if you can't use Wi-Fi calling on your phone, these solutions might help you fix the problem. Fix 1 – Enable Wi-Fi Calling Manually You must enable the Wi-Fi Calling feature in your iPhone settings. Step 1 – For this, you have to open Settings. Step 2 – Next, just scroll down to find and open the “Phone” settings Step 3 – In the phone settings, scroll down and open the “Wi-Fi Calling” setting. Step 4 – In the Wi-Fi Calling page, change “This iPhone
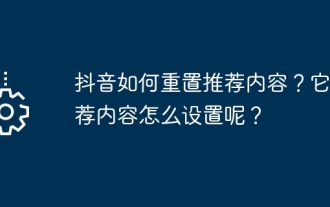
Douyin, the world's most popular short video platform, allows everyone to become a creator and share every moment of life. Sometimes we may get tired of the content recommended by Douyin and hope to reset the recommended content so that the platform can re-evaluate and push content more suitable for us. So, how does Douyin reset recommended content? This article will answer this question in detail. 1. How to reset recommended content on Douyin? Douyin makes personalized recommendations based on users’ viewing history, interactive behavior, interests and preferences and other data. Currently, the Douyin platform does not directly provide the option to reset recommended content. However, there are a few things users can do to try to reset recommended content, such as clearing their viewing history, unfollowing content creators they are not interested in, and diversifying their viewing content to change their recommendations.
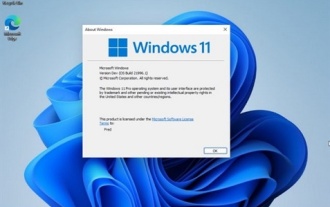
After the launch of win11, many users directly updated it. However, after the update, many users experienced reset failure and did not know how to solve it. So today we bring you the solution to win11 reset failure. Come and see how it works. How to solve win11 reset failure 1. First, click Start in the lower left corner, click Settings, and then select Update and Security. 2. Click the Recovery button on the left and click Start under the Reset this PC option. 3. You can then select an option. 4. Finally, wait for the installation of win11 system to solve the problem. 5. Users can also directly download the win11 system from this site to solve the problem.
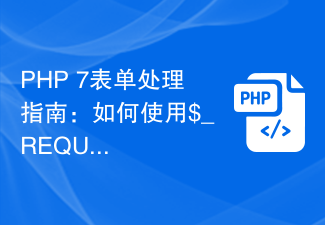
PHP7 Form Processing Guide: How to use the $_REQUEST array to obtain form data Overview: When a user fills out a form on a web page and submits it, the server-side code needs to process the form data. In PHP7, developers can easily obtain form data using the $_REQUEST array. This article will introduce how to correctly use the $_REQUEST array to process form data, and provide some code examples to help readers better understand. 1. Understand the $_REQUEST array: $_REQUES
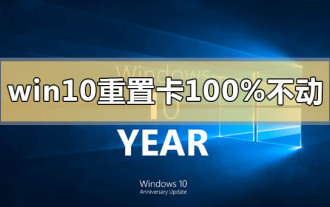
If we want to reset our win10 system to solve some problems and faults, many friends do not know how to solve the problem of being stuck at 100% and unresponsive during the reset process. Then the editor thinks it may be because there are still some files that need to be compiled after the reset of our computer. Generally, it takes 3-4 hours to reset the system, so just wait patiently. Let’s take a look at what the editor said for the detailed steps~ What to do if win10 reset is stuck at 100% and unresponsive. Method 1: 1. Press the power button directly on the Hello interface that has been stuck to shut down 2. Or press Press the restart button to restart, and press ctrl+shift+f33 when Iogo appears on startup. You can enter after skipping the installation settings. There is a high probability that you can enter, but
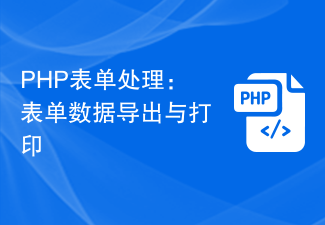
PHP form processing: form data export and printing In website development, forms are an indispensable part. When a form on the website is filled out and submitted by the user, the developer needs to process the form data. This article will introduce how to use PHP to process form data, and demonstrate how to export the data to an Excel file and print it out. 1. Form submission and basic processing First, you need to create an HTML form for users to fill in and submit data. Let's say we have a simple feedback form with name, email, and comments. HTM
