


Code generation for inventory logging function in PHP inventory management system
Code generation for the inventory logging function in the PHP inventory management system
Introduction:
In the inventory management system, inventory logging is very important part of the system that helps us track and manage inventory changes of goods. This article will introduce how to use PHP to write the inventory logging function in the inventory management system, and use code examples to help readers understand and implement this function.
1. Create a database table
First you need to create a database table to store inventory log records. The following is a simple inventory log table structure example:
CREATE TABLE IF NOT EXISTS inventory_logs
(
id
int(11) NOT NULL AUTO_INCREMENT,
product_id
int(11) NOT NULL,
action
varchar(255) NOT NULL,
quantity
int(11) NOT NULL,
created_at
timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (id
)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
This table has The following fields:
- id: The unique identifier of the inventory log record, using an auto-increasing primary key.
- product_id: Product ID, used to associate specific products.
- action: Inventory operation type, such as inbound, outbound, etc.
- quantity: Operation quantity, indicating the quantity of goods for this operation.
- created_at: Record the timestamp created.
2. Write inventory logging code function
Next we will use PHP to write an inventory logging function and operate the database by using PDO. The following is a sample code:
class InventoryLog
{
private $pdo; public function __construct(PDO $pdo) { $this->pdo = $pdo; } public function addLog($product_id, $action, $quantity) { $sql = "INSERT INTO inventory_logs (product_id, action, quantity) VALUES (:product_id, :action, :quantity)"; $stmt = $this->pdo->prepare($sql); $stmt->bindValue(':product_id', $product_id, PDO::PARAM_INT); $stmt->bindValue(':action', $action, PDO::PARAM_STR); $stmt->bindValue(':quantity', $quantity, PDO::PARAM_INT); $stmt->execute(); } public function getLogs($product_id) { $sql = "SELECT * FROM inventory_logs WHERE product_id = :product_id ORDER BY created_at DESC"; $stmt = $this->pdo->prepare($sql); $stmt->bindValue(':product_id', $product_id, PDO::PARAM_INT); $stmt->execute(); return $stmt->fetchAll(PDO::FETCH_ASSOC); }
}
// Usage example
$dbHost = ' localhost';
$dbName = 'inventory';
$dbUser = 'root';
$dbPassword = 'password';
$dsn = "mysql:host=$dbHost; dbname=$dbName;charset=utf8";
$pdo = new PDO($dsn, $dbUser, $dbPassword);
$inventoryLog = new InventoryLog($pdo);
// Add an inventory log record
$inventoryLog->addLog(1, 'Inventory', 10);
// Get the inventory log record of product 1
$logs = $inventoryLog-> ;getLogs(1);
foreach ($logs as $log) {
echo "商品ID: " . $log['product_id'] . " 操作类型: " . $log['action'] . " 操作数量: " . $log['quantity'] . "
";
}
?>
In the above code, we first create A class named InventoryLog is created to handle the inventory logging function. The constructor accepts a PDO object to connect to the database. The addLog method is used to add an inventory log record to the database, and the getLogs method is used to obtain the inventory log record of the specified product.
In the usage example, we first create a PDO object and pass it to the constructor of the InventoryLog class, then use the addLog method to add an inventory log record, and finally use the getLogs method to obtain the inventory log record of the specified product, and Carry out traversal printing.
Summary:
Through simple sample code, we show how to use PHP to write the inventory logging function in the inventory management system. Readers can expand and optimize according to their actual needs. I hope this article can help readers understand and implement the inventory logging function.
The above is the detailed content of Code generation for inventory logging function in PHP inventory management system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
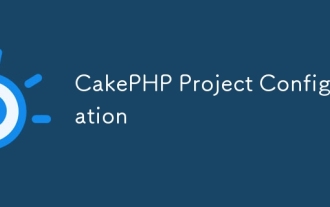
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
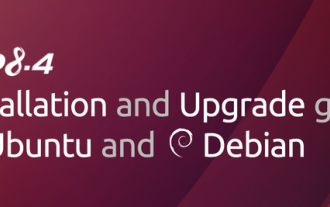
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
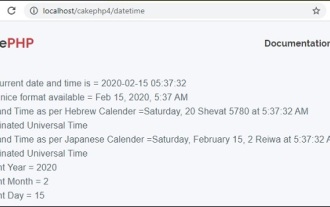
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
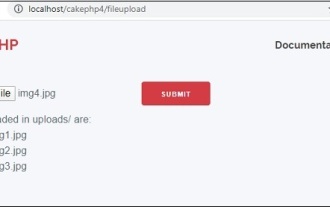
To work on file upload we are going to use the form helper. Here, is an example for file upload.
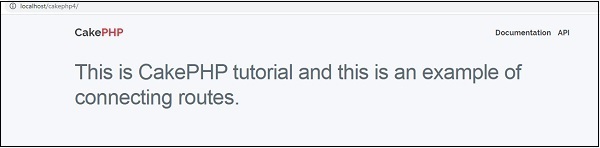
In this chapter, we are going to learn the following topics related to routing ?
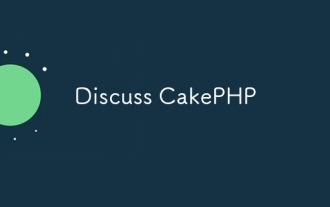
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
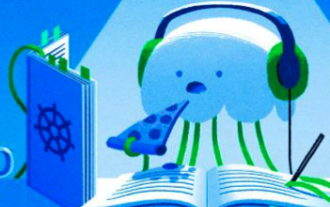
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
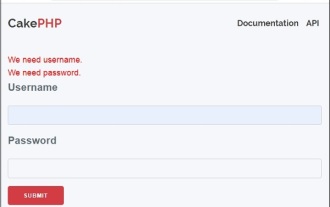
Validator can be created by adding the following two lines in the controller.
