Form data processing and validation using PHP and XML
Use PHP and XML to implement form data processing and validation
Foreword:
In website development, forms are one of the most common and important components. Users enter various data through forms, and we need to process and verify this data to ensure the accuracy and security of the data. In this article, we will use PHP and XML to implement form data processing and validation.
1. Create an HTML form
First, we need to create an HTML form to collect user input data. Here is a simple form example:
<form method="POST" action="process.php"> <label for="name">姓名:</label> <input type="text" name="name" id="name" required> <br> <label for="email">邮箱:</label> <input type="email" name="email" id="email" required> <br> <label for="phone">电话:</label> <input type="text" name="phone" id="phone" required> <br> <input type="submit" value="提交"> </form>
In the above example, we created a form that contains name, email, and phone number. The submission target of the form is process.php
, that is, the form data will be sent to process.php
for processing.
2. Create an XML configuration file
Next, we need to create an XML configuration file to define the validation rules for the form fields. The following is an example configuration file:
<?xml version="1.0" encoding="UTF-8"?> <fields> <field name="name"> <required>true</required> <min_length>2</min_length> <max_length>50</max_length> </field> <field name="email"> <required>true</required> <pattern>^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,4}$</pattern> </field> <field name="phone"> <required>true</required> <pattern>^d{11}$</pattern> </field> </fields>
In the above example, we define validation rules for three fields: the name field is required and the length is between 2 and 50 characters, and the email field is required And it meets the regular expression format. The phone field is required and must be 11 digits.
3. Create a PHP processing script
Now, we need to create a PHP script to process form data and verify results. The following is an example of processing a script:
<?php // 读取XML配置文件 $xml = simplexml_load_file("config.xml"); // 处理表单数据 if ($_SERVER["REQUEST_METHOD"] == "POST") { $errors = []; foreach ($_POST as $key => $value) { // 根据字段名获取对应的验证规则 $field = $xml->xpath("//field[@name='$key']")[0]; // 验证是否必填 if ((string)$field->required == "true" && empty($value)) { $errors[$key] = "必填字段"; } // 验证长度 $length = strlen($value); if ((string)$field->min_length && $length < (int)$field->min_length) { $errors[$key] = "长度太短"; } if ((string)$field->max_length && $length > (int)$field->max_length) { $errors[$key] = "长度太长"; } // 验证正则表达式 if ((string)$field->pattern && !preg_match("/" . (string)$field->pattern . "/i", $value)) { $errors[$key] = "格式不正确"; } } // 输出错误信息或成功提示 if (count($errors) > 0) { echo "表单验证失败:"; foreach ($errors as $key => $error) { echo $key . " " . $error . "<br>"; } } else { echo "表单验证成功!"; } } ?>
In the above example, we first read the XML configuration file through the simplexml_load_file
function and use XPath expressions to find the corresponding field validation rules . Then, use foreach
to loop through the received POST data and validate it based on the validation rules. Finally, an error message or success prompt is output based on the verification results.
4. Test code
Now, we can save the above code as a process.php
script, and put the HTML form and XML configuration file in the same directory as the script file . Then, access the form page in your browser, enter the data and submit. Based on the validity of the data, the system will output corresponding prompt information.
Summary:
Using PHP and XML to process and validate form data allows us to more easily define and modify validation rules, while improving the maintainability and reusability of the code. Through the above steps, we can implement a simple but powerful form data processing and validation system. Hope this article is helpful to you!
The above is the detailed content of Form data processing and validation using PHP and XML. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


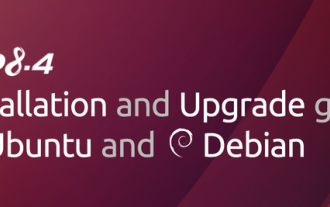
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
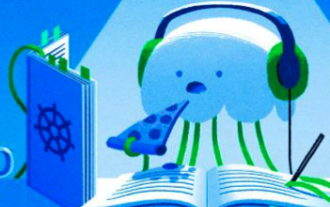
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
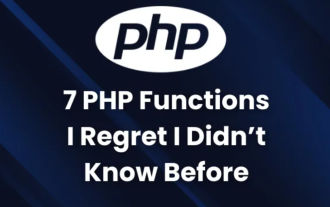
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
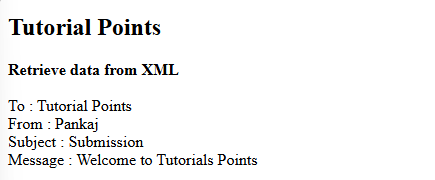
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
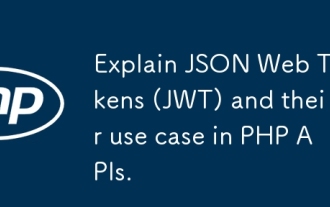
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
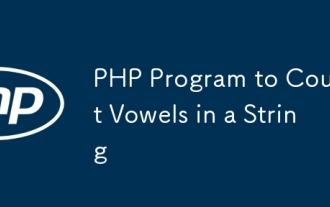
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
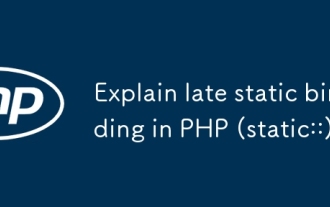
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
