


How to perform code refactoring in PHP backend function development?
How to refactor code in PHP back-end function development?
With the continuous development of software development, code refactoring plays a very important role in the entire development process. Code refactoring can not only improve the readability and maintainability of the code, but also increase the reusability and performance of the code. In the development of PHP back-end functions, code refactoring is also an important part. This article will introduce how to refactor code in PHP back-end function development and give some practical code examples.
- Understand the purpose and principles of code refactoring
The purpose of code refactoring is to improve the internal structure of the software and make it easier to understand and modify. When refactoring code, we need to follow the following principles:
- Keep functionality unchanged: Code refactoring should not change the functionality of the code, but only improve its structure and performance.
- Proceed step by step: Code refactoring should be a gradual process, with only a small part modified at a time.
- Be prepared: Before refactoring the code, make sure there are complete test cases to ensure that the code still runs correctly after refactoring.
- Extract duplicate code as functions or classes
Duplicate code is one of the first goals of code refactoring. We can extract recurring code into a function or class to improve code reusability and maintainability. The following is a sample code as an example:
function calculateArea($radius) { $pi = 3.14; $area = $pi * pow($radius, 2); return $area; } $area1 = calculateArea(5); $area2 = calculateArea(7);
In the above code, the code for calculating the area of a circle is reused twice. We can extract it into a function to reduce the redundancy of the code:
function calculateArea($radius) { $pi = 3.14; $area = $pi * pow($radius, 2); return $area; } $area1 = calculateArea(5); $area2 = calculateArea(7);
- Use design patterns to improve the scalability of the code
Design patterns are a set of reusable solutions summarized through practice solutions to common design problems in software development. In PHP backend development, we can use some common design patterns to improve the scalability of the code. The following takes the singleton mode as an example:
class Database { private static $instance; private function __construct() { // 连接数据库 } public static function getInstance() { if (self::$instance === null) { self::$instance = new self(); } return self::$instance; } } $db1 = Database::getInstance(); $db2 = Database::getInstance();
In the above code, using the singleton mode can ensure that only one database instance is created to reduce the overhead of database connection.
- Reduce the complexity of functions and classes
The complexity of functions and classes is one of the important factors affecting the readability and maintainability of the code. When refactoring your code, you can do this by splitting complex functions and classes into smaller, more concise parts. The following is a sample code as an example:
class User { private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function getName() { return $this->name; } public function getAge() { return $this->age; } public function isAdult() { if ($this->age >= 18) { return true; } else { return false; } } }
In the above code, the logic of the isAdult() function can be more concise and clear. We can directly return a Boolean value by judging age to reduce the complexity of the function:
class User { // 属性和构造函数省略 public function isAdult() { return $this->age >= 18; } }
Through the above code reconstruction, we can find that the readability and maintainability of the code are greatly improved.
Summary: Code refactoring in PHP back-end function development is an important means to improve code quality and maintainability. We need to understand the purpose and principles of code refactoring, and use methods such as extracting duplicate code as functions or classes, using design patterns, and reducing the complexity of functions and classes to refactor code. Through reasonable code refactoring, we can make the code clearer and concise and improve the efficiency of later maintenance.
The above is the detailed content of How to perform code refactoring in PHP backend function development?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


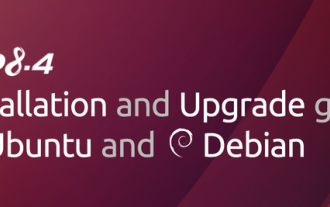
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
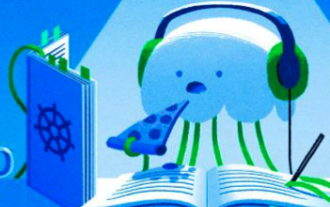
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
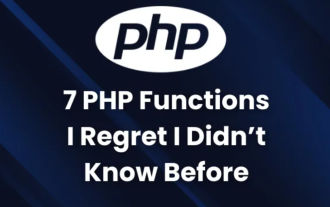
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
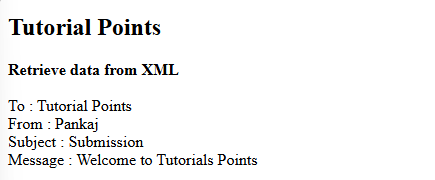
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
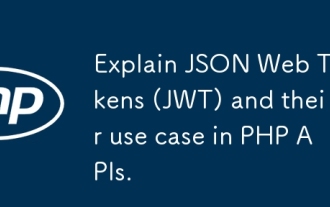
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
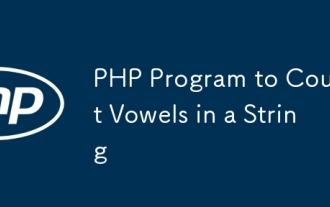
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
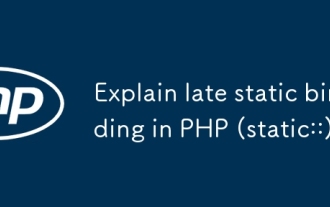
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
