Java development form data history record and version control function
Java development form data history record and version control function
Introduction:
With the rapid development of the Internet, Java, as a programming language widely used in Web development, plays an important role . In web development, form data carries important information for users to interact with applications. In order to ensure the integrity and traceability of data, developers often need to implement the history recording and version control functions of form data.
1. History Recording Function
The history recording function of form data can record every data modification and its changes, making it convenient for developers to view and restore data.
Implementation method:
-
Database table design
First, you need to create a history table for the form data. The table needs to contain the following fields:- id: unique identifier
- form_id: id of the form to which it belongs
- data: JSON format of form data
- create_time: Creation time
-
Database trigger
Create a trigger on the main table of form data to automatically add data when the data is inserted or updated. Add to history table. The logic of the trigger is as follows:CREATE TRIGGER history_trigger AFTER INSERT OR UPDATE ON form_data FOR EACH ROW BEGIN INSERT INTO history_data (form_id, data, create_time) VALUES (NEW.form_id, NEW.data, NOW()); END;
Copy after login
Sample code:
Please refer to the following sample code to demonstrate how to create the history recording function of form data.
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.SQLException; public class FormDataHistory { // 数据库连接信息 private static final String URL = "jdbc:mysql://localhost:3306/mydb"; private static final String USER = "root"; private static final String PASSWORD = "123456"; // 插入数据的SQL语句 private static final String INSERT_SQL = "INSERT INTO form_data (form_id, data) VALUES (?, ?)"; public static void main(String[] args) { try (Connection conn = DriverManager.getConnection(URL, USER, PASSWORD); PreparedStatement stmt = conn.prepareStatement(INSERT_SQL)) { // 假设表单id为1,数据为JSON字符串 int formId = 1; String data = "{"name": "张三", "age": 20}"; stmt.setInt(1, formId); stmt.setString(1, data); int rows = stmt.executeUpdate(); if (rows > 0) { System.out.println("数据插入成功"); } else { System.out.println("数据插入失败"); } } catch (SQLException e) { e.printStackTrace(); } } }
The above sample code demonstrates how to insert form data into the database, and the trigger will automatically add the data to the history table. Developers can customize query historical data and perform analysis and operations as needed.
2. Version control function
The version control function can help developers back up and roll back form data to ensure the consistency and correctness of the data.
Implementation method:
Version field design
First, add a version field to the main table of the form data. When the data is updated, this field will automatically increase by 1, indicating the version number of the current data.Sample code:
ALTER TABLE form_data ADD COLUMN version INT NOT NULL DEFAULT 1;
Copy after loginData backup
Create a trigger on the main table of the form data to automatically save the current version of the data when the data is updated Back up to history table.Sample code:
CREATE TRIGGER backup_trigger AFTER UPDATE ON form_data FOR EACH ROW BEGIN IF NEW.version > OLD.version THEN INSERT INTO history_data (form_id, data, create_time) VALUES (NEW.form_id, NEW.data, NOW()); END IF; END;
Copy after loginData rollback
Developers can select a specific version of data by querying the history table and restore it to in the main table. The sample code is as follows:private static final String SELECT_SQL = "SELECT data FROM history_data WHERE form_id = ? AND version = ?"; private static final String UPDATE_SQL = "UPDATE form_data SET data = ? WHERE form_id = ?"; try (Connection conn = DriverManager.getConnection(URL, USER, PASSWORD); PreparedStatement selectStmt = conn.prepareStatement(SELECT_SQL); PreparedStatement updateStmt = conn.prepareStatement(UPDATE_SQL)) { int formId = 1; int version = 2; selectStmt.setInt(1, formId); selectStmt.setInt(2, version); ResultSet rs = selectStmt.executeQuery(); if (rs.next()) { String data = rs.getString("data"); updateStmt.setString(1, data); updateStmt.setInt(2, formId); int rows = updateStmt.executeUpdate(); if (rows > 0) { System.out.println("数据回滚成功"); } else { System.out.println("数据回滚失败"); } } else { System.out.println("找不到指定版本的数据"); } } catch (SQLException e) { e.printStackTrace(); }
Copy after login
The above sample code demonstrates how to restore a specific version of data in the history table to the main table. Developers can customize query historical data and perform data rollback operations as needed.
Conclusion:
By implementing the historical recording and version control functions of form data, developers can better track and manage data changes and ensure data integrity and traceability. This is crucial for data management and maintenance of web applications, and provides users with better user experience and service quality.
The above is the detailed content of Java development form data history record and version control function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


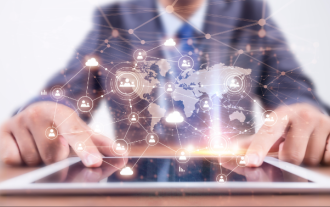
This AI-assisted programming tool has unearthed a large number of useful AI-assisted programming tools in this stage of rapid AI development. AI-assisted programming tools can improve development efficiency, improve code quality, and reduce bug rates. They are important assistants in the modern software development process. Today Dayao will share with you 4 AI-assisted programming tools (and all support C# language). I hope it will be helpful to everyone. https://github.com/YSGStudyHards/DotNetGuide1.GitHubCopilotGitHubCopilot is an AI coding assistant that helps you write code faster and with less effort, so you can focus more on problem solving and collaboration. Git
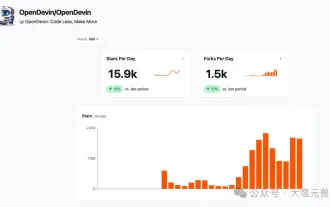
On March 3, 2022, less than a month after the birth of the world's first AI programmer Devin, the NLP team of Princeton University developed an open source AI programmer SWE-agent. It leverages the GPT-4 model to automatically resolve issues in GitHub repositories. SWE-agent's performance on the SWE-bench test set is similar to Devin, taking an average of 93 seconds and solving 12.29% of the problems. By interacting with a dedicated terminal, SWE-agent can open and search file contents, use automatic syntax checking, edit specific lines, and write and execute tests. (Note: The above content is a slight adjustment of the original content, but the key information in the original text is retained and does not exceed the specified word limit.) SWE-A
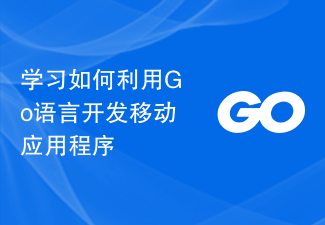
Go language development mobile application tutorial As the mobile application market continues to boom, more and more developers are beginning to explore how to use Go language to develop mobile applications. As a simple and efficient programming language, Go language has also shown strong potential in mobile application development. This article will introduce in detail how to use Go language to develop mobile applications, and attach specific code examples to help readers get started quickly and start developing their own mobile applications. 1. Preparation Before starting, we need to prepare the development environment and tools. head
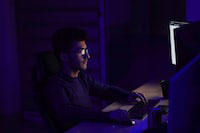
Introduction to SVN SVN (Subversion) is a centralized version control system used to manage and maintain code bases. It allows multiple developers to collaborate on code development simultaneously and provides a complete record of historical modifications to the code. By using SVN, developers can: Ensure code stability and avoid code loss and damage. Track code modification history and easily roll back to previous versions. Collaborative development, multiple developers modify the code at the same time without conflict. Basic SVN Operations To use SVN, you need to install an SVN client, such as TortoiseSVN or SublimeMerge. Then you can follow these steps to perform basic operations: 1. Create the code base svnmkdirHttp://exampl
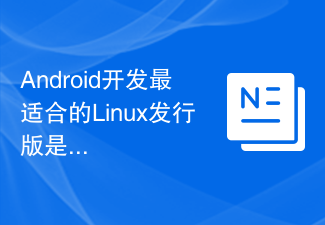
Android development is a busy and exciting job, and choosing a suitable Linux distribution for development is particularly important. Among the many Linux distributions, which one is most suitable for Android development? This article will explore this issue from several aspects and give specific code examples. First, let’s take a look at several currently popular Linux distributions: Ubuntu, Fedora, Debian, CentOS, etc. They all have their own advantages and characteristics.
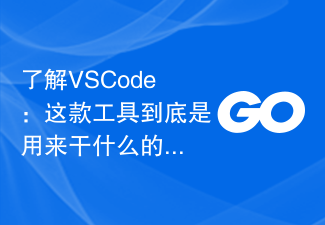
"Understanding VSCode: What is this tool used for?" 》As a programmer, whether you are a beginner or an experienced developer, you cannot do without the use of code editing tools. Among many editing tools, Visual Studio Code (VSCode for short) is very popular among developers as an open source, lightweight, and powerful code editor. So, what exactly is VSCode used for? This article will delve into the functions and uses of VSCode and provide specific code examples to help readers
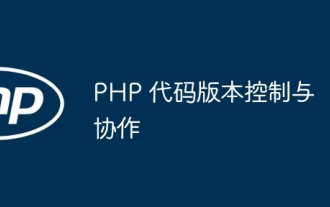
PHP code version control: There are two version control systems (VCS) commonly used in PHP development: Git: distributed VCS, where developers store copies of the code base locally to facilitate collaboration and offline work. Subversion: Centralized VCS, a unique copy of the code base is stored on a central server, providing more control. VCS helps teams track changes, collaborate and roll back to earlier versions.
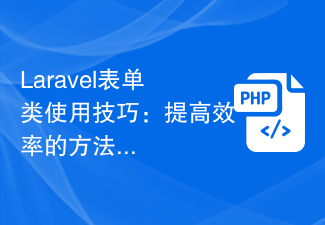
Forms are an integral part of writing a website or application. Laravel, as a popular PHP framework, provides rich and powerful form classes, making form processing easier and more efficient. This article will introduce some tips on using Laravel form classes to help you improve development efficiency. The following explains in detail through specific code examples. Creating a form To create a form in Laravel, you first need to write the corresponding HTML form in the view. When working with forms, you can use Laravel
