How to write testability code in Golang project
How to write testable code in Golang projects
Introduction:
Writing testable code is a key part of developing high-quality software. In Golang projects, the maintainability and stability of the code can be effectively improved through good code structure and writing testable code. This article will introduce some best practices for writing testable code in Golang projects and provide some examples to illustrate.
1. Using interfaces
In Golang, interface is a declaration method used to define the contract of a function. By using interfaces we can provide convenient testing and simulation points. For example, suppose we have a data storage interface as shown below:
type Storage interface { Save(data string) error Load() (string, error) }
In the code that uses this interface, we can unit test it by implementing a storage simulator. This way we can easily simulate saving and loading operations of data without actually accessing the real storage.
2. Dependency Injection
Dependency injection is a design pattern used to decouple code and its dependencies. By externalizing dependencies and passing them to the code, we can easily replace dependencies for testing. In Golang, we can use parameter injection to implement dependency injection.
type MyService struct { storage Storage } func NewMyService(storage Storage) *MyService { return &MyService{storage: storage} } func (s *MyService) SaveData(data string) error { return s.storage.Save(data) }
In the above example, we implemented dependency injection by injecting the storage instance into the MyService structure. This way, when writing test code, we can easily pass a mocked storage instance to test.
3. Use testing tools
Golang provides a wealth of built-in testing tools, such as go test and testing packages. These tools help us write and execute test cases and generate test reports.
When writing test cases, we can use various methods provided by the testing package to assert and verify the output of the code. For example, we can use the methods of the testing.T structure to determine whether some conditions are true, or use the methods provided by the testing package to compare whether the actual output and the expected output are the same.
import ( "testing" ) func TestSaveData(t *testing.T) { storage := &MockStorage{} service := NewMyService(storage) err := service.SaveData("test data") if err != nil { t.Errorf("expected nil, got %v", err) } if storage.Data != "test data" { t.Errorf("expected %s, got %s", "test data", storage.Data) } } type MockStorage struct { Data string } func (s *MockStorage) Save(data string) error { s.Data = data return nil }
In the above example, we used the testing.T method to assert whether the stored data is the same as expected. By using a mock storage instance, we can easily build a fake storage for use in testing.
Conclusion:
Writing testable code is one of the important means to ensure software quality. By using interfaces, dependency injection and testing tools, we can write testable code in Golang projects. These techniques improve code maintainability and stability and provide us with a trustworthy test suite.
(Note: The code example is only for illustration and does not fully realize the function)
The above is the detailed content of How to write testability code in Golang project. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


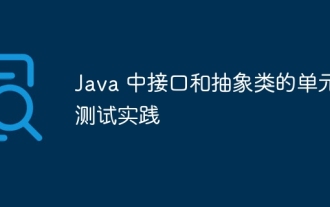
Steps for unit testing interfaces and abstract classes in Java: Create a test class for the interface. Create a mock class to implement the interface methods. Use the Mockito library to mock interface methods and write test methods. Abstract class creates a test class. Create a subclass of an abstract class. Write test methods to test the correctness of abstract classes.

PHP unit testing tool analysis: PHPUnit: suitable for large projects, provides comprehensive functionality and is easy to install, but may be verbose and slow. PHPUnitWrapper: suitable for small projects, easy to use, optimized for Lumen/Laravel, but has limited functionality, does not provide code coverage analysis, and has limited community support.
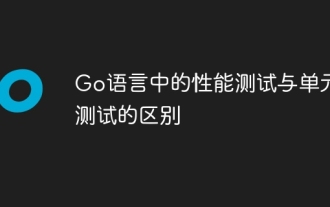
Performance tests evaluate an application's performance under different loads, while unit tests verify the correctness of a single unit of code. Performance testing focuses on measuring response time and throughput, while unit testing focuses on function output and code coverage. Performance tests simulate real-world environments with high load and concurrency, while unit tests run under low load and serial conditions. The goal of performance testing is to identify performance bottlenecks and optimize the application, while the goal of unit testing is to ensure code correctness and robustness.

Unit testing and integration testing are two different types of Go function testing, used to verify the interaction and integration of a single function or multiple functions respectively. Unit tests only test the basic functionality of a specific function, while integration tests test the interaction between multiple functions and integration with other parts of the application.
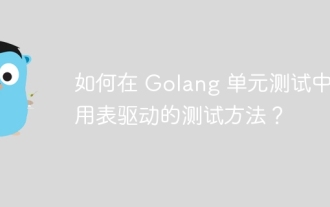
Table-driven testing simplifies test case writing in Go unit testing by defining inputs and expected outputs through tables. The syntax includes: 1. Define a slice containing the test case structure; 2. Loop through the slice and compare the results with the expected output. In the actual case, a table-driven test was performed on the function of converting string to uppercase, and gotest was used to run the test and the passing result was printed.
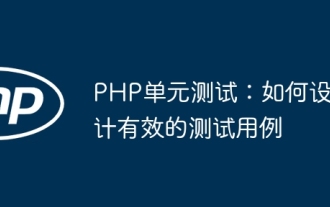
It is crucial to design effective unit test cases, adhering to the following principles: atomic, concise, repeatable and unambiguous. The steps include: determining the code to be tested, identifying test scenarios, creating assertions, and writing test methods. The practical case demonstrates the creation of test cases for the max() function, emphasizing the importance of specific test scenarios and assertions. By following these principles and steps, you can improve code quality and stability.
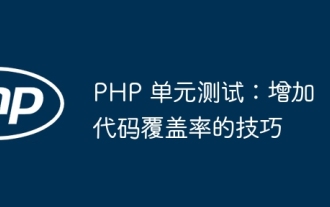
How to improve code coverage in PHP unit testing: Use PHPUnit's --coverage-html option to generate a coverage report. Use the setAccessible method to override private methods and properties. Use assertions to override Boolean conditions. Gain additional code coverage insights with code review tools.
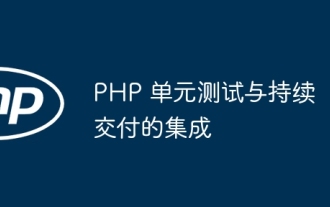
Summary: By integrating the PHPUnit unit testing framework and CI/CD pipeline, you can improve PHP code quality and accelerate software delivery. PHPUnit allows the creation of test cases to verify component functionality, and CI/CD tools such as GitLabCI and GitHubActions can automatically run these tests. Example: Validate the authentication controller with test cases to ensure the login functionality works as expected.
