


Error handling in Golang: Handling errors generated by database operations
Error handling in Golang: Handling errors generated by database operations
In Golang, error handling is an essential part, especially when processing database operations hour. This article will introduce how to effectively handle errors caused by database operations in Golang, and how to use the error handling mechanism to enhance the readability and stability of the code.
Golang’s error handling mechanism returns an error type value to represent errors that may occur during function execution. In database operations, errors are usually divided into two categories: one is errors caused by network connection problems, such as database connection failure; the other is errors caused by operation execution failure, such as inserting duplicate data. For these two types of errors, we need to have different handling methods.
First, we need to establish a database connection. Before performing database operations, we need to ensure that the connection to the database server is successfully established, otherwise subsequent operations will not be possible. In Golang, we can use the database/sql package to perform database operations. The following is a sample code using the mysql driver:
import ( "database/sql" "fmt" "log" _ "github.com/go-sql-driver/mysql" ) func ConnectDB() (*sql.DB, error) { db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/database_name") if err != nil { return nil, err } err = db.Ping() if err != nil { return nil, err } return db, nil }
In the above code, we use the sql.Open function to establish a connection with the mysql database, and use the Ping function to test the availability of the connection. If the connection fails, we will return an error.
Next, we need to deal with possible errors in database operations. In Golang, we usually use if statements to determine whether there is an error and handle the error accordingly. The following is a sample code that demonstrates how to perform a database insertion operation and handle possible errors:
func InsertData(db *sql.DB, data string) error { stmt, err := db.Prepare("INSERT INTO table_name (column_name) VALUES (?)") if err != nil { return err } _, err = stmt.Exec(data) if err != nil { if mysqlErr, ok := err.(*mysql.MySQLError); ok && mysqlErr.Number == 1062 { return fmt.Errorf("数据已存在") } return err } return nil }
In the above code, we prepare a SQL statement template through the Prepare function and pass data as a parameter to Exec The function performs the insertion operation. If the insertion operation is successful, the function will return nil; otherwise, we first determine whether the error is of the mysql.MySQLError type through type assertion, and further determine whether the error code is 1062, that is, the data already exists. If so, we return a custom error; otherwise, the original error is returned.
Finally, we need to handle errors where the database operation is called. The following is a sample code that shows how to handle errors returned by database operations:
func main() { db, err := ConnectDB() if err != nil { log.Fatal(err) } data := "example" err := InsertData(db, data) if err != nil { log.Println(err) } }
In the above code, we first try to connect to the database. If the connection fails, we use the log.Fatal function to print the error message and exit. program. Next, we defined a data variable for insertion operations. Finally, we call the InsertData function to perform the insertion operation and use the log.Println function to print the error message.
Through the above example code, we can see that in Golang, by properly using the error handling mechanism, we can better handle abnormal situations that may occur in database operations and enhance the stability and stability of the code. readability. At the same time, we can also handle errors differently according to specific business needs to achieve better user experience and functional effects.
To summarize, error handling in Golang is crucial for handling errors generated during database operations. By rationally utilizing the error handling mechanism, we can better respond to various possible errors and handle different errors differently. This not only enhances the stability and readability of the code, but also provides us with a better user experience and functionality.
The above is the detailed content of Error handling in Golang: Handling errors generated by database operations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Error handling in Golang: Handling errors generated by database operations In Golang, error handling is an essential part, especially when dealing with database operations. This article will introduce how to effectively handle errors caused by database operations in Golang, and how to use the error handling mechanism to enhance the readability and stability of the code. Golang's error handling mechanism returns an error type value to indicate errors that may occur during function execution. In database operations, errors usually
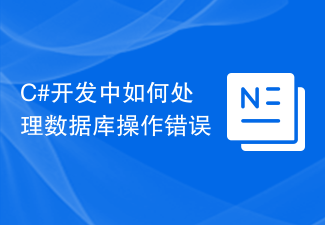
How to handle database operation errors in C# development In C# development, database operation is a common task. However, when performing database operations, you may encounter various errors, such as connection failure, query failure, update failure, etc. In order to ensure the robustness and stability of the program, we need to take corresponding strategies and measures when dealing with database operation errors. Here are some suggestions and specific code examples for handling errors in database operations: Exception Handling In C#, you can use the exception handling mechanism to catch and handle errors in database operations. exist
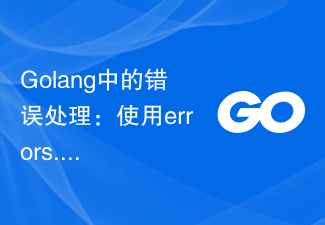
Error handling in Golang: Create custom errors using errors.New function Error handling is an integral part of software development. In Golang, error handling is handled by returning error information as a return value. Golang itself provides some error types, such as the error interface and functions in the errors package. This article will focus on how to use the errors.New function to create custom errors. In Golang, the errors.New function is used to create a
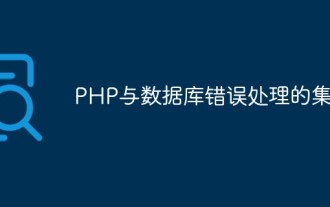
Database error handling is a very important part of the development process. Because we cannot guarantee that every data is correct and complete, some unexpected errors may occur during the process of reading and writing the database. Therefore, developers must pay attention to handling these errors reasonably in the program to ensure the robustness of the program. PHP is a very commonly used back-end programming language. One of its advantages is that it is very convenient to integrate with the database. For database error handling, some built-in functions of PHP, such as PDO, mysqli, mysq
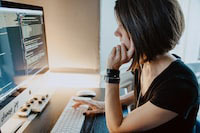
PDO (PHPDataObject) is a powerful tool for handling database interaction in PHP. To ensure code robustness and handle errors gracefully, exception handling is crucial in PDO. This article will delve into PHPPDO's exception handling mechanism and provide code examples to help you effectively handle database errors and improve code quality. Exception Handling OverviewException handling is a programming technique that allows code to respond in a controlled manner when it encounters errors or unexpected conditions. In PHP, exceptions are represented by the Exception class and its subclasses such as PDOException. Using exception handling, you can catch and handle errors, avoid code interruptions, and provide meaningful error messages. PDO exception handling PDO provides
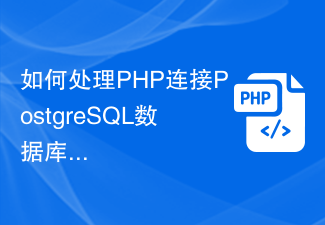
How to deal with error prompts when PHP connects to PostgreSQL database. In PHP development, connecting to the database is a common operation. When connecting to PostgreSQL database, sometimes you will encounter some error prompts. This article will introduce how to handle error prompts when PHP connects to PostgreSQL database, and provide specific code examples to help developers solve the problem. When using PHP to connect to a PostgreSQL database, PDO (PHPDataObjects) is usually used
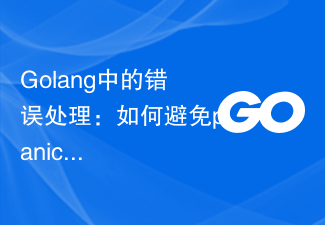
Error handling in Golang: How to avoid panic? In Golang, error handling is a very important task. Handling errors correctly not only improves the robustness of your program, it also makes your code more readable and maintainable. In error handling, a very common problem is the occurrence of panic. This article will introduce the concept of panic and discuss how to avoid panic and how to handle errors correctly. What is panic? In Golang, panic is an exception
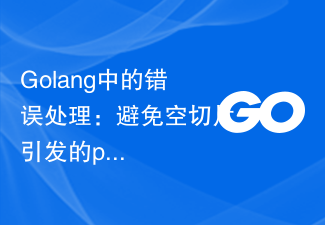
Error handling in Golang: Avoid panic caused by empty slices Introduction: Error handling is a very important part when writing programs in Golang. Good error handling practices can help us avoid potential problems in our programs and improve program stability and reliability. This article will focus on a common error handling scenario, namely panic caused by empty slices, and provide corresponding code examples. Importance of error handling: In Golang, error handling is by returning an error object in response to possible
